RESTful Web服务的操作
1.首先我们说一下Http协议是无状态的
HTTP协议是无状态的,我们看到查到的用到的返回404,500,200,201,202,301.这些不是HTTP协议的状态码。
是HTTP的状态码,就是HTTP请求服务器返回的状态码。HTTP协议和HTTP请求返回状态码是二回事。
HTTP请求方法并不是只有GET和POST,只是最常用的。据RFC2616标准(现行的HTTP/1.1)得知,通常有以下8种方法:OPTIONS、GET、HEAD、POST、PUT、DELETE、TRACE和CONNECT。
HTTP请求方法并不是只有GET和POST,只是最常用的。据RFC2616标准(现行的HTTP/1.1)得知,通常有以下8种方法:OPTIONS、GET、HEAD、POST、PUT、DELETE、TRACE和CONNECT。
HTTP请求方法并不是只有GET和POST,只是最常用的。据RFC2616标准(现行的HTTP/1.1)得知,通常有以下8种方法:OPTIONS、GET、HEAD、POST、PUT、DELETE、TRACE和CONNECT。
重要的事情说三遍
2.现在比较流行的RSETful Web服务架构
关于REST及RESTful的概念,已有不少文章介绍,这里整理几篇我觉得不错的参考:
- 维基百科的定义: REST
- 什么是REST跟RESTful? REST理论的中文详述,其中你可以了解到WCF Restful属于RPC 样式的 Web 服务,ASP.NET Web API属于RESTful Web 服务。
- 深入浅出REST InfoQ的专文介绍,文中甚至有Roy T. Fielding当年REST博士论文的中文翻译链接。另外值得一提的,大家可能没听过Roy Fielding的大名,但如果得知他是HTTP规格的主要作者及Apache HTTP Server项目的发起人之一,应该不会有人怀疑他在Web技术领域的分量
REST是什么?
REST ( REpresentational State Transfer ),State Transfer 为 "状态传输" 或 "状态转移 ",Representational 中文有人翻译为"表征"、"具象",合起来就是 "表征状态传输" 或 "具象状态传输" 或 "表述性状态转移",不过,一般文章或技术文件都比较不会使用翻译后的中文来撰写,而是直接引用 REST 或 RESTful 来代表,因为 REST 一整个观念,想要只用六个中文字来完整表达真有难度。
REST 一词的出于《Architectural Styles and
the Design of Network-based Software Architectures》论文,我们先简单从标题来看,它应该是一种架构样式 (Architectural Styles) 与软件架构 (Software Architectures),而且是以网络 (Network-based) 为基础,重点就是:
- 架构样式 (Architectural Styles)
- 软件架构 (Software Architectures)
- 网络 (Network-based) 为基础
REST 本身是设计风格而不是标准。REST 谈论一件非常重要的事,如何正确地使用 Web标准,例如,HTTP 和 URI。想要了解 REST 最好的方式就是思索与了解 Web 及其工作方式。如果你设计的应用程序能符合 REST 原则 (REST principles),这些符合 REST 原则的 REST 服务可称为 "RESTful web service" 也称 "RESTful Web API"。"-ful" 字尾强调它们的设计完全符合 REST 论文里的建议内容。
3.那咱们长话短说,看怎么使用。
首先我们看一下HTTP状态码
我们常常使用的主要是GET,POST,PUT,DELETE正好和我们的CRUD(C:Create,R:Read,U:update,D:delete)对应
所以我们使用主要是如下列表
HTTP Method 与 CURD 数据处理操作对应 |
||
HTTP方法 |
数据处理 |
说明 |
POST |
Create |
新增一个没有id的资源 |
GET |
Read |
取得一个资源 |
PUT |
Update |
更新一个资源。或新增一个含 id 资源(如果 id 不存在) |
DELETE |
Delete |
删除一个资源 |
主要是如此:
package com.louis.lion.admin.controller; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.MalformedURLException; import java.net.URL; import java.net.URLEncoder; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.ResponseBody; import com.louis.lion.admin.service.SysUserService; @Controller public class RestfulController { @Autowired private SysUserService userService; // 修改 @RequestMapping(value = "put/{param}", method = RequestMethod.PUT) public @ResponseBody String put(@PathVariable String param) { return "put:" + param; } // 新增 @RequestMapping(value = "post/{param}", method = RequestMethod.POST) public @ResponseBody String post(@PathVariable String param,String id,String name) { System.out.println("id:"+id); System.out.println("name:"+name); return "post:" + param; } // 删除 @RequestMapping(value = "delete/{param}", method = RequestMethod.DELETE) public @ResponseBody String delete(@PathVariable String param) { return "delete:" + param; } // 查找 @RequestMapping(value = "get/{param}", method = RequestMethod.GET) public @ResponseBody String get(@PathVariable String param) { return "get:" + param; } // HttpURLConnection 方式调用Restful接口 // 调用接口 @RequestMapping(value = "dealCon/{param}") public @ResponseBody String dealCon(@PathVariable String param) { try { String url = "http://localhost:8080/tao-manager-web/"; url+=(param+"/xxx"); URL restServiceURL = new URL(url); HttpURLConnection httpConnection = (HttpURLConnection) restServiceURL .openConnection(); //param 输入小写,转换成 GET POST DELETE PUT httpConnection.setRequestMethod(param.toUpperCase()); // httpConnection.setRequestProperty("Accept", "application/json"); if("post".equals(param)){ //打开输出开关 httpConnection.setDoOutput(true); // httpConnection.setDoInput(true); //传递参数 String input = "&id="+ URLEncoder.encode("abc", "UTF-8"); input+="&name="+ URLEncoder.encode("啊啊啊", "UTF-8"); OutputStream outputStream = httpConnection.getOutputStream(); outputStream.write(input.getBytes()); outputStream.flush(); } if (httpConnection.getResponseCode() != 200) { throw new RuntimeException( "HTTP GET Request Failed with Error code : " + httpConnection.getResponseCode()); } BufferedReader responseBuffer = new BufferedReader( new InputStreamReader((httpConnection.getInputStream()))); String output; System.out.println("Output from Server: \n"); while ((output = responseBuffer.readLine()) != null) { System.out.println(output); } httpConnection.disconnect(); } catch (MalformedURLException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } return "success"; } }
然后大家可以看一下这篇文章,看看SpringMVC和RESTful的结合
前后端分离SpringMvc和RESTful理解
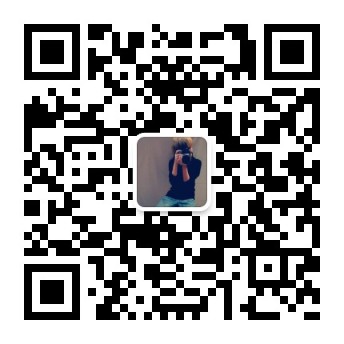