1. 最终效果:
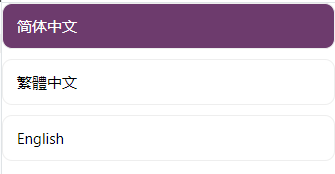
2. 实现代码:
import React, { useState } from 'react';
import {
FlatList,
SafeAreaView,
StatusBar,
StyleSheet,
Text,
TouchableOpacity,
} from 'react-native';
const DATA = [
{
id: 'zh_CN',
title: '简体中文',
},
{
id: 'zh_TW',
title: '繁體中文',
},
{
id: 'en_US',
title: 'English',
},
];
const Item = ({ item, onPress, backgroundColor, textColor }) => (
<TouchableOpacity
onPress={onPress}
style={[styles.item, { backgroundColor }]}>
<Text style={[styles.title, { color: textColor }]}>{item.title}</Text>
</TouchableOpacity>
);
const App = () => {
const [selectedId, setSelectedId] = useState();
const renderItem = ({ item }) => {
const backgroundColor = item.id === selectedId ? '#6e3b6e' : '#fff';
const color = item.id === selectedId ? 'white' : 'black';
return (
<Item
item={item}
onPress={() => setSelectedId(item.id)}
backgroundColor={backgroundColor}
textColor={color}
/>
);
};
return (
<SafeAreaView style={styles.container}>
<FlatList
data={DATA}
renderItem={renderItem}
keyExtractor={(item) => item.id}
extraData={selectedId}
/>
</SafeAreaView>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
marginTop: StatusBar.currentHeight || 0,
},
item: {
paddingVertical: 11,
paddingHorizontal: 14,
marginBottom: 10,
borderWidth: 1,
borderColor: '#EEEEEE',
borderRadius: 10,
},
title: {
fontSize: 15,
lineHeight: 22,
color: '#000',
},
});
export default App;
3. 参考文档:
React Native FlatList 文档