12.1 控制器介绍 — 精通 MVC 3 框架
Introducing the Controller
控制器介绍
You’ve seen the use of controllers in almost all of the chapters so far. Now it is time to take a step back and look behind the scenes. To begin, we need to create a project for our examples.
到目前为止几乎所有的章节中,你都已经在看到了控制器的使用。现在到了回过头来考查场景背后的东西的时候了。一开始,我们需要生成一个例子项目。
Preparing the Project
准备项目
To prepare for this chapter, we created a new MVC 3 project using the Empty template and called the project ControllersAndActions. We chose the Empty template because we are going to create all of the controllers and views we need as we go through the chapter.
为了做好本章的准备,我们用空模板生成了一个新的MVC 3项目,并将之称为ControllersAndActions。我们选择空模板是因为,随着本章的进行,我们要生成所有控制器和视图。
Creating a Controller with IController
生成一个带有IController的控制器
In the MVC Framework, controller classes must implement the IController interface from the System.Web.Mvc namespace, as shown in Listing 12-1.
在MVC框架中,控制器类必须实现System.Web.Mvc命名空间的IController接口,如清单12-1所示。
Listing 12-1. The System.Web.Mvc.IController Interface
public interface IController { void Execute(RequestContext requestContext); }
This is a very simple interface. The sole method, Execute, is invoked when a request is targeted at the controller class. The MVC Framework knows which controller class has been targeted in a request by reading the value of the controller property generated by the routing data.
这是一个很简单的接口。它只有一个单一的方法,Execute,当一个请求以这个控制器类为目标时被调用。MVC框架通过读取由路由数据生成的controller属性的值,会知道一个请求的目标是哪个控制器。
You can choose to create controller classes by implementing IController, but it is a pretty low-level interface, and you must do a lot of work to get anything useful done. Listing 12-2 shows a simple controller called BasicController that provides a demonstration.
通过实现IController,你可以选择自己去生成控制器类,但这是一个相当低级的接口,因此你必须做很多工作才能让事情变得有用。清单12-2显示了一个名为BasicController的简单控制器,它提供了一个演示。
Listing 12-2. The BasicController Class
using System.Web.Mvc; using System.Web.Routing; namespace ControllersAndActions.Controllers { public class BasicController : IController { public void Execute(RequestContext requestContext) { string controller = (string)requestContext.RouteData.Values["controller"]; string action = (string)requestContext.RouteData.Values["action"]; requestContext.HttpContext.Response.Write( string.Format("Controller: {0}, Action: {1}", controller, action)); } } }
To create this class, we right-clicked the Controllers folder in the example project and selected Add →New Class. We then gave the name for our controller, BasicController, and entered the code you see in Listing 12-2.
为了生成这个类,我们右击例子项目中的Controllers文件夹,并选择“添加”→“新类”。然后给出该控制器的名字,BasicController,并输入你在清单12-2中所看到的代码。
In our Execute method, we read the value of the controller and action variables from the RouteData object associated with the request and write them to the result. If you run the application and navigate to /Basic/Index, you can see the output generated by our controller, as shown in Figure 12-1.
在我们的Execute方法中,我们从与这个请求相关联的RouteData对象读取了controller和action变量的值,并把它们写到结果。如果你运行这个应用程序,并导航到Basic/Index,你可以看到该控制器所生成的输出,如图12-1所示。
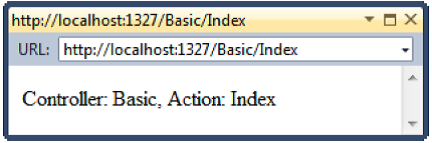
Figure 12-1. A result generated from the BasicController class
Implementing the IController interface allows you to create a class that the MVC Framework recognizes as a controller and sends requests to, but it would be pretty hard to write a complex application. The MVC Framework doesn’t specify how a controller deals with requests, which means that you can create any approach you want.
实现IController接口允许你生成一个类,以使MVC框架把它看作是一个控制器,并把请求发送给它,但编写一个复杂的应用程序是相当困难的。MVC框架并未指定一个控制器如何处理请求,这意味着你可以采用任何办法来处理请求。
Creating a Controller by Deriving from the Controller Class
通过从Controller类派生的办法生成一个控制器
The MVC Framework is endlessly customizable and extensible. You can implement the IController interface to create any kind of request handling and result generation you require. Don’t like action methods? Don’t care for rendered views? Then you can just take matters in your own hands and write a better, faster, and more elegant way of handling requests. Or you could use the features that the MVC Framework team has provided, which is achieved by deriving your controllers from the System.Web.Mvc.Controller class.
MVC框架是无限可定制和可扩展的。你可以实现IController接口来生成你需要的各种请求处理和结果生成。不喜欢动作方法?不关心渲染的视图?那么,你可以把事情掌握在自己的手中,并编写请求处理更好、更快、以及更雅致的方式。或者,你可以使用MVC框架团队已经提供的特性,这是通过从System.Web.Mvc.Controller类派生你的控制器来获得的。
System.Web.Mvc.Controller is the class that provides the request handling support that most MVC developers will be familiar with. It’s what we have been using in all of our examples in previous chapters.
System.Web.Mvc.Controller是大多数MVC开发人员需要熟悉的用来提供请求处理支持的类。这是我们前面章节中所有例子一直在使用的一个类。
The Controller class provides three key features:
Controller类提供了三个关键特性:
- Action methods: A controller’s behavior is partitioned into multiple methods (instead of having just one single Execute() method). Each action method is exposed on a different URL and is invoked with parameters extracted from the incoming request.
动作方法:一个控制器的行为被分解成多个方法(而不是只有一个单一的Execute()方法)。每个动作方法被暴露给不同的URL,并通过从输入请求提取的参数进行调用。 - Action results: You can return an object describing the result of an action (for example, rendering a view, or redirecting to a different URL or action method), which is then carried out on your behalf. The separation between specifying results and executing them simplifies unit testing.
动作结果:你可以返回一个描述动作结果的对象(例如,渲染一个视图、或重定向到一个不同的URL或动作方法),然后通过该对象实现你的目的。这种指定结果和执行它们之间的分离简化了单元测试。 - Filters: You can encapsulate reusable behaviors (for example, authentication, as you saw in Chapter 9) as filters, and then tag each behavior onto one or more controllers or action methods by putting an [Attribute] in your source code.
过滤器:你可以把可重用的行为(例如,认证,正如你在第9章所看到的那样)封装成过滤器,然后通过在你的源代码中放置一个[Attribute]的办法,把各个行为连接在一个或多个控制或动作方法上。
Unless you have a very specific requirement in mind, the best way to create controllers is to derive from the Controller class, and, as you might hope, this is what Visual Studio does when it creates a new class in response to the Add → Controller menu item. Listing 12-3 shows a simple controller created this way. We have called our class DerivedController.
除非你在头脑中有一个非常明确的需求,生成控制器最好的办法是通过Controller类进行派生,而且,正如你可能希望的,这就是Visual Studio在响应“添加”→“控制器”菜单项生成一个新类时为你所做的事情。清单12-3显示了用这种办法生成的一个简单的控制器。我们称之为DerivedController。
Listing 12-3. A Simple Controller Derived from the System.Web.Mvc.Controller Class
using System.Web.Mvc; namespace ControllersAndActions.Controllers { public class DerivedController : Controller { public ActionResult Index() { ViewBag.Message = "Hello from the DerivedController Index method"; return View("MyView"); } } }
The Controller base class implements the Execute method and takes responsibility for invoking the action method whose name matches the action value in the route data.
Controller基类实现了Execute方法,并负责调用名字与路由数据中的action值匹配的动作方法。
The Controller class is also our link to the view system. In the listing, we return the result of the View method, passing in the name of the view we want rendered to the client as a parameter. Listing 12-4 shows this view, called MyView.cshtml and located in the Views/Derived folder in the project.
Controller类也是我们通向视图系统的连接。在上述清单中,我们返回了View方法的结果,在其中作为参数传递了我们想渲染给客户端的视图名。清单12-4显示了名为MyView.cshtml并位于项目的Views/Derived文件夹的这个视图。
Listing 12-4. The MyView.cshtml File
@{ ViewBag.Title = "MyView"; } <h2>MyView</h2> Message: @ViewBag.Message
If you start the application and navigate to /Derived/Index, the action method we have defined will be executed, and the view we named will be rendered, as shown in Figure 12-2.
如果你启动这个应用程序,并导航到/Derived/Index,我们已经定义的这个动作方法将被执行,我们所命名的视图将被渲染,如图12-2所示。
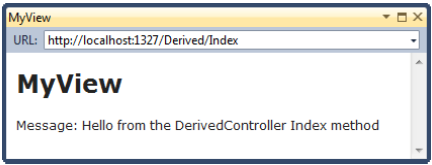
Figure 12-2. A result generated from the DerviedController class
Our job, as a derivation of the Controller class, is to implement action methods, obtain whatever input we need to process a request, and generate a suitable response. The myriad of ways that we can do this are covered in the rest of this chapter.
作为Controller类的一个派生,我们所要做的事情是实现动作方法,获取我们需要处理一个请求的任何输入,并生成一个适当的响应。本章的其余部分涉及我们能够做这种事情的无数办法。