java+selenium, python+selenium浏览界面,滑动页面
用到selenium的时候,有时需要滑动屏幕,模拟浏览,这时候就可以用到下边的代码了:
首先是java的selenium滑屏代码(不知道为啥后边就乱了排版,自己调整下就好~)
private static Random random = new Random();
private void pretendScrolling(JavascriptExecutor js, int sum) throws InterruptedException {
sum--;
int step;
int length;
switch (sum) {
case 0:
step = random.nextInt(30) + 1 + 50;
length = random.nextInt(3000) + 1 + 3000;
smoothlyScrolling(js, 0, step, length, true);
smoothlyScrolling(js, 0, step, length, false);
smoothlyScrolling(js, 0, step, length, true);
smoothlyScrolling(js, length, step, 8000, true);
step = random.nextInt(20) + 1 + 80;
smoothlyScrolling(js, 0, step, 8000, false);
length = random.nextInt(2000) + 1 + 3000;
smoothlyScrolling(js, 0, step, length, true);
smoothlyScrolling(js, 0, 20, length, false);
break;
case 1:
step = random.nextInt(10) + 1 + 40;
smoothlyScrolling(js, 0, step, 6000, true);
Thread.sleep(3000);
smoothlyScrolling(js, 0, step, 6000, false);
length = random.nextInt(1500) + 1 + 1000;
step = random.nextInt(10) + 1 + 40;
Thread.sleep(3000);
smoothlyScrolling(js, 0, step, length, true);
Thread.sleep(5000);
step = random.nextInt(10) + 1 + 40;
smoothlyScrolling(js, length, step, 7000, true);
Thread.sleep(3000);
step = random.nextInt(20) + 1 + 80;
smoothlyScrolling(js, 0, step, 8000, false);
Thread.sleep(3000);
break;
case 2:
step = random.nextInt(10) + 1 + 30;
length = random.nextInt(1300) + 1 + 1000;
smoothlyScrolling(js, 0, step, length, true); Thread.sleep(8000); step = random.nextInt(10) + 1 + 20; smoothlyScrolling(js, length, step, 6000, true); Thread.sleep(3000); length = random.nextInt(1500) + 1 + 1500; step = random.nextInt(20) + 1 + 40; smoothlyScrolling(js, length, step, 6000, false); Thread.sleep(3000); step = random.nextInt(30) + 1 + 70;
smoothlyScrolling(js, length, step, 6000, true);
Thread.sleep(4000);
step = random.nextInt(20) + 1 + 30;
smoothlyScrolling(js, 0, step, 6000, false);
Thread.sleep(3000);
break;
case 3:
step = random.nextInt(10) + 1 + 30;
smoothlyScrolling(js, 0, step, 7000, true);
Thread.sleep(3000);
step = random.nextInt(30) + 1 + 20;
smoothlyScrolling(js, 0, step, 7000, false);
Thread.sleep(3000);
break;
case 4:
step = random.nextInt(10) + 1 + 50;
smoothlyScrolling(js, 0, step, 7000, true);
Thread.sleep(3000);
length = random.nextInt(1500) + 1 + 2000;
step = random.nextInt(25) + 1 + 20;
smoothlyScrolling(js, length, step, 7000, false);
Thread.sleep(3000);
step = random.nextInt(20) + 1 + 30;
smoothlyScrolling(js, length, step, 7000, true);
Thread.sleep(3000);
step = random.nextInt(20) + 1 + 40;
smoothlyScrolling(js, 0, step, 7000, false);
Thread.sleep(2000);
break;
case 5:
step = random.nextInt(20) + 1 + 30;
smoothlyScrolling(js, 0, step, 2500, true);
Thread.sleep(3000);
step = random.nextInt(30) + 1 + 40;
smoothlyScrolling(js, 0, step, 2500, false);
Thread.sleep(3000);
step = random.nextInt(20) + 1 + 30;
smoothlyScrolling(js, 0, step, 7000, true);
Thread.sleep(6000);
step = random.nextInt(20) + 1 + 30;
smoothlyScrolling(js, 0, step, 7000, false);
Thread.sleep(2000);
break;
default:
case 6:
step = random.nextInt(30) + 1 + 30;
length = random.nextInt(1500) + 1 + 2500;
smoothlyScrolling(js, 0, step, length, true);
step = random.nextInt(30) + 1 + 70;
smoothlyScrolling(js, 0, step, length, false);
step = random.nextInt(20) + 1 + 40;
smoothlyScrolling(js, 0, step, 7000, true);
step = random.nextInt(25) + 1 + 20;
smoothlyScrolling(js, 0, step, 7000, false);
Thread.sleep(3000);
step = random.nextInt(25) + 1 + 20;
length = random.nextInt(1500) + 1 + 3000;
smoothlyScrolling(js, 0, step, length, true);
Thread.sleep(3000);
step = random.nextInt(15) + 1 + 30;
smoothlyScrolling(js, 0, step, length, false);
break;
}
}
private void smoothlyScrolling(JavascriptExecutor js, int floor, int step, int celling, boolean direction) {
if (direction) {
for (int i = floor; i <= celling; i += step) {
js.executeScript("scrollTo(0," + i + ")");
}
} else {
for (int i = celling; i >= floor; i -= step) {
js.executeScript("scrollTo(0," + i + ")");
}
}
}
用法如下:
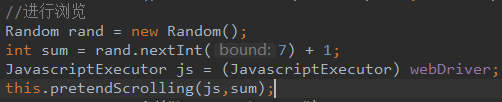
两个参数,其中js表示的是driver, sum是一个随机数,表示随机的从7中浏览方式之间选一种
再然后是在python selenium中的应用,我专门写了一个类出来存放浏览界面的代码,然后在需要用到它的地方导入,引用
import random
import time
class PretendScrolling:
@staticmethod
def pretend_scrolling(driver, sum):
step = 1
length = 1
if sum == 1:
step = random.randint(0, 30) + 1 + 50
length = random.randint(0, 3000) + 1 + 3000
smoothlyScrolling(driver, 0, step, length, True)
smoothlyScrolling(driver, 0, step, length, False)
smoothlyScrolling(driver, 0, step, length, True)
smoothlyScrolling(driver, length, step, 8000, False)
step = random.randint(0, 20)+ 1+80
smoothlyScrolling(driver, 0 ,step, 8000, False)
length = random.randint(0, 2000) + 1 + 3000
smoothlyScrolling(driver, 0 , step, length, True)
smoothlyScrolling(driver, 0 , 20, length, False)
elif sum == 2:
step = random.randint(0, 10) + 1 + 40
smoothlyScrolling(driver, 0, step, 6000, True)
time.sleep(3)
smoothlyScrolling(driver, 0, step, 6000, False)
length = random.randint(0, 1500) + 1 + 1000
step = random.randint(0, 10) + 1 + 40
time.sleep(3)
smoothlyScrolling(driver, 0, step, length, True)
time.sleep(5)
step = random.randint(0, 10)+1+40
smoothlyScrolling(driver, length, step, 7000, True)
time.sleep(3)
step = random.randint(0, 20)+1+80
smoothlyScrolling(driver, 0, step, 8000, False)
time.sleep(3)
elif sum == 3:
step = random.randint(0, 10) + 1 + 30
length = random.randint(0, 1300) + 1 + 1000
smoothlyScrolling(driver, 0, step, length, True)
time.sleep(8)
step = random.randint(0, 10) + 1 + 20
smoothlyScrolling(driver, length, step, 6000, True)
time.sleep(3)
length = random.randint(0, 1500) + 1 + 1500
step = random.randint(0, 20) + 1 + 40
smoothlyScrolling(driver, length, step, 6000, False)
time.sleep(3)
step = random.randint(0, 30) + 1 + 70
smoothlyScrolling(driver, length, step, 6000, True)
time.sleep(4)
step = random.randint(0, 20) + 1 + 30
smoothlyScrolling(driver, 0, step, 6000, False)
time.sleep(3)
elif sum == 4:
step = random.randint(0, 10) + 1 + 30
smoothlyScrolling(driver, 0, step, 7000, True)
time.sleep(3)
step = random.randint(0, 30) + 1 + 20
smoothlyScrolling(driver, 0, step, 7000, False)
time.sleep(3)
elif sum == 5:
step = random.randint(0, 10) + 1 + 50
smoothlyScrolling(driver, 0, step, 7000, True)
time.sleep(3)
length = random.randint(0, 1500) + 1 + 2000
step = random.randint(0, 25) + 1 + 20
smoothlyScrolling(driver, length, step, 7000, False)
time.sleep(3)
step = random.randint(0, 20) + 1 + 30
smoothlyScrolling(driver, length, step, 7000, True) time.sleep(3) step = random.randint(0, 20) + 1 + 40 smoothlyScrolling(driver, 0, step, 7000, False) time.sleep(2) elif sum == 6: step = random.randint(0, 20) + 1 + 30 smoothlyScrolling(driver, 0, step, 2500, True) time.sleep(3)
step = random.randint(0, 30) + 1 + 40
smoothlyScrolling(driver, 0, step, 2500, False)
time.sleep(3)
step = random.randint(0, 20) + 1 + 30
smoothlyScrolling(driver, 0, step, 7000, True)
time.sleep(6)
step = random.randint(0, 20) + 1 + 30
smoothlyScrolling(driver, 0, step, 7000, False)
time.sleep(2)
elif sum == 7:
step = random.randint(0, 30) + 1 + 30
length = random.randint(0, 1500) + 1 + 2500
smoothlyScrolling(driver, 0, step, length, True)
step = random.randint(0, 30) + 1 + 70
smoothlyScrolling(driver, 0, step, length, False)
step = random.randint(0, 20) + 1 + 40
smoothlyScrolling(driver, 0, step, 7000, True)
step = random.randint(0, 25) + 1 + 20
smoothlyScrolling(driver, 0, step, 7000, False)
time.sleep(3)
step = random.randint(0, 25) + 1 + 20
length = random.randint(0, 1500) + 1 + 3000
smoothlyScrolling(driver, 0, step, length, True)
time.sleep(3)
step = random.randint(0, 15) + 1 + 30
smoothlyScrolling(driver, 0, step, length, False)
else:
pass
#(不知道为啥会乱排版,这是另一个方法)def smoothlyScrolling(driver, floor, step, celling, direction):
if direction is True:
i = floor
while i <= celling:
i += step
jscript = "window.scrollTo(1, {height_i})".format(height_i=i)
driver.execute_script(jscript)
else:
i = celling
while i >= floor:
i -= step
jscript = "window.scrollTo(1, {height_i})".format(height_i=i)
driver.execute_script(jscript)
在python的其它类中引用如下:
首先先在相关类中引入滚动界面的类
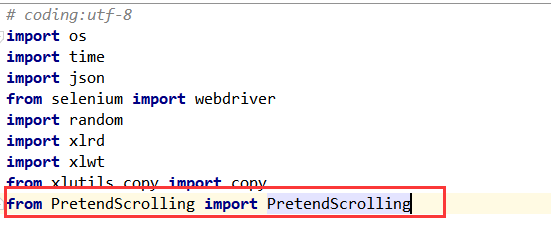
然后调用:
以上就是selenium里边滑动界面的一组方法了,支持java和python.
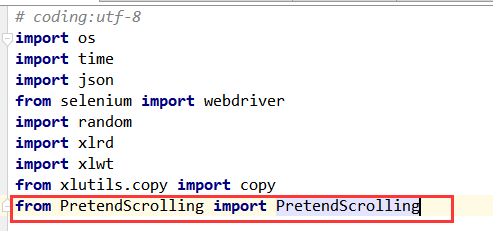

因为图片好像没有加载出来,所以再放一下.然后也不知道为啥排版会乱,要用的话自己调整一下即可.