题目
- 给你一个二叉树的根节点 root ,树中每个节点都存放有一个 0 到 9 之间的数字。
每条从根节点到叶节点的路径都代表一个数字:
例如,从根节点到叶节点的路径 1 -> 2 -> 3 表示数字 123 。
计算从根节点到叶节点生成的 所有数字之和 。
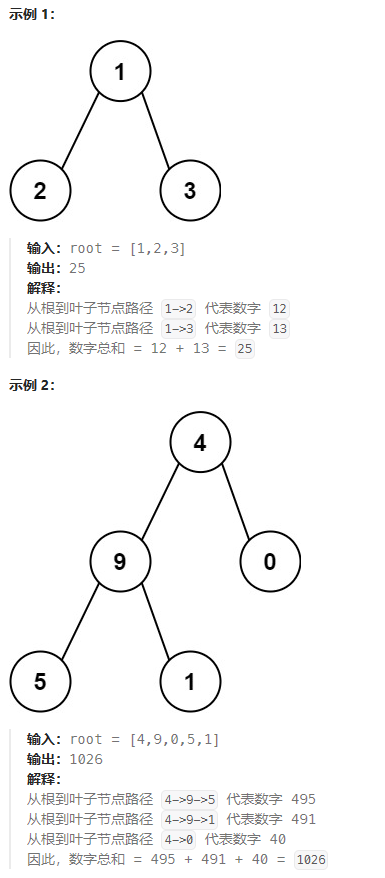
题解:回溯
class Solution:
def sumNumbers(self, root: TreeNode) -> int:
res = 0 # 用于保存最终的结果
path = [] # 用于保存当前路径上的节点值
def backtrace(root):
nonlocal res # nonlocal在嵌套函数内引用外部函数中的 res 变量
if not root:
return # 节点为空则返回
path.append(root.val) # 将当前节点值添加到路径中
if not root.left and not root.right: # 遇到叶子节点
res += get_sum(path) # 将路径上的节点值组成数字并累加到结果中
if root.left: # 左子树不为空
backtrace(root.left) # 递归遍历左子树
if root.right: # 右子树不为空
backtrace(root.right) # 递归遍历右子树
path.pop() # 回溯,将最后一个节点从路径中弹出
def get_sum(arr):
s = 0
for i in range(len(arr)):
s = s * 10 + arr[i] # 将路径上的节点值组成数字
return s
backtrace(root) # 调用回溯函数开始遍历二叉树
return res # 返回最终的结果