常见内置模块3和购物车
hashlib加密模块
加密是什么
将明文数据通过一些媒介变成密文数据;密文数据的表现形式一般都是一串没有规则的字符串。
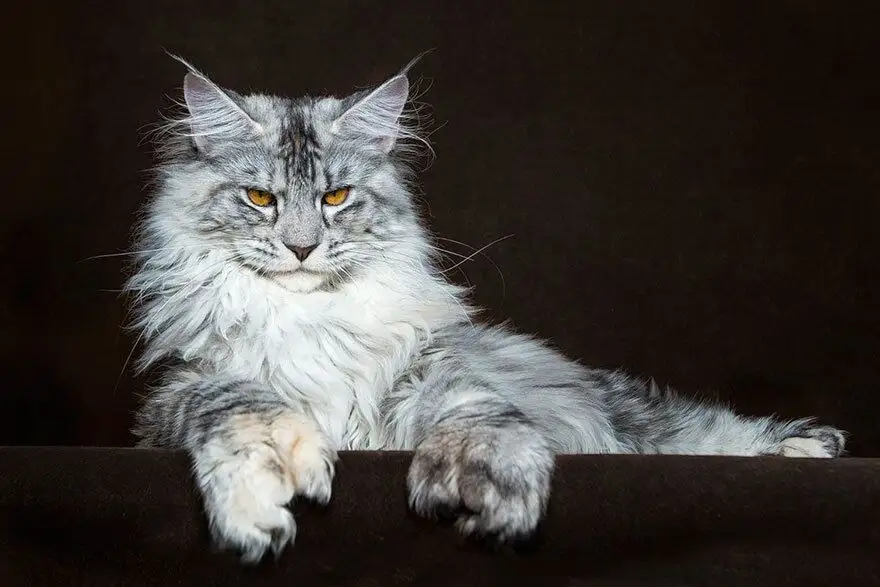
加密算法
加密算法是将明文变密文的内部规则,种类繁多。
算法的难易程度一般根据产生密文的字符个数判断,相同的明文加密后字符串越长算法越复杂。
加密使用场景
涉及到隐私数据的时候,最为常见的就是对用户的密码加密,保障密码安全
基本使用
import hashlib
md5 = hashlib.md5()
md5.update('trump'.encode('utf8'))
print(md5.hexdigest()) # 6ad5d29de368db3dcf6f9d8e133a223a
md5 = hashlib.md5()
md5.update('maga'.encode('utf8'))
print(md5.hexdigest()) # ca2f848f64fecd2159026ef8c0d71363
# 在传入数据的时候,只要名文(被加密对象)不变,结果就不变
md5 = hashlib.md5()
md5.update(b'trump')
md5.update(b'maga')
print(md5.hexdigest()) # 29ef6a5f3edb411865e4df5999480ffe
md5 = hashlib.md5()
md5.update(b'trumpmaga')
print(md5.hexdigest()) # 29ef6a5f3edb411865e4df5999480ffe
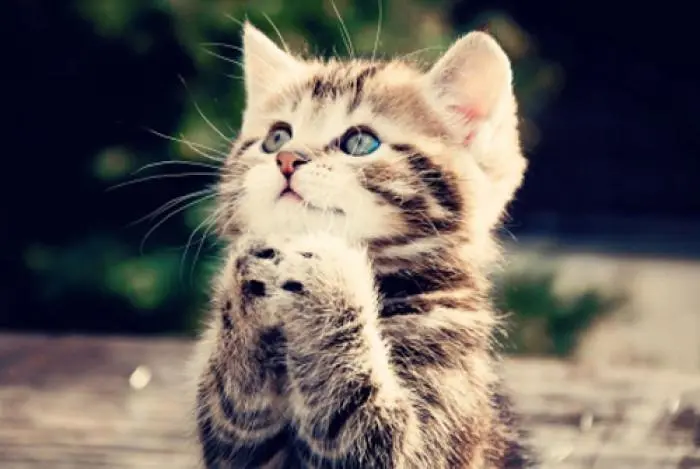
补充
# 1.加密之后的结果是无法直接反解密的
import hashlib
md5 = hashlib.md5()
md5.update(b'abc')
print(md5.hexdigest()) # 900150983cd24fb0d6963f7d28e17f72
md5 = hashlib.md5()
md5.update(b'111')
print(md5.hexdigest()) # 698d51a19d8a121ce581499d7b701668
md5 = hashlib.md5()
md5.update(b'222')
print(md5.hexdigest()) # bcbe3365e6ac95ea2c0343a2395834dd
# 所谓的反解密其实是暴力破解
"""
md5解密内部本质
提前用常见明文加密,保存明文-密文组合,拿到一个密文就去找对应的明文
abc 加密保存900150983cd24fb0d6963f7d28e17f72
111 加密保存698d51a19d8a121ce581499d7b701668
222 加密保存bcbe3365e6ac95ea2c0343a2395834dd
明文-密文组合{'密文1':abc,'密文2':111,'密文2':222}
破解是就在组合中一一寻找
"""
# 2.加盐处理
# 目的是增加破解的难度
md5 = hashlib.md5()
# 加盐处理(添加干扰项)
md5.update('机密文件'.encode('utf8'))
md5.update(b'abc')
print(md5.hexdigest()) # d6a5375cecc24d1ad16bd1b930be3e91
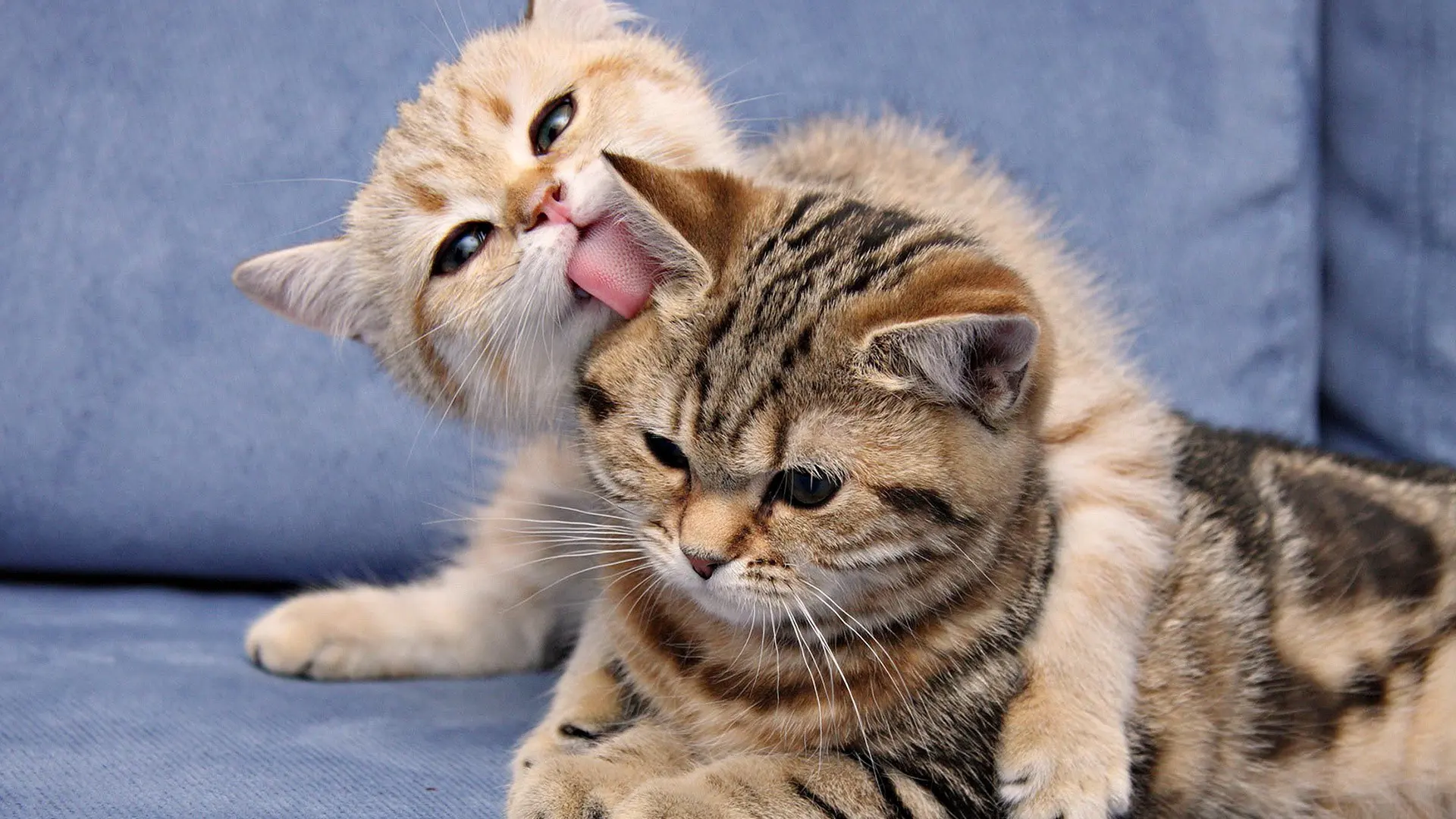
logging模块
日志模块就是在程序的各个环境记录
日志等级
日志按照重要程度分为五个级别:默认只有达到warning警告级别及以上才会记录日志保存
import logging
logging.debug('debug message')
logging.info('info message')
logging.warning('warning message')
logging.error('error message')
logging.critical('critical message')
基本使用
import logging
file_handler = logging.FileHandler(filename='x1.log', mode='a', encoding='utf-8', )
logging.basicConfig(
format='%(asctime)s - %(name)s - %(levelname)s -%(module)s: %(message)s',
datefmt='%Y-%m-%d %H:%M:%S %p',
handlers=[file_handler, ],
level=logging.ERROR
)
logging.error('warnings!!!!!')
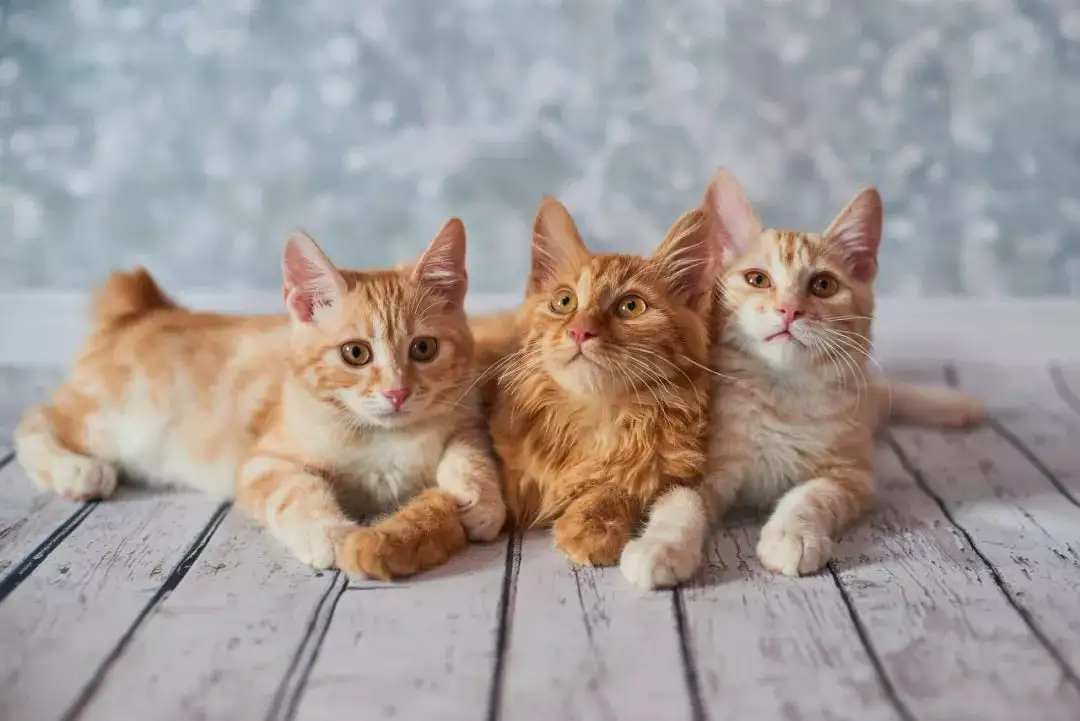
购物车练习
import os
import json
import hashlib
current_path = os.path.dirname(__file__)
db_path = os.path.join(current_path, 'db')
if os.path.isdir(db_path):
pass
else:
os.mkdir(db_path)
goods_list = [
['钢笔', 50],
['漫画书', 5],
['小冰箱', 888],
['空调', 1999],
['沙发', 500],
['电脑', 8000],
['限量手办', 50000]
]
paid_list = []
is_login = {'username': None}
from functools import wraps
def login_auth(func):
@wraps(func)
def inner(*args, **kwargs):
if is_login.get('username'):
res = func(*args, **kwargs)
return res
else:
login_auth1()
if is_login.get('username'):
res = func(*args, **kwargs)
return res
return inner
def register():
new_username = input('请输入新的用户名>>>:').strip()
new_pwd = input('请输入密码>>>:').strip()
confirm_pwd = input('确认密码>>>:').strip()
full_path = os.path.join(db_path, f'{new_username}.json')
print(full_path)
if new_pwd == confirm_pwd:
md5 = hashlib.md5()
md5.update(new_username.encode('utf8'))
new_username1 = md5.hexdigest() # 用变量名new_username1接收密文
md5 = hashlib.md5()
md5.update(new_pwd.encode('utf8'))
new_pwd1 = md5.hexdigest() # # 用变量名new_pwd1接收密文
temp = {'name': new_username1, 'pwd': new_pwd1, 'money': 20000, 'shop_car': {}}
if not os.path.isfile(full_path):
with open(full_path, 'w', encoding='utf8') as f:
json.dump(temp, f, ensure_ascii=False)
print(f'{new_username}注册成功')
return
else:
print('密码不一致')
def login_auth1():
username = input('请输入您的用户名>>>:').strip()
pwd = input('请输入密码>>>:').strip()
temp_path = os.path.join(db_path, f'{username}.json')
md5 = hashlib.md5()
md5.update(username.encode('utf8'))
username1 = md5.hexdigest() # 用变量名username1接收密文
md5 = hashlib.md5()
md5.update(pwd.encode('utf8'))
pwd1 = md5.hexdigest() # 用变量名pwd1接收密文
if os.path.isfile(temp_path):
with open(temp_path, 'r', encoding='utf8') as f1:
temp_user_dict = json.load(f1)
if pwd1 == temp_user_dict['pwd'] and username1 == temp_user_dict['name']:
print('登陆成功')
is_login['username'] = username
else:
print('密码错误')
else:
print('用户不存在')
@login_auth
def add_shop_car():
flag = True
while flag:
for i, j in enumerate(goods_list, start=1):
print("""
商品编号:%s | 商品名称:%s | 商品价格:%s
""" % (i, j[0], j[1]))
choice = input('请输入商品编号>>>,输入n退出:').strip()
if choice == 'n':
break
purchase_num = input('请输入购买数量>>>:').strip()
if not choice.isdigit():
print('编号为纯数字')
break
if not int(choice) < len(goods_list) + 1:
print('输入有误')
break
choice = int(choice) - 1
if not purchase_num.isdigit():
print('数字!!!')
purchase_num = int(purchase_num)
if not int(purchase_num) > 0:
print('必须大于0')
print(f"""
您的购物车:
商品名称{goods_list[choice][0]}
数量{purchase_num}
价格{goods_list[choice][1]}
""")
temp_shop_car = {goods_list[choice][0]: [purchase_num, goods_list[choice][1]]}
file_path = os.path.join(db_path, f"{is_login['username']}.json")
with open(file_path, 'r', encoding='utf8') as f1:
real_shop_car = json.load(f1)
for goods_name in temp_shop_car:
if goods_name in real_shop_car['shop_car']:
real_shop_car['shop_car'][goods_list[choice][0]][0] += int(temp_shop_car[goods_list[choice][0]][0])
break
else:
real_shop_car['shop_car'][goods_list[choice][0]] = temp_shop_car[goods_list[choice][0]]
with open(file_path, 'w', encoding='utf8') as f2:
json.dump(real_shop_car, f2, ensure_ascii=False)
print('添加成功')
return
@login_auth
def paid_money():
tag1 = True
while tag1:
file_path = os.path.join(db_path, f"{is_login['username']}.json")
with open(file_path, 'r', encoding='utf8') as f:
user_dict = json.load(f)
shop_car = user_dict['shop_car']
sum_price = 0
for goods_num, goods_price in shop_car.values():
sum_price += goods_num * goods_price
for item in user_dict['shop_car'].items():
print("""
商品名称:%s
购买数量:%s
商品单价:%s
""" % (item[0], item[1][0], item[1][1]))
print(f"本次付款金额为{sum_price}元,您的账户目前有{user_dict['money']}元")
if user_dict['money'] < sum_price:
print('余额不足')
tag1 = False
if user_dict['money'] >= sum_price:
cmd = input('余额足够,输入y确认购买>>>:').strip()
if cmd == 'y':
rest_money = user_dict['money'] - sum_price
print(f"付款金额为{sum_price}元,消费后余额为{rest_money}元")
user_dict['money'] = rest_money
user_dict['shop_car'] = {}
with open(file_path, 'w', encoding='utf8') as f1:
json.dump(user_dict, f1, ensure_ascii=False)
tag1 = False
break
def exit():
global tag
tag = False
func_dict = {'1': register,
'2': login_auth1,
'3': add_shop_car,
'4': paid_money,
'5': exit
}
tag = True
def start():
while tag:
print("""
1.用户注册
2.用户登录
3.添加购物车
4.结算购物车
5.退出
""")
choice = input('请选择功能编号>>>:').strip()
if choice.isdigit() and choice in func_dict:
func_dict.get(choice)()
else:
print('输入有误')
start()
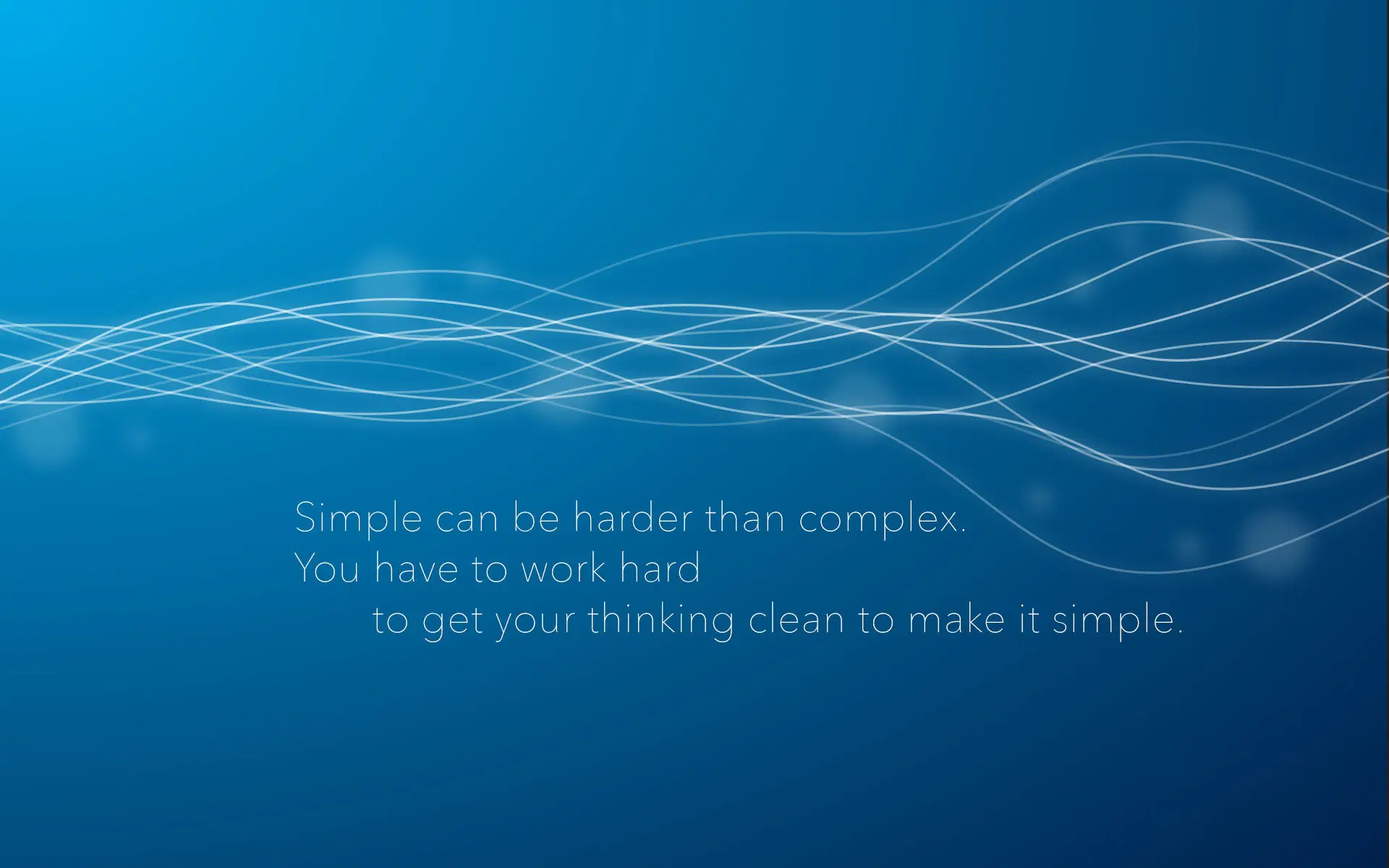