"""
Created on Fri Jan 12 17:21:11 2018
@author: Administrator
"""
"""
Created on Thu Jan 11 11:09:36 2018
@author: Administrator
"""
import pymongo
import matplotlib.pyplot as plt
import pandas
import random
client136 = pymongo.MongoClient('192.168.0.136',27017)
db136 = client136.fangjia
dsf136 = db136.average_statistics
ct='上海'
rl='徐汇'
lk='嘉创华狮国际公寓'
query1 = {"city":ct,'region':rl,'name':lk}
fields={ 'city':1,'region':1,'name':1 ,
'level':1,'fangjia_price':1,'s_date':1}
lct= list(dsf136.find(query1,fields))
df=pandas.DataFrame(lct).dropna()
import numpy as np
def matrix_lstsqr(x, y):
X = np.vstack([x, np.ones(len(x))]).T
return (np.linalg.inv(X.T.dot(X)).dot(X.T)).dot(y)
def classic_lstsqr(x_list, y_list):
N = len(x_list)
x_avg = sum(x_list)/N
y_avg = sum(y_list)/N
var_x, cov_xy = 0, 0
for x,y in zip(x_list, y_list):
temp = x - x_avg
var_x += temp**2
cov_xy += temp * (y - y_avg)
slope = cov_xy / var_x
y_interc = y_avg - slope*x_avg
return (slope, y_interc)
"""
x=list(df.index)
y=list(df['fangjia_price'])
#slope, intercept = matrix_lstsqr(x, y)
slope, intercept = classic_lstsqr(x, y) # 或用classic_lstsqr
print('slope=',slope, 'intercept=',intercept)
"""
random.seed(12345)
x = [x_i*random.randrange(8,12)/10 for x_i in range(500)]
y = [y_i*random.randrange(8,12)/10 for y_i in range(100,600)]
slope, intercept = matrix_lstsqr(x, y)
line_x = [round(min(x)) - 1, round(max(x)) + 1]
line_y = [slope*x_i + intercept for x_i in line_x]
plt.figure(figsize=(8,8))
plt.scatter(x,y)
plt.plot(line_x, line_y, color='red', lw='2')
plt.ylabel('y')
plt.xlabel('x')
plt.title('Linear regression via least squares fit')
plt.show()
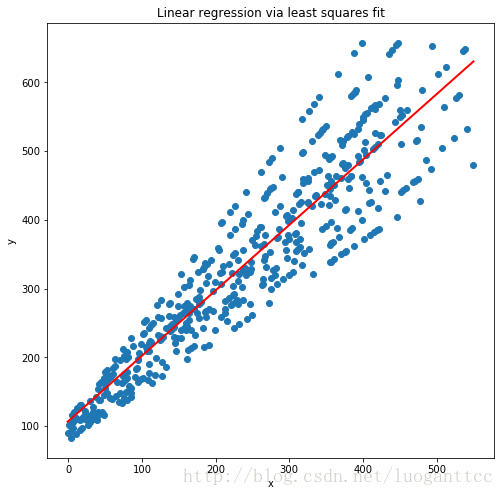