Java用于取得当前日期相对应的月初,月末,季初,季末,年初,年末时间
1 package com.zrar.date; 2 import java.util.Calendar; 3 /** 4 * 5 * 描述:此类用于取得当前日期相对应的月初,月末,季初,季末,年初,年末,返回值均为String字符串 6 * 1、得到当前日期 today() 7 * 2、得到当前月份月初 thisMonth() 8 * 3、得到当前月份月底 thisMonthEnd() 9 * 4、得到当前季度季初 thisSeason() 10 * 5、得到当前季度季末 thisSeasonEnd() 11 * 6、得到当前年份年初 thisYear() 12 * 7、得到当前年份年底 thisYearEnd() 13 * 8、判断输入年份是否为闰年 leapYear 14 * 15 * 注意事项: 日期格式为:xxxx-yy-zz (eg: 2007-12-05) 16 * 17 * 实例: 18 * 19 * @author pure 20 */ 21 public class DateThis { 22 private int x; // 日期属性:年 23 private int y; // 日期属性:月 24 private int z; // 日期属性:日 25 private Calendar localTime; // 当前日期 26 public DateThis() { 27 localTime = Calendar.getInstance(); 28 } 29 /** 30 * 功能:得到当前日期 格式为:xxxx-yy-zz (eg: 2007-12-05)<br> 31 * @return String 32 * @author pure 33 */ 34 public String today() { 35 String strY = null; 36 String strZ = null; 37 x = localTime.get(Calendar.YEAR); 38 y = localTime.get(Calendar.MONTH) + 1; 39 z = localTime.get(Calendar.DATE); 40 strY = y >= 10 ? String.valueOf(y) : ("0" + y); 41 strZ = z >= 10 ? String.valueOf(z) : ("0" + z); 42 return x + "-" + strY + "-" + strZ; 43 } 44 /** 45 * 功能:得到当前月份月初 格式为:xxxx-yy-zz (eg: 2007-12-01)<br> 46 * @return String 47 * @author pure 48 */ 49 public String thisMonth() { 50 String strY = null; 51 x = localTime.get(Calendar.YEAR); 52 y = localTime.get(Calendar.MONTH) + 1; 53 strY = y >= 10 ? String.valueOf(y) : ("0" + y); 54 return x + "-" + strY + "-01"; 55 } 56 /** 57 * 功能:得到当前月份月底 格式为:xxxx-yy-zz (eg: 2007-12-31)<br> 58 * @return String 59 * @author pure 60 */ 61 public String thisMonthEnd() { 62 String strY = null; 63 String strZ = null; 64 boolean leap = false; 65 x = localTime.get(Calendar.YEAR); 66 y = localTime.get(Calendar.MONTH) + 1; 67 if (y == 1 || y == 3 || y == 5 || y == 7 || y == 8 || y == 10 || y == 12) { 68 strZ = "31"; 69 } 70 if (y == 4 || y == 6 || y == 9 || y == 11) { 71 strZ = "30"; 72 } 73 if (y == 2) { 74 leap = leapYear(x); 75 if (leap) { 76 strZ = "29"; 77 } 78 else { 79 strZ = "28"; 80 } 81 } 82 strY = y >= 10 ? String.valueOf(y) : ("0" + y); 83 return x + "-" + strY + "-" + strZ; 84 } 85 /** 86 * 功能:得到当前季度季初 格式为:xxxx-yy-zz (eg: 2007-10-01)<br> 87 * @return String 88 * @author pure 89 */ 90 public String thisSeason() { 91 String dateString = ""; 92 x = localTime.get(Calendar.YEAR); 93 y = localTime.get(Calendar.MONTH) + 1; 94 if (y >= 1 && y <= 3) { 95 dateString = x + "-" + "01" + "-" + "01"; 96 } 97 if (y >= 4 && y <= 6) { 98 dateString = x + "-" + "04" + "-" + "01"; 99 } 100 if (y >= 7 && y <= 9) { 101 dateString = x + "-" + "07" + "-" + "01"; 102 } 103 if (y >= 10 && y <= 12) { 104 dateString = x + "-" + "10" + "-" + "01"; 105 } 106 return dateString; 107 } 108 /** 109 * 功能:得到当前季度季末 格式为:xxxx-yy-zz (eg: 2007-12-31)<br> 110 * @return String 111 * @author pure 112 */ 113 public String thisSeasonEnd() { 114 String dateString = ""; 115 x = localTime.get(Calendar.YEAR); 116 y = localTime.get(Calendar.MONTH) + 1; 117 if (y >= 1 && y <= 3) { 118 dateString = x + "-" + "03" + "-" + "31"; 119 } 120 if (y >= 4 && y <= 6) { 121 dateString = x + "-" + "06" + "-" + "30"; 122 } 123 if (y >= 7 && y <= 9) { 124 dateString = x + "-" + "09" + "-" + "30"; 125 } 126 if (y >= 10 && y <= 12) { 127 dateString = x + "-" + "12" + "-" + "31"; 128 } 129 return dateString; 130 } 131 /** 132 * 功能:得到当前年份年初 格式为:xxxx-yy-zz (eg: 2007-01-01)<br> 133 * @return String 134 * @author pure 135 */ 136 public String thisYear() { 137 x = localTime.get(Calendar.YEAR); 138 return x + "-01" + "-01"; 139 } 140 /** 141 * 功能:得到当前年份年底 格式为:xxxx-yy-zz (eg: 2007-12-31)<br> 142 * @return String 143 * @author pure 144 */ 145 public String thisYearEnd() { 146 x = localTime.get(Calendar.YEAR); 147 return x + "-12" + "-31"; 148 } 149 /** 150 * 功能:判断输入年份是否为闰年<br> 151 * 152 * @param year 153 * @return 是:true 否:false 154 * @author pure 155 */ 156 public boolean leapYear(int year) { 157 boolean leap; 158 if (year % 4 == 0) { 159 if (year % 100 == 0) { 160 if (year % 400 == 0) leap = true; 161 else leap = false; 162 } 163 else leap = true; 164 } 165 else leap = false; 166 return leap; 167 } 168 }
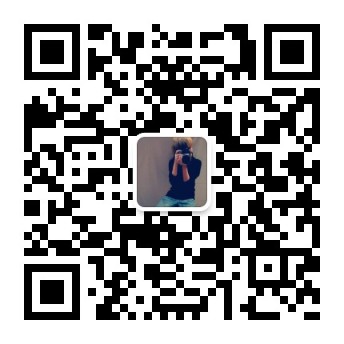