实验3
任务1
1 #include<stdio.h> 2 #include<math.h> 3 #include<time.h> 4 #include<stdlib.h> 5 char score_to_grade(int score); 6 7 8 int main() 9 { 10 int score; 11 char grade; 12 while(scanf("%d",&score)!=EOF) 13 { 14 grade=score_to_grade(score); 15 printf("分数:%d,等级:%c\n\n",score,grade); 16 } 17 return 0; 18 } 19 20 char score_to_grade(int score) 21 { 22 char ans; 23 switch(score/10){ 24 case 10: 25 case 9: ans='A';break; 26 case 8: ans='B';break; 27 case 7: ans='C';break; 28 case 6: ans='D';break; 29 default: ans='E'; 30 31 } 32 return ans; 33 } 34
问题1:函数score_to_grade的功能是根据不同的分数段将分数转化为评分,形参类型为整型,返回值类型为字符型
问题2:有问题,如果没有break语句无论哪种情况都会一直执行到Switch结构结束,均输出E
任务2
1 #include<stdio.h> 2 #include<math.h> 3 #include<time.h> 4 #include<stdlib.h> 5 6 7 int sum_digits(int n); 8 9 int main() 10 { 11 int n; 12 int ans; 13 14 while(printf("Enter n:"),scanf("%d",&n)!=EOF) 15 { 16 ans=sum_digits(n); 17 printf("n=%d,ans=%d\n\n",n,ans); 18 19 } 20 21 return 0; 22 } 23 24 int sum_digits(int n) 25 { 26 int ans=0; 27 while(n!=0) 28 { 29 ans+=n%10; 30 n/=10; 31 } 32 return ans; 33 } 34
问题1:函数sum_digits的功能是将一个数每个位数上的数相加并输出
问题2:能实现相同的效果
前者是迭代的思维,后者是递归的思维
任务3
1 #include<stdio.h> 2 #include<math.h> 3 #include<time.h> 4 #include<stdlib.h> 5 6 7 int power(int x,int n); 8 9 10 int main() 11 { 12 int x,n; 13 int ans; 14 15 while(printf("Enter x and n:"),scanf("%d%d",&x,&n)!=EOF) 16 { 17 ans=power(x,n); 18 printf("n=%d,ans=%d\n\n",n,ans); 19 20 } 21 return 0; 22 } 23 int power(int x,int n) 24 { 25 int t; 26 if(n==0) 27 return 1; 28 else if(n%2) 29 return x*power(x,n-1); 30 else{ 31 t=power(x,n/2); 32 return t*t; 33 } 34 }
问题1:power函数的作用是运算x的n次方的值并输出
问题2:power函数是递归函数,数学模型:
当n==0,任何数的 0 次幂都为 1,所以函数直接返回 1;
当n为奇数,x^n==x*x^(n-1)
当n为偶数,x^n=(x^(n/2))^2
任务4
1 #include<stdio.h> 2 #include<math.h> 3 #include<time.h> 4 #include<stdlib.h> 5 int is_prime(int n); 6 int main() 7 { 8 int i,sum=0; 9 printf("100以内的孪生素数:\n"); 10 for(i=2;i<=98;++i) 11 { 12 if(is_prime(i)&&is_prime(i+2)) 13 { 14 printf("%d %d\n",i,i+2); 15 sum+=1; 16 } 17 else 18 { 19 continue; 20 } 21 22 23 } 24 printf("100以内的孪生素数共有%d组\n",sum); 25 26 return 0; 27 } 28 29 30 int is_prime(int n) { 31 if (n <= 1) { 32 return 0; 33 } 34 for (int i=2; i<=sqrt(1.0*n); i++) { 35 if (n % i == 0) { 36 return 0; 37 } 38 } 39 return 1; 40 }
任务5
1 #include<stdio.h> 2 #include<math.h> 3 #include<time.h> 4 #include<stdlib.h> 5 unsigned int move_count = 0; 6 void hanoi(unsigned int n, char from, char temp, char to); 7 void moveplate(unsigned int n, char from, char to); 8 int main() 9 { 10 unsigned int n; 11 while(scanf("%u",&n)!=EOF) 12 { 13 move_count = 0; 14 hanoi(n, 'A','B','C'); 15 printf("一共移动了: %u次\n", move_count); 16 getchar(); 17 } 18 19 return 0; 20 } 21 22 23 void hanoi(unsigned int n, char from, char temp, char to) 24 { 25 26 if(n==1) 27 moveplate(n, from, to); 28 else 29 { 30 hanoi(n-1,from, to, temp); 31 moveplate(n, from, to); 32 hanoi(n-1,temp,from, to); 33 } 34 } 35 36 37 void moveplate(unsigned int n, char from, char to) 38 { 39 printf(" %u:%c-->%c\n", n, from, to); 40 41 move_count++; 42 } 43 44 45 46 47 48 49 50
任务6
递归实现:
1 #include<stdio.h> 2 #include<math.h> 3 #include<time.h> 4 #include<stdlib.h> 5 int func(int n,int m); 6 7 int main() 8 { 9 int n,m; 10 int ans; 11 while(scanf("%d%d",&n,&m)!=EOF) 12 { 13 ans=func(n,m); 14 printf("n=%d,m=%d,ans=%d\n\n",n,m,ans); 15 16 } 17 18 return 0; 19 } 20 21 22 int func(int n,int m) 23 { 24 25 if(m==0||m==n) 26 return 1; 27 else if(n<m) 28 return 0; 29 else 30 return func( n-1, m)+func( n-1,m-1); 31 32 33 34 }
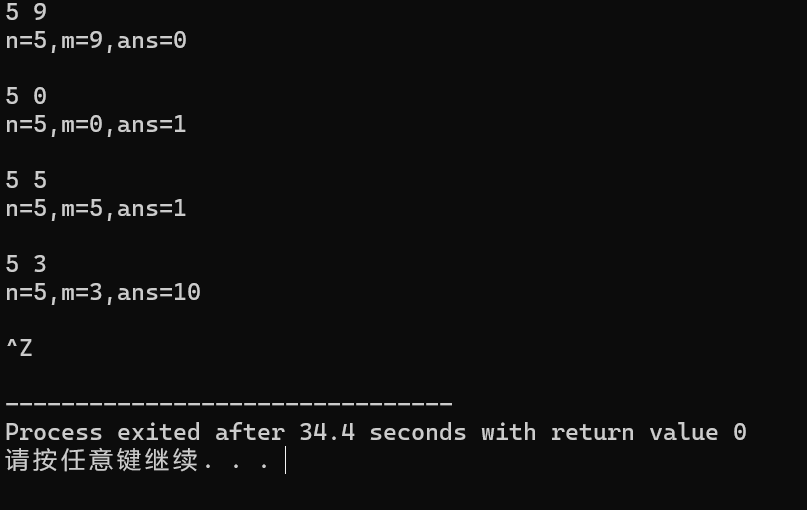
迭代实现:
1 #include<stdio.h> 2 #include<math.h> 3 #include<time.h> 4 #include<stdlib.h> 5 int func(int n); 6 7 int main() 8 { 9 int n,m; 10 int ans; 11 while(scanf("%d%d",&n,&m)!=EOF) 12 { 13 ans=func(n)/func(m)/func(n-m); 14 printf("n=%d,m=%d,ans=%d\n\n",n,m,ans); 15 16 } 17 18 return 0; 19 } 20 21 22 int func(int n) 23 { int i; 24 int ans=1; 25 26 for(i=1;i<=n;++i) 27 { 28 ans*=i; 29 } 30 return ans; 31 32 }