1. 绑定到其它元素
1 |
< Grid > |
2 |
< StackPanel > |
3 |
< TextBox x:Name = "textbox1" /> |
4 |
< Label Content = "{Binding ElementName=textbox1, Path=Text}" /> |
5 |
</ StackPanel > |
6 |
</ Grid > |
2. 绑定到静态资源
1 |
< Window.Resources > |
2 |
< ContentControl x:Key = "text" >Hello, World!</ ContentControl > |
3 |
</ Window.Resources > |
4 |
< Grid > |
5 |
< StackPanel > |
6 |
< Label x:Name = "label1" Content = "{Binding Source={StaticResource text}}" /> |
7 |
</ StackPanel > |
8 |
</ Grid > |
9 |
< STRONG >3. 绑定到自身</ STRONG > |
1 |
< Grid > |
2 |
< StackPanel > |
3 |
< Label x:Name = "label1" Content = "{Binding RelativeSource={RelativeSource Self}, Path=Name}" /> |
4 |
</ StackPanel > |
5 |
</ Grid > |
4. 绑定到指定类型的父元素
1 |
< Grid x:Name = "Grid1" > |
2 |
< StackPanel > |
3 |
< Label x:Name = "label1" Content="{Binding RelativeSource={RelativeSource FindAncestor, |
4 |
AncestorType={x:Type Grid}}, Path = Name }" /> |
5 |
</ StackPanel > |
6 |
</ Grid > |
5. 绑定到对象
1 |
public class Person |
2 |
{ |
3 |
public string Name { get ; set ; } |
4 |
public int Age { get ; set ; } |
5 |
} |
1 |
< StackPanel x:Name = "stackPanel" > |
2 |
< StackPanel.DataContext > |
3 |
< local:Person Name = "Jack" Age = "30" ></ local:Person > |
4 |
</ StackPanel.DataContext > |
5 |
< TextBlock Text = "{Binding Path=Name}" ></ TextBlock > |
6 |
< TextBlock Text = "{Binding Path=Age}" ></ TextBlock > |
7 |
|
8 |
</ StackPanel > |
6. 绑定到集合
1 |
public class Person |
2 |
{ |
3 |
public string Name { get ; set ; } |
4 |
public int Age { get ; set ; } |
5 |
} |
6 |
|
7 |
public class PersonList : ObservableCollection<Person> |
8 |
{ } |
01 |
< Window.Resources > |
02 |
< local:PersonList x:Key = "person" > |
03 |
< local:Person Name = "Jack" Age = "30" ></ local:Person > |
04 |
< local:Person Name = "Tom" Age = "32" ></ local:Person > |
05 |
</ local:PersonList > |
06 |
</ Window.Resources > |
07 |
< StackPanel x:Name = "stackPanel" > |
08 |
< ListBox ItemsSource = "{Binding Source={StaticResource ResourceKey=person}}" |
09 |
DisplayMemberPath = "Name" > |
10 |
</ ListBox > |
11 |
</ StackPanel > |
7. DataContext共享源
我们需要将同一资源绑定到多个 UI 元素上,很显然到处写 "{Binding Source={StaticResource person}}" 是件很繁琐且不利于修改的做法。WPF 提供了一个称之为 "数据上下文 (DataContext)" 的东西让我们可以在多个元素上共享一个源对象,只需将其放到父元素 DataContext 属性即可。当我们不给 Binding 扩展标志指定 Source 属性时,它会自动寻找上级父元素的数据上下文。
01 |
< Window.Resources > |
02 |
< local:PersonList x:Key = "person" > |
03 |
< local:Person Name = "Jack" Age = "30" ></ local:Person > |
04 |
< local:Person Name = "Tom" Age = "32" ></ local:Person > |
05 |
</ local:PersonList > |
06 |
</ Window.Resources > |
07 |
< StackPanel x:Name = "stackPanel" DataContext = "{StaticResource person}" > |
08 |
< ListBox ItemsSource = "{Binding}" |
09 |
DisplayMemberPath = "Name" > |
10 |
</ ListBox > |
11 |
</ StackPanel > |
8. 使用XML作为Binding的源
XML:
01 |
<? xml version = "1.0" encoding = "utf-8" ?> |
02 |
< PersonList > |
03 |
< Person Id = "1" > |
04 |
< Name >Jack</ Name > |
05 |
</ Person > |
06 |
< Person Id = "2" > |
07 |
< Name >Tom</ Name > |
08 |
</ Person > |
09 |
< Person Id = "3" > |
10 |
< Name >Justin</ Name > |
11 |
</ Person > |
12 |
< Person Id = "4" > |
13 |
< Name >David</ Name > |
14 |
</ Person > |
15 |
</ PersonList > |
XAML:
01 |
< StackPanel > |
02 |
< ListView x:Name = "personListView" > |
03 |
< ListView.View > |
04 |
< GridView > |
05 |
< GridViewColumn Header = "Id" Width = "100" |
06 |
DisplayMemberBinding = "{Binding XPath=@Id}" /> |
07 |
< GridViewColumn Header = "Name" Width = "100" |
08 |
DisplayMemberBinding = "{Binding XPath=Name}" /> |
09 |
</ GridView > |
10 |
</ ListView.View > |
11 |
</ ListView > |
12 |
< Button Click = "Button_Click" >Load Data</ Button > |
13 |
</ StackPanel > |
后台代码:
01 |
private void Button_Click( object sender, RoutedEventArgs e) |
02 |
{ |
03 |
XmlDocument xmlDocument = new XmlDocument(); |
04 |
xmlDocument.Load( "Person.xml" ); |
05 |
|
06 |
XmlDataProvider xdp = new XmlDataProvider(); |
07 |
xdp.Document = xmlDocument; |
08 |
xdp.XPath = @"/PersonList/Person" ; |
09 |
|
10 |
this .personListView.DataContext = xdp; |
11 |
this .personListView.SetBinding(ListView.ItemsSourceProperty, new Binding()); |
12 |
} |
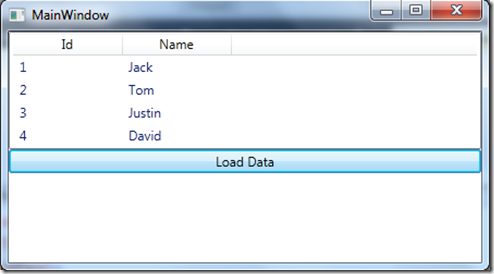