记录评论功能实体类
目前功能还在设计;
初步方案使用Springboot和Mongodb结合,完成一个jar的简单评论功能;
前期只设计接口方案。
Mongodb安装
后期会增加前端使用界面和方法
package com.comment.domain;
import java.io.Serializable;
import java.time.LocalDateTime;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
import lombok.Data;
/**
* 评价系统数据模型结构
*/
@Document
@Data
public class Comment implements Serializable {
private static final long serialVersionUID = 1L;
/**
* 评论内容主键id
*/
@Id
private Long _id;
/**
* 评论人昵称(如果获取不到可以使用javafaker来造一个)
*/
private String nickName;
/**
* 评论人ID(不允许为空)
*/
private Long userId;
/**
* 评论人Ip地址
*/
private String ipaddr;
/**
* 评论人IP所在地址
*/
private String ipLocation;
/**
* 评论人所使用的浏览器类型
*/
private String browser;
/**
* 评论人所使操作系统
*/
private String os;
/**
* 评论主体文章的ID(不允许为空)
*/
private Long subjectId;
/**
* 评论内容(不允许为空)
*/
private String content;
/**
* 是否是作者本人;默认不是
*/
private boolean flag = false;
/**
* 是否删除;默认不删除(允许为空)
*/
private boolean delete = false;
/**
* 父ID,如果默认是空就是没有人回复的帖子(允许为空)
*/
private Long parentId;
/**
* 发布日期
*/
private LocalDateTime publishDate;
/**
* 附件内容(可以为空)
*/
private String attachment;
}
使用的Dao方法
package com.comment.dao;
import java.util.List;
import org.springframework.data.mongodb.repository.MongoRepository;
import com.comment.domain.Comment;
public interface CommentDao extends MongoRepository<Comment, Long>{
/**
* @Title: findAllCommentBySubjectId
* @Description: 根据评论文章ID查询评论列表信息
* @Author 刘 仁
* @DateTime 2021-3-3 9:36:19
* @param subjectId
* @return
*/
public List<Comment> findAllCommentBySubjectId(Long subjectId);
}
Service方法
package com.comment.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.comment.dao.CommentDao;
import com.comment.domain.Comment;
import com.comment.util.SnowflakeIdWorker;
@Service
public class CommentService {
@Autowired
private CommentDao commentDao;
/**
* @Title: addComment
* @Description: 新增评论
* @Author 刘 仁
* @DateTime 2021-3-3 9:34:27
* @param comment
*/
public void addComment(Comment comment) {
SnowflakeIdWorker idWorker = new SnowflakeIdWorker(0, 0);
comment.set_id(idWorker.nextId());
commentDao.save(comment);
}
/**
* @Title: findAllCommentBySubjectId
* @Description: 根据文章ID查询评论列表信息
* @Author 刘 仁
* @DateTime 2021-3-3 9:38:15
* @param subjectId
* @return
*/
public List<Comment> findAllCommentBySubjectId(Long subjectId){
return commentDao.findAllCommentBySubjectId(subjectId);
}
}
controller方法
package com.comment.controller;
import java.time.LocalDateTime;
import java.util.List;
import java.util.Locale;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.comment.domain.Comment;
import com.comment.service.CommentService;
import com.github.javafaker.Faker;
@RestController
@RequestMapping("/comment")
public class CommentController {
@Autowired
private CommentService commentService;
@PostMapping("/add")
public void addComment() {
Faker fakerWithCN = new Faker(Locale.CHINA);
Comment comment = new Comment();
comment.setNickName(fakerWithCN.name().fullName());
comment.setContent("随机的评论内容");
comment.setUserId(1L);
comment.setIpaddr(fakerWithCN.internet().ipV4Address());
comment.setIpLocation(fakerWithCN.internet().ipV4Cidr());
comment.setBrowser(fakerWithCN.internet().userAgentAny());
comment.setOs(fakerWithCN.artist().name());
comment.setSubjectId(10001L);
comment.setParentId(null);
comment.setPublishDate(LocalDateTime.now());
commentService.addComment(comment);
}
@PostMapping("/list")
public List<Comment> findAllCommentBySubjectId(){
return commentService.findAllCommentBySubjectId(10001L);
}
}
直接调用http://localhost:9666/comment/add方法,每调用一次就新增一条记录
调用http://localhost:9666/comment/list根据文章ID查询该文章的所有评论信息
[
{
"_id": 816615260071919616,
"nickName": "刘立辉",
"userId": 1,
"ipaddr": "127.0.0.1",
"ipLocation": "聊城",
"browser": "Windows os",
"os": "os",
"subjectId": 10001,
"content": "随机的评论内容",
"flag": false,
"delete": false,
"parentId": null,
"publishDate": "2021-03-03T10:17:29.978",
"attachment": null
},
{
"_id": 816615958461284352,
"nickName": "袁立果",
"userId": 1,
"ipaddr": "127.0.0.1",
"ipLocation": "南昌",
"browser": "Windows os",
"os": "os",
"subjectId": 10001,
"content": "随机的评论内容",
"flag": false,
"delete": false,
"parentId": null,
"publishDate": "2021-03-03T10:20:16.488",
"attachment": null
},
{
"_id": 816616016950853632,
"nickName": "程风华",
"userId": 1,
"ipaddr": "127.0.0.1",
"ipLocation": "马鞍山",
"browser": "Windows os",
"os": "os",
"subjectId": 10001,
"content": "随机的评论内容",
"flag": false,
"delete": false,
"parentId": null,
"publishDate": "2021-03-03T10:20:30.433",
"attachment": null
},
{
"_id": 816616021933686784,
"nickName": "汪耀杰",
"userId": 1,
"ipaddr": "127.0.0.1",
"ipLocation": "深圳",
"browser": "Windows os",
"os": "os",
"subjectId": 10001,
"content": "随机的评论内容",
"flag": false,
"delete": false,
"parentId": null,
"publishDate": "2021-03-03T10:20:31.621",
"attachment": null
},
{
"_id": 816616022713827328,
"nickName": "范鹏涛",
"userId": 1,
"ipaddr": "127.0.0.1",
"ipLocation": "开封",
"browser": "Windows os",
"os": "os",
"subjectId": 10001,
"content": "随机的评论内容",
"flag": false,
"delete": false,
"parentId": null,
"publishDate": "2021-03-03T10:20:31.807",
"attachment": null
},
{
"_id": 816616023389110272,
"nickName": "汪驰",
"userId": 1,
"ipaddr": "127.0.0.1",
"ipLocation": "济宁",
"browser": "Windows os",
"os": "os",
"subjectId": 10001,
"content": "随机的评论内容",
"flag": false,
"delete": false,
"parentId": null,
"publishDate": "2021-03-03T10:20:31.968",
"attachment": null
},
{
"_id": 816616024072781824,
"nickName": "李瑾瑜",
"userId": 1,
"ipaddr": "127.0.0.1",
"ipLocation": "云浮",
"browser": "Windows os",
"os": "os",
"subjectId": 10001,
"content": "随机的评论内容",
"flag": false,
"delete": false,
"parentId": null,
"publishDate": "2021-03-03T10:20:32.131",
"attachment": null
},
{
"_id": 816616024781619200,
"nickName": "武展鹏",
"userId": 1,
"ipaddr": "127.0.0.1",
"ipLocation": "无锡",
"browser": "Windows os",
"os": "os",
"subjectId": 10001,
"content": "随机的评论内容",
"flag": false,
"delete": false,
"parentId": null,
"publishDate": "2021-03-03T10:20:32.3",
"attachment": null
},
{
"_id": 816616025490456576,
"nickName": "徐鹏飞",
"userId": 1,
"ipaddr": "127.0.0.1",
"ipLocation": "中山",
"browser": "Windows os",
"os": "os",
"subjectId": 10001,
"content": "随机的评论内容",
"flag": false,
"delete": false,
"parentId": null,
"publishDate": "2021-03-03T10:20:32.469",
"attachment": null
}
]
Windows安装MongoDB.zip绿色版
参考地址:https://www.cnblogs.com/qiyecao/p/11658340.html
启动失败的话,需要先删除安装的服务,重新安装
服务的删除:
有些小伙伴通过 cmd->services.msc 找到 mongodb服务,但却无法删除
方法:通过cmd 进入安装的mongodb路径,进入bin目录,使用命令 mongod.exe --remove --serviceName "MongoDB"
根据项目制作成一个jar包信息
kelan-comment-1.0.0.jar 打包成功后只有8K大小
整体项目组信压缩包下载:https://files.cnblogs.com/files/lr393993507/xomment.zip
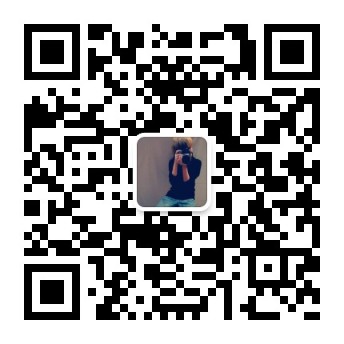