LeetCode in Python 236. Lowest Common Ancestor of a Binary Tree
https://leetcode.com/problems/lowest-common-ancestor-of-a-binary-tree/
Given a binary tree, find the lowest common ancestor (LCA) of two given nodes in the tree.
According to the definition of LCA on Wikipedia: “The lowest common ancestor is defined between two nodes p and q as the lowest node in T that has both p and q as descendants (where we allow a node to be a descendant of itself).”
Given the following binary tree: root = [3,5,1,6,2,0,8,null,null,7,4]
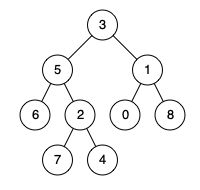
Example 1:
Input: root = [3,5,1,6,2,0,8,null,null,7,4], p = 5, q = 1 Output: 3 Explanation: The LCA of nodes5
and1
is3.
Example 2:
Input: root = [3,5,1,6,2,0,8,null,null,7,4], p = 5, q = 4 Output: 5 Explanation: The LCA of nodes5
and4
is5
, since a node can be a descendant of itself according to the LCA definition.
Note:
- All of the nodes' values will be unique.
- p and q are different and both values will exist in the binary tree.
Solution:
# Definition for a binary tree node. # class TreeNode(object): # def __init__(self, x): # self.val = x # self.left = None # self.right = None class Solution(object): def lowestCommonAncestor(self, root, p, q): """ :type root: TreeNode :type p: TreeNode :type q: TreeNode :rtype: TreeNode """ def isAncestor(m, n): if not m: return False if m == n or m.left == n or m.right == n: return True return isAncestor(m.left, n) or isAncestor(m.right, n) if isAncestor(p, q): return p elif isAncestor(q, p): return q elif (isAncestor(root.left, p) and isAncestor(root.right, q)) or (isAncestor(root.right, p) and isAncestor(root.left, q)): return root elif isAncestor(root.left, p) and isAncestor(root.left, q): return self.lowestCommonAncestor(root.left, p, q) else: return self.lowestCommonAncestor(root.right, p, q)
三种情况:1.p,q有亲缘关系,返回父节点 2.p,q都在同一个子树,递归调用 3.p,q分别在左右子树,LCA是root