WinForm 過濾數據 / 按Del鍵刪除信息
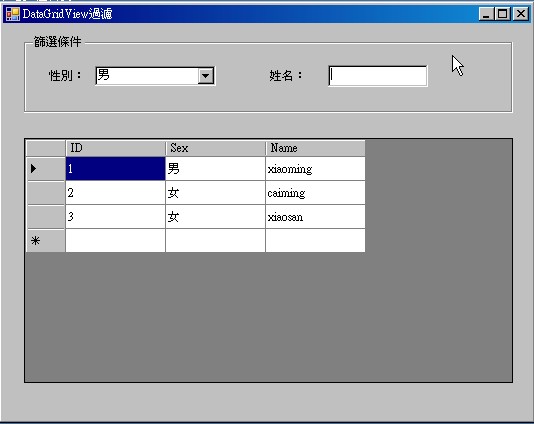

1 public partial class Form1 : Form
2 {
3 DataView dv = null;
4 public Form1()
5 {
6 InitializeComponent();
7 }
8
9 private void Form1_Load(object sender, EventArgs e)
10 {
11 this.comboBox1.DataSource = LoadSex();
12 this.comboBox1.DisplayMember = "text";
13 this.comboBox1.ValueMember = "ID";
14 this.dataGridView1.DataSource = LoadData();
15
16 }
17 /// <summary>
18 /// 加載數據
19 /// </summary>
20 /// <returns></returns>
21 private DataView LoadData()
22 {
23 DataTable dt = new DataTable();
24
25 DataColumn dc = new DataColumn("ID",typeof(Int32));
26 dt.Columns.Add(dc);
27 DataColumn dc2 = new DataColumn("Sex", typeof(string));
28 dt.Columns.Add(dc2);
29 DataColumn dc3 = new DataColumn("Name",typeof(string));
30 dt.Columns.Add(dc3);
31
32 DataRow dr1 = dt.NewRow();
33 dr1["ID"] = 1;
34 dr1["Sex"] = "男";
35 dr1["Name"] = "xiaoming";
36 dt.Rows.Add(dr1);
37
38 DataRow dr2 = dt.NewRow();
39 dr2["ID"] = 2;
40 dr2["Sex"] = "女";
41 dr2["Name"] = "caiming";
42 dt.Rows.Add(dr2);
43
44 DataRow dr3 = dt.NewRow();
45 dr3["ID"] = 3;
46 dr3["Sex"] = "女";
47 dr3["Name"] = "xiaosan";
48 dt.Rows.Add(dr3);
49
50 dv = dt.DefaultView;
51
52 return dv;
53 }
54
55 private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
56 {
57 Filter_Data();
58 }
59 /// <summary>
60 /// 過濾數據方法
61 /// </summary>
62 private void Filter_Data()
63 {
64 //判斷DV是否為空
65 if (dv == null)
66 return;
67 dv.RowFilter = string.Format(" Name like '%{1}%' and Sex like '%{0}%'", this.comboBox1.SelectedValue.ToString(), this.textBox1.Text);
68
69 }
70
71 private DataTable LoadSex()
72 {
73 DataTable dt = new DataTable();
74 DataColumn dc = new DataColumn("text",typeof(string));
75 dt.Columns.Add(dc);
76 DataColumn dc2 = new DataColumn("ID",typeof(string));
77 dt.Columns.Add(dc2);
78 DataRow dr = dt.NewRow();
79 dr["text"] = "男";
80 dr["ID"] = "男";
81 dt.Rows.Add(dr);
82 DataRow dr2 = dt.NewRow();
83 dr2["text"] = "女";
84 dr2["ID"] = "女";
85 dt.Rows.Add(dr2);
86 return dt;
87 }
88
89 private void textBox1_TextChanged(object sender, EventArgs e)
90 {
91 Filter_Data();
92 }
93
94 private void dataGridView1_KeyUp(object sender, KeyEventArgs e)
95 {
96
97 List<int> RowIndexs = new List<int>();
98
99 int rowIndex=-1;
100 if (e.KeyData == Keys.Delete)
101 {
102 DialogResult DelDr = MessageBox.Show(AlertMessage.DelMessage.delMessage, "提示", MessageBoxButtons.OKCancel, MessageBoxIcon.Information);
103 if (DelDr == DialogResult.OK)
104 {
105 //獲得主鍵
106 if (this.dataGridView1.SelectedCells.Count > 0)
107 {
108 for(int i = 0;i<this.dataGridView1.SelectedCells.Count;i++)
109 {
110 rowIndex = this.dataGridView1.SelectedCells[i].RowIndex;
111 RowIndexs.Add(rowIndex);
112
113 }
114
115 }
116 else if (this.dataGridView1.SelectedColumns.Count > 0)
117 rowIndex = this.dataGridView1.SelectedColumns[0].Index;
118
119 //获得改行的主键
120 List<int> primaryKeys = new List<int>();
121 foreach (int rowIndex2 in RowIndexs)
122 {
123 int primaryKey = int.Parse(this.dataGridView1.Rows[rowIndex2].Cells["ID"].Value.ToString());
124 primaryKeys.Add(primaryKey);
125 }
126
127
128 if (dv == null)
129 return;
130 string DelPrimaryKey = "";
131 foreach (int primaryKey2 in primaryKeys)
132 {
133 DelPrimaryKey += primaryKey2.ToString()+',';
134 }
135 DelPrimaryKey += "-1";
136
137 dv.RowFilter = string.Format("ID not in ({0}) ", DelPrimaryKey);
138 }
139
140 }
141 }
142
143
144