版本一:增加、删除
<script setup>
import { ref } from 'vue'
// 给每个 todo 对象一个唯一的 id
let id = 0
const newTodo = ref('')
const todos = ref([
{ id: id++, text: 'Learn HTML' },
{ id: id++, text: 'Learn JavaScript' },
{ id: id++, text: 'Learn Vue' }
])
function addTodo() {
todos.value.push({ id: id++, text: newTodo.value })
newTodo.value = ''
}
function removeTodo(todo) {
// filter方法是根据条件过滤返回一个新的,t表示todos里的每一项,todo表示选中的这项,两者不同就过滤出来
todos.value = todos.value.filter((t) => t !== todo)
}
</script>
<template>
//监听表单的提交事件 (@submit)在提交事件被触发时,阻止表单的默认提交行为 (prevent)
<form @submit.prevent="addTodo">
<input v-model="newTodo" required placeholder="new todo">
<button>Add Todo</button>
</form>
<ul>
<li v-for="todo in todos" :key="todo.id">
{{ todo.text }}
<button @click="removeTodo(todo)">X</button>
</li>
</ul>
</template>
版本二:筛选出未完成的:computed
<script setup>
import { ref, computed } from 'vue'
let id = 0
const newTodo = ref('')
const hideCompleted = ref(false)
const todos = ref([
{ id: id++, text: 'Learn HTML', done: true },
{ id: id++, text: 'Learn JavaScript', done: true },
{ id: id++, text: 'Learn Vue', done: false }
])
//用计算属性确定呈现的数据数组,如果hideCompleted.value的值是true就过滤出done为false的项,否则保持原样。
const filteredTodos = computed(() => {
return hideCompleted.value
? todos.value.filter((t) => t.done==false)
: todos.value
})
function addTodo() {
todos.value.push({ id: id++, text: newTodo.value, done: false })
newTodo.value = ''
}
function removeTodo(todo) {
todos.value = todos.value.filter((t) => t !== todo)
}
</script>
<template>
<form @submit.prevent="addTodo">
<input v-model="newTodo" required placeholder="new todo">
<button>Add Todo</button>
</form>
<ul>
<li v-for="todo in filteredTodos" :key="todo.id">
//添加一个checkbox,绑定todo的done
<input type="checkbox" v-model="todo.done">
//通过done的值来给该元素加类,类里实现属性line-through
<span :class="{ done: todo.done }">{{ todo.text }}</span>
<button @click="removeTodo(todo)">X</button>
</li>
</ul>
//通过按钮控制控制展示内容(按钮文字及计算的数组内容)
<button @click="hideCompleted = !hideCompleted">
{{ hideCompleted ? 'Show all' : 'Hide completed' }}
</button>
</template>
<style>
.done {
text-decoration: line-through;
}
</style>
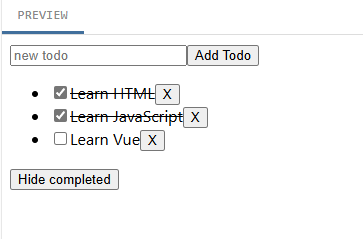