寒假小软件
彩票模拟
编程前PSP:
PSP2.1 |
Personal Software Process Stages |
Time |
Planning |
计划 |
15min |
· Estimate |
· 估计这个任务需要多少时间 |
10h |
Development |
开发 |
10h |
· Analysis |
· 需求分析 (包括学习新技术) |
1h |
· Design Spec |
· 生成设计文档 |
1h |
· Design Review |
· 设计复审 (和同事审核设计文档) |
1h |
· Coding Standard |
· 代码规范 (为目前的开发制定合适的规范) |
1h |
· Design |
· 具体设计 |
2h |
· Coding |
· 具体编码 |
1h |
· Code Review |
· 代码复审 |
1h |
· Test |
· 测试(自我测试,修改代码,提交修改) |
1h |
Reporting |
报告 |
1h |
· Test Report |
· 测试报告 |
20min |
· Size Measurement |
· 计算工作量 |
20min |
· Postmortem & Process Improvement Plan |
· 事后总结, 并提出过程改进计划 |
20min |
|
合计 |
10h |
双色球模拟
package balls; import java.util.Arrays; import java.util.Random; public class Ball1 { public String ball1() { String[] pool = {"01","02","03","04","05", "06","07","08","09","10", "11","12","13","14","15", "16","17","18","19","20", "21","22","23","24","25", "26","27","28","29","30", "31","32","33"}; boolean[] used = new boolean[pool.length]; String[] balls = new String[6]; Random random = new Random(); int i; int index = 0; while(true){ i = random.nextInt(pool.length); if(used[i]){ continue; } balls[index++] =pool[i]; used[i] = true; if(index == balls.length){ break; } } Arrays.sort(balls); String[] newBalls = new String[7]; for(int m = 0; m < balls.length; m++){ newBalls[m] = balls[m]; } newBalls[newBalls.length - 1] = pool[random.nextInt(16)]; String r = Arrays.toString(newBalls); return r; } }
大乐透模拟
package balls; import java.util.Arrays; import java.util.Random; public class Ball2 { public String ball2() { String[] pool = {"01","02","03","04","05", "06","07","08","09","10", "11","12","13","14","15", "16","17","18","19","20", "21","22","23","24","25", "26","27","28","29","30", "31","32","33","34","35"}; boolean[] used = new boolean[pool.length]; String[] balls = new String[5]; Random random = new Random(); int i; int index = 0; while(true){ i = random.nextInt(pool.length); if(used[i]){ continue; } balls[index++] =pool[i]; used[i] = true; if(index == balls.length){ break; } } Arrays.sort(balls); String[] newBalls = new String[7]; for(int m = 0; m < balls.length; m++){ newBalls[m] = balls[m]; } newBalls[newBalls.length - 2] = pool[random.nextInt(12)]; while(true){ String j = pool[random.nextInt(12)]; if(j == newBalls[5]){ continue; }else{ newBalls[6] = j; break; } } /*if(newBalls[5] > (newBalls[6]){ String x = newBalls[5]; newBalls[5] = newBalls[6]; newBalls[6] = x; }*/ String r = Arrays.toString(newBalls); return r; } }
java JFrame窗口
package window; import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.imageio.ImageIO; import java.io.IOException; import balls.*; public class BallsWindow extends JFrame { public void Window() { /*标题 String path = "/Img/彩票.jpg"; try { Image img = ImageIO.read(this.getClass().getResource(path)); this.setIconImage(img); } catch (IOException e) { e.printStackTrace(); } //this.setIconImage(Toolkit.getDefaultToolkit().getImage("E:\\彩票.jpg")); */ this.setTitle("我要中大奖"); //窗口控制 //this.setBounds(300, 200, 580, 600); this.setLocation(500, 100); this.setSize(500, 300); this.setResizable(false); this.setLayout(new FlowLayout()); setLayout(null); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); this.setVisible(true); //按钮控制 Button bu1 = new Button("双色球"); bu1.setFont(new Font("宋体", Font.BOLD, 20)); bu1.setBounds(100,70,100,100); Button bu2 = new Button("大乐透"); bu2.setFont(new Font("宋体", Font.BOLD, 20)); bu2.setBounds(280,70,100,100); this.add(bu1); this.add(bu2); bu1.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { JTextField jtf1;//文本框 JFrame frame1 = new JFrame("双色球"); frame1.setVisible(true); frame1.setLocation(500, 100); frame1.setSize(500, 300); frame1.setDefaultCloseOperation(EXIT_ON_CLOSE); frame1.setLayout(new FlowLayout()); // 第一行 JLabel jl1 = new JLabel("输入模拟次数"); jtf1 = new JTextField(12); frame1.add(jl1); frame1.add(jtf1); // 第二行 JButton jb1, jb2;//按钮 jb1 = new JButton("确定"); jb1.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String cmd = e.getActionCommand();// 根据动作命令,来进行分别处理 if (cmd.equals("确定")) { String n = jtf1.getText(); int m = Integer.parseInt( n ); JFrame frame1 = new JFrame("双色球"); frame1.setVisible(true); frame1.setLocation(500, 100); frame1.setSize(500, 300); frame1.setDefaultCloseOperation(EXIT_ON_CLOSE); frame1.setLayout(new FlowLayout()); JTextArea jta = new JTextArea(50,14); Ball1 b1 = new Ball1(); jta.append(" ---------红球----------蓝球-"+"\n"); for(int i = 0; i < m; i++) { jta.append(b1.ball1()+"\n"); } frame1.add(jta); } }});// 添加动作响应器 jb2 = new JButton("重置"); jb2.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String cmd = e.getActionCommand();// 根据动作命令,来进行分别处理 if (cmd.equals("重置")) { jtf1.setText(""); } }});// 添加动作响应器 frame1.add(jb1); frame1.add(jb2); } }); bu2.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { JTextField jtf1;//文本框 JFrame frame2 = new JFrame("大乐透"); frame2.setVisible(true); frame2.setLocation(500, 100); frame2.setSize(500, 300); frame2.setDefaultCloseOperation(EXIT_ON_CLOSE); frame2.setLayout(new FlowLayout()); // 第一行 JLabel jl1 = new JLabel("输入模拟次数"); jtf1 = new JTextField(12); frame2.add(jl1); frame2.add(jtf1); // 第二行 JButton jb1, jb2;//按钮 jb1 = new JButton("确定"); jb1.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String cmd = e.getActionCommand();// 根据动作命令,来进行分别处理 if (cmd.equals("确定")) { String n = jtf1.getText(); int m = Integer.parseInt( n ); JFrame frame2 = new JFrame("大乐透"); frame2.setVisible(true); frame2.setLocation(500, 100); frame2.setSize(500, 300); frame2.setDefaultCloseOperation(EXIT_ON_CLOSE); frame2.setLayout(new FlowLayout()); JTextArea jta = new JTextArea(50,14); Ball2 b2 = new Ball2(); jta.append(" ----------前区--------*后区*"+"\n"); for(int i = 0; i < m; i++) { jta.append(b2.ball2()+"\n"); } frame2.add(jta); } }});// 添加动作响应器 jb2 = new JButton("重置"); jb2.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String cmd = e.getActionCommand();// 根据动作命令,来进行分别处理 if (cmd.equals("重置")) { jtf1.setText(""); } }});// 添加动作响应器 frame2.add(jb1); frame2.add(jb2); } }); } }
主函数
package play; import window.BallsWindow; public class BallsPlay { public static void main(String[] args) { BallsWindow b = new BallsWindow(); b.Window(); } }
结果:
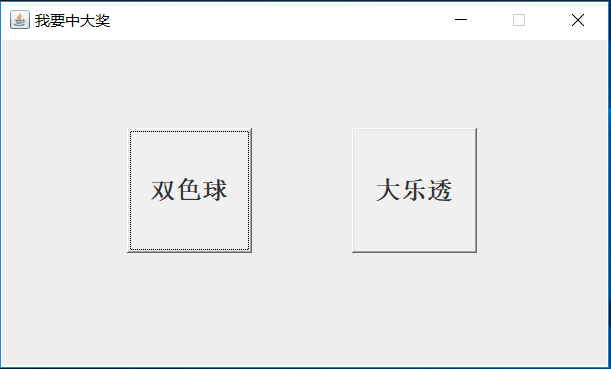
编程后PSP:
PSP2.1 |
Personal Software Process Stages |
Time |
Planning |
计划 |
15min |
· Estimate |
· 估计这个任务需要多少时间 |
10h |
Development |
开发 |
10h |
· Analysis |
· 需求分析 (包括学习新技术) |
1h |
· Design Spec |
· 生成设计文档 |
1h |
· Design Review |
· 设计复审 (和同事审核设计文档) |
1h |
· Coding Standard |
· 代码规范 (为目前的开发制定合适的规范) |
1h |
· Design |
· 具体设计 |
2h |
· Coding |
· 具体编码 |
1h |
· Code Review |
· 代码复审 |
1h |
· Test |
· 测试(自我测试,修改代码,提交修改) |
1h |
Reporting |
报告 |
1h |
· Test Report |
· 测试报告 |
20min |
· Size Measurement |
· 计算工作量 |
20min |
· Postmortem & Process Improvement Plan |
· 事后总结, 并提出过程改进计划 |
20min |
|
合计 |
10h |
缺陷记录日志
①界面不美观,字体大小没有调整的好