re模块补充和常用内置模块
目录
re模块补充
findall与分组
findall默认是分组优先展示。正则表达式中如果有括号分组,默认只展示括号内正则表达式匹配到的内容
也可以用(?:)取消分组优先展示的机制
import re
res = re.findall('n(o) ', "The wise man builds no hopes for the future, entertains no regrets for the past")
print(res) # ['o', 'o']
res = re.findall('n(?:o) ', "The wise man builds no hopes for the future, entertains no regrets for the past")
print(res) # ['no ', 'no ']
res = re.findall('(n)(o)( )', "The wise man builds no hopes for the future, entertains no regrets for the past")
print(res) # [('n', 'o', ' '), ('n', 'o', ' ')]
res = re.findall('(?P<name1>n)(o)( )', "The wise man builds no hopes for the future, entertains no regrets for the past")
print(res) # [('n', 'o', ' '), ('n', 'o', ' ')] 起别名没有意义,不支持group调用
# print(res.group('name1')) # 'list' object has no attribute 'group'
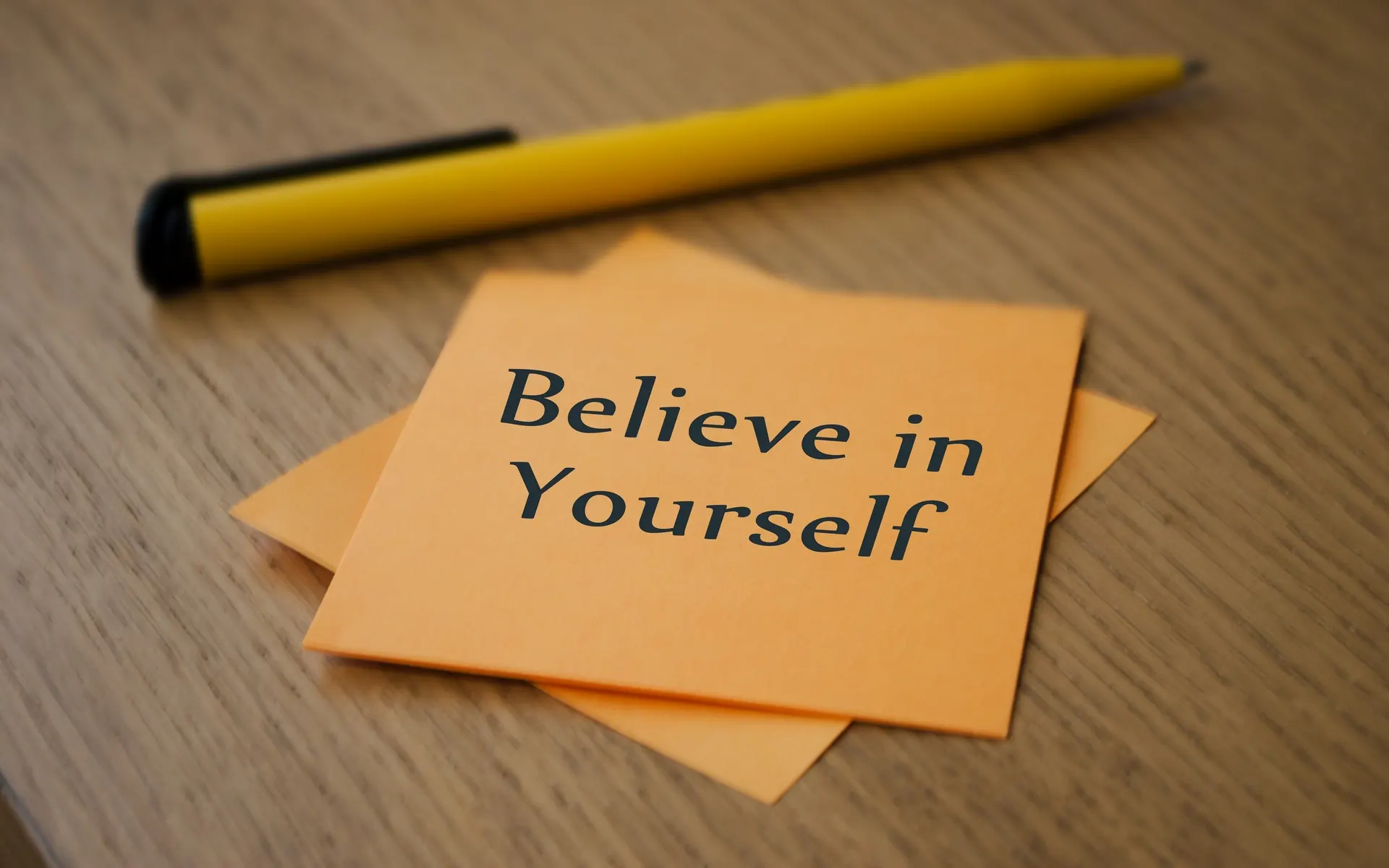
search与分组
# 针对search有多少个分组,group方法括号内参数最大就是多少
res = re.search('n(o) ', "The wise man builds no hopes for the future, entertains no regrets for the past")
print(res.group()) # no
print(res.group(0)) # no
print(res.group(1)) # o
res = re.search('n(o)( )', "The wise man builds no hopes for the future, entertains no regrets for the past")
print(res.group()) # no
print(res.group(0)) # no
print(res.group(1)) # o
print(res.group(2)) # 空格
# 分组之后还可以给组起别名
res = re.search('(?P<name1>n)(?P<name2>o)( )', "The wise man builds no hopes for the future, entertains no regrets for the past")
print(res.group('name1')) # n
print(res.group('name2')) # o
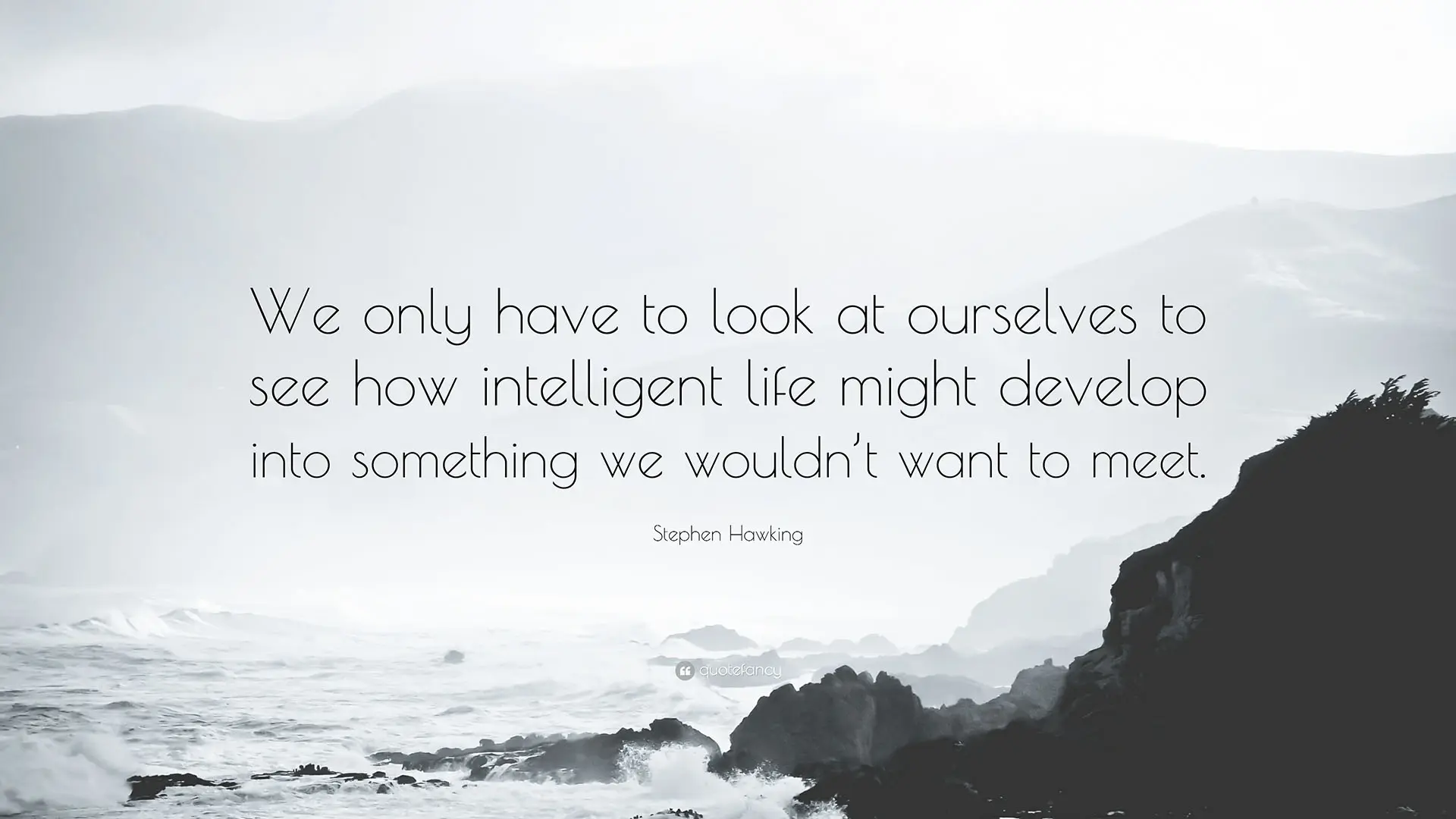
match与分组
# 针对match有多少个分组,group方法括号内参数最大就是多少
res = re.match('(T)he', "The wise man builds no hopes for the future, entertains no regrets for the past")
print(res.group()) # The
print(res.group(0)) # The
print(res.group(1)) # T
res = re.match('(T)(h)e', "The wise man builds no hopes for the future, entertains no regrets for the past")
print(res.group()) # The
print(res.group(0)) # The
print(res.group(1)) # T
print(res.group(2)) # h
# 分组之后还可以给组起别名
res = re.match('(?P<name1>T)(?P<name2>h)(e)', "The wise man builds no hopes for the future, entertains no regrets for the past")
print(res.group('name1')) # T
print(res.group('name2')) # h
常用内置模块
collections模块
具名元组
from collections import namedtuple
# 第一步、先产生一个元组对象模板
point = namedtuple('商品', ['product_name', 'product_price'])
# 第二步、创建诸多元组数据
product_info = point('pen', '$50')
product_info1 = point('book', '$20')
product_info2 = point('desk', '$100')
print(product_info, product_info1, product_info2) # 商品(product_name='pen', product_price='$50') 商品(product_name='book', product_price='$20') 商品(product_name='desk', product_price='$100')
print(product_info.product_name) # pen
print(product_info.product_price) # $50
print(product_info2.product_name) # desk
print(product_info2.product_price) # $100
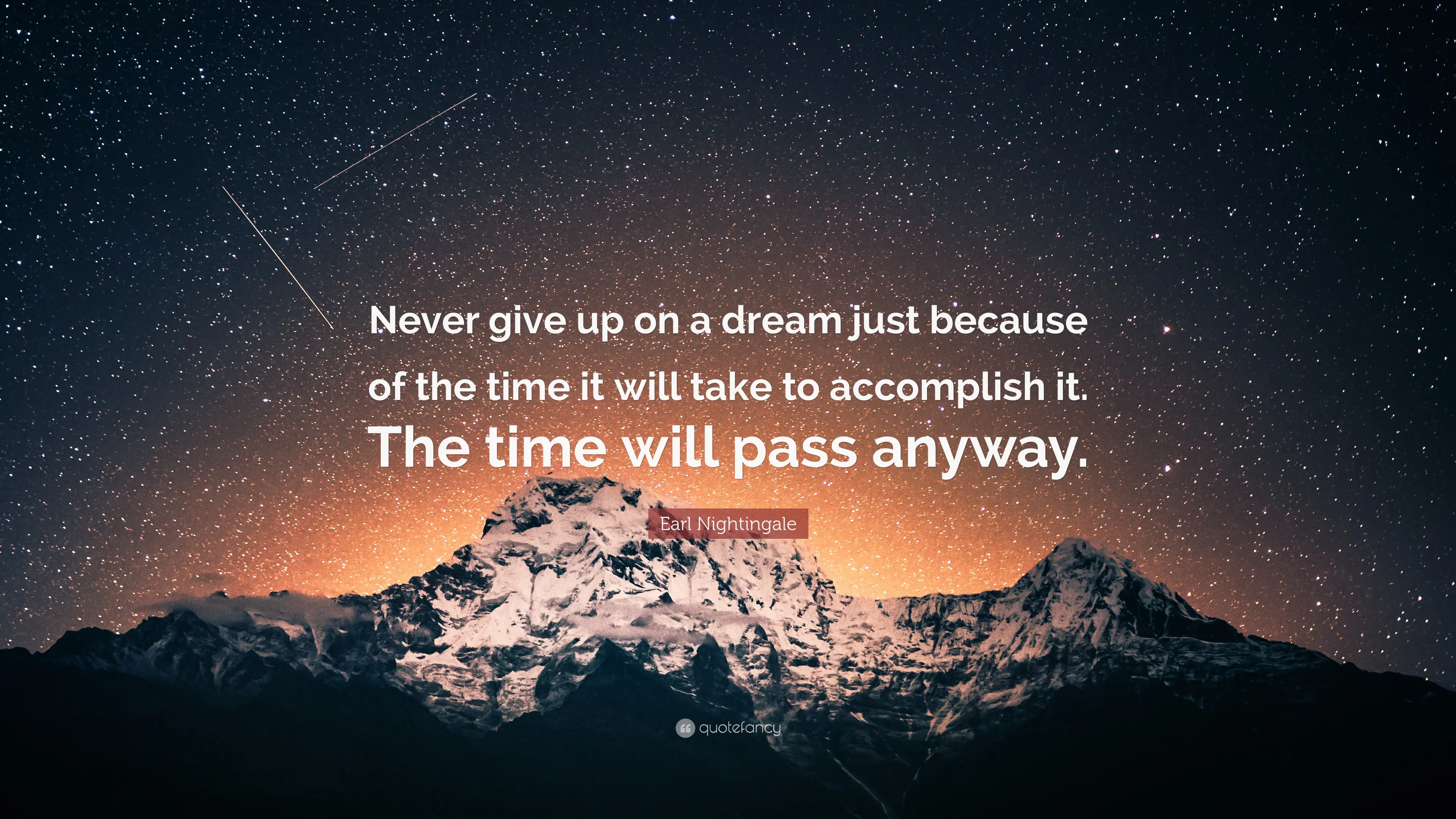
双端队列
# 普通队列
import queue
name_info = queue.Queue(5) # 最大只能放五个元素
# 存放元素
name_info.put('jack')
name_info.put('tom')
name_info.put('annie')
name_info.put('sam')
name_info.put('mary')
# name_info.put('jerry') # 队列以满,原地等待
# 获取元素
print(name_info.get()) # jack
print(name_info.get()) # tom
print(name_info.get()) # annie
print(name_info.get()) # sam
print(name_info.get()) # mary
# print(name_info.get()) # 队列以空,原地等待
# 双端队列
from collections import deque
name_info = deque(['jack', 'tom', 'annie'])
print(name_info) # deque(['jack', 'tom', 'annie'])
name_info.append('sam') # 右边添加元素
print(name_info) # deque(['jack', 'tom', 'annie', 'sam'])
name_info.appendleft('mary') # 左边添加元素
print(name_info) # deque(['mary', 'jack', 'tom', 'annie', 'sam'])
name_info.pop() # 右边弹出元素
name_info.popleft() # 左边弹出元素
print(name_info) # deque(['jack', 'tom', 'annie'])
字典相关
# 正常的字典内部是无序的
d1 = dict([('name', 'sam'), ('age', 20), ('hobby', 'play games'), ('height', '175cm')])
for i in d1:
print(i) # name age hobby height
print(d1) # {'name': 'sam', 'age': 20, 'hobby': 'play games', 'height': '175cm'}
print(d1.keys()) # dict_keys(['name', 'age', 'hobby', 'height'])
# 有序字典
from collections import OrderedDict
d2 = OrderedDict([('a', 97), ('b', 98), ('c', 99)])
print(d2) # OrderedDict([('a', 97), ('b', 98), ('c', 99)])
print(chr(102)) # f
d2['d'] = 100
d2['e'] = 101
d2['f'] = 102
print(d2) # OrderedDict([('a', 97), ('b', 98), ('c', 99), ('d', 100), ('e', 101), ('f', 102)])
print(d2.keys()) # odict_keys(['a', 'b', 'c', 'd', 'e', 'f'])
将列表中[11, 22, 33, 44, 55, 66, 77, 88, 99, 111, 222, 333, 444, 555, 666]大于111的值保存到字典的第一个key中,将不大于111的值保存至第二个key的值中。
l1 = [11, 22, 33, 44, 55, 66, 77, 88, 99, 111, 222, 333, 444, 555, 666]
dict1 = {'k1': [], 'k2': []}
for i in l1:
if i > 111:
dict1['k1'].append(i)
else:
dict1['k2'].append(i)
print(dict1) # {'k1': [222, 333, 444, 555, 666], 'k2': [11, 22, 33, 44, 55, 66, 77, 88, 99, 111]}
from collections import defaultdict
l2 = [11, 22, 33, 44, 55, 66, 77, 88, 99, 111, 222, 333, 444, 555, 666]
dict2 = defaultdict(list)
for value in l2:
if value > 111:
dict2['k1'].append(value)
else:
dict2['k2'].append(value)
print(dict2) # defaultdict(<class 'list'>, {'k2': [11, 22, 33, 44, 55, 66, 77, 88, 99, 111], 'k1': [222, 333, 444, 555, 666]})
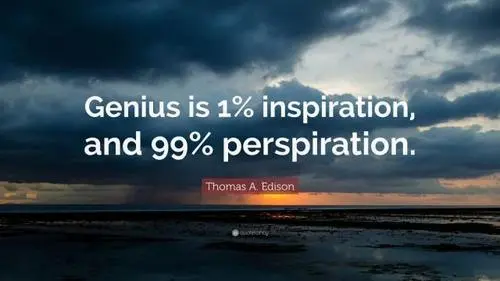
计数器
string = 'aasdhjbhsBHjababbcbdabbbahabbcajjcbahdhbchccajjcaajcahchahccaasbb'
# 统计字符串中所有字符出现的次数
dict1 = {}
for i in string:
if i not in dict1:
dict1[i] = 1
else:
dict1[i] += 1
print(dict1) # {'a': 16, 's': 3, 'd': 3, 'h': 9, 'j': 7, 'b': 14, 'B': 1, 'H': 1, 'c': 11}
from collections import Counter
res = Counter(string)
print(res) # Counter({'a': 16, 'b': 14, 'c': 11, 'h': 9, 'j': 7, 's': 3, 'd': 3, 'B': 1, 'H': 1})
print(res.get('a')) # 16
print(res.get('b')) # 14
print(res.get('j')) # 7
time模块
常用方法
import time
time.sleep(1) # 推迟指定的时间运行,单位为秒
print(time.time()) # 获取当前时间戳
三种用于表示时间的格式
- 时间戳
距离1970年1月1日0时0分0秒至此相差的秒数 - 结构化时间
该时间类型主要方便计算机识别
import time
print(time.localtime()) # time.struct_time(tm_year=2022, tm_mon=3, tm_mday=29, tm_hour=20, tm_min=58, tm_sec=17, tm_wday=1, tm_yday=88, tm_isdst=0)
索引 | 元组元素名称 | 意义 | 举例 |
---|---|---|---|
0 | tm_year | 年 | 2022 |
1 | tm_mon | 月 | 3 |
2 | tm_mday | 日 | 29 |
3 | tm_hour | 时 | 20 |
4 | tm_min | 分 | 58 |
5 | tm_sec | 秒 | 50 |
6 | tm_wday | 周几 | 1 |
7 | tm_yday | 一年中的第几天 | 88 |
8 | tm_isdst | 是否夏令时 | 0 |
- 格式化时间
人最容易接收的一种时间格
import time
print(time.strftime('%Y-%m-%d %H:%M:%S')) # 2022-03-29 21:15:38
print(time.strftime('%Y-%m-%d %X')) # 2022-03-29 21:15:38
特殊符号 | 意义 |
---|---|
%y | 两位数的年份表示(00-99) |
%Y | 四位数的年份表示(000-9999) |
%m | 月份(01-12) |
%d | 月内中的一天(0-31) |
%H | 24小时制小时数(0-23) |
%I | 12小时制小时数(01-12) |
%M | 分钟数(00=59) |
%S | 秒(00-59) |
%a | 本地简化星期名称 |
%A | 本地完整星期名称 |
%b | 本地简化的月份名称 |
%B | 本地完整的月份名称 |
%c | 本地相应的日期表示和时间表示 |
%j | 年内的一天(001-366) |
%p | 本地A.M.或P.M.的等价符 |
%U | 一年中的星期数(00-53)星期天为星期的开始 |
%w | 星期(0-6),星期天为星期的开始 |
%W | 一年中的星期数(00-53)星期一为星期的开始 |
%x | 本地相应的日期表示 |
%X | 本地相应的时间表示 |
%Z | 当前时区的名称 |
%% | %号本身 |
时间类型的转换
格式化时间<--> 结构化时间 <--> 时间戳
import time
# 格式化时间-->结构化时间
print(time.strptime("2017-03-16", "%Y-%m-%d"))
print(time.strptime("2017/03", "%Y/%m")) # 两个参数需要对应
# 结构化时间-->格式化时间
print(time.strftime("%Y-%m-%d %X")) # 2022-03-29 21:40:22
print(time.strftime("%Y-%m-%d", time.localtime(1000000000))) # 2001-09-09
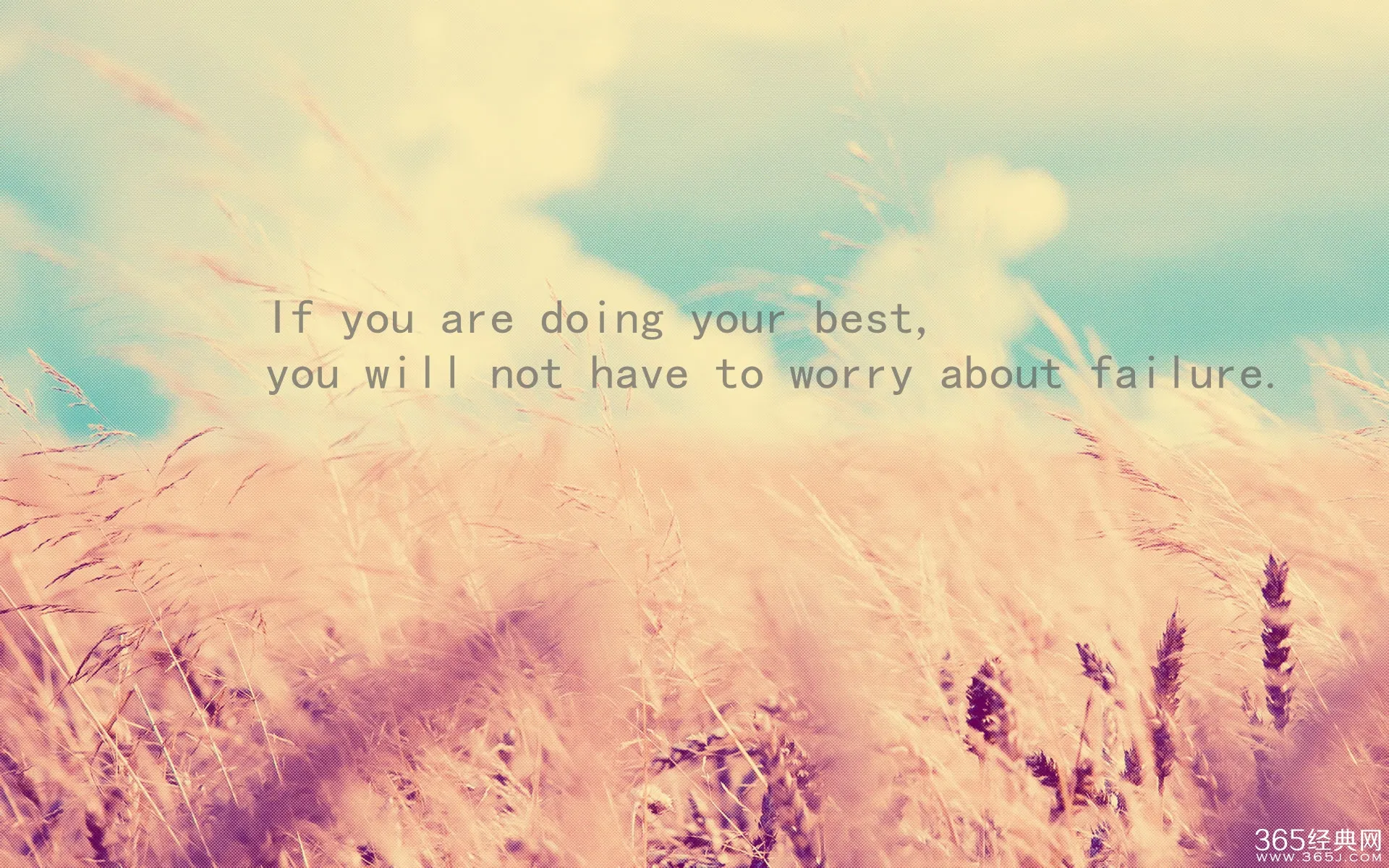
datetime模块
# 基本操作
import datetime
print(datetime.date.today()) # 2022-03-29
print(datetime.datetime.today()) # 2022-03-29 21:43:37.659159
"""
date 意思就是年月日
datetime 意思就是年月日时分秒
"""
res = datetime.date.today()
print(res.year) # 2022
print(res.month) # 3
print(res.day) # 29
print(res.weekday()) # 1
print(res.isoweekday()) # 2
# 时间差
ctime = datetime.datetime.today()
time_tel = datetime.timedelta(days=5)
print(ctime) # 2022-03-29 21:45:12.608221
print(ctime + time_tel) # 2022-04-03 21:45:12.608221
print(ctime - time_tel) # 2022-03-24 21:45:12.608221
"""
时间计算的公式
日期对象 = 日期对象 +/- timedelta对象
timedelta对象 = 日期对象 +/- 日期对象
"""
res = ctime + time_tel
print(res - ctime) # 5 days, 0:00:00
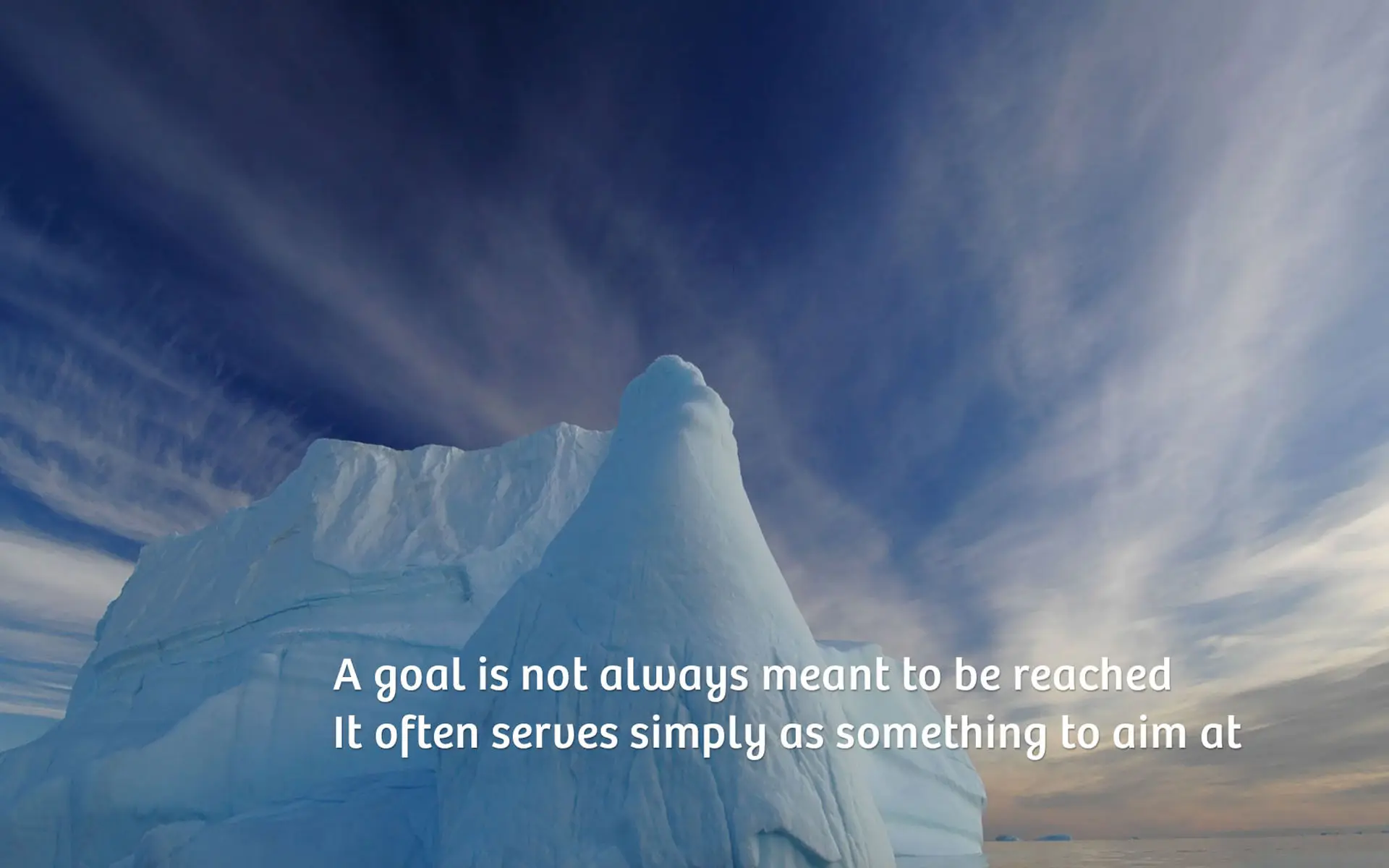
random模块
import random
# 基本使用
print(random.random()) # 随机产生一个0到1之间的小数
print(random.uniform(7, 10)) # 随机产生一个7到10之间的小数
print(random.randint(0, 9)) # 随机产生一个0到9之间的整数(包含0和9)
# 实际应用,随机生成验证码
verification_code_length = input('生成验证码的个数>>>:').strip()
code1 = []
for i in range(97, 122):
code1.append(chr(i))
for j in range(65, 90):
code1.append(chr(j))
for k in range(0, 9):
code1.append(str(k))
code2 = random.sample(code1, int(verification_code_length))
code3 = "".join(code2)
print('验证码为:', code3)
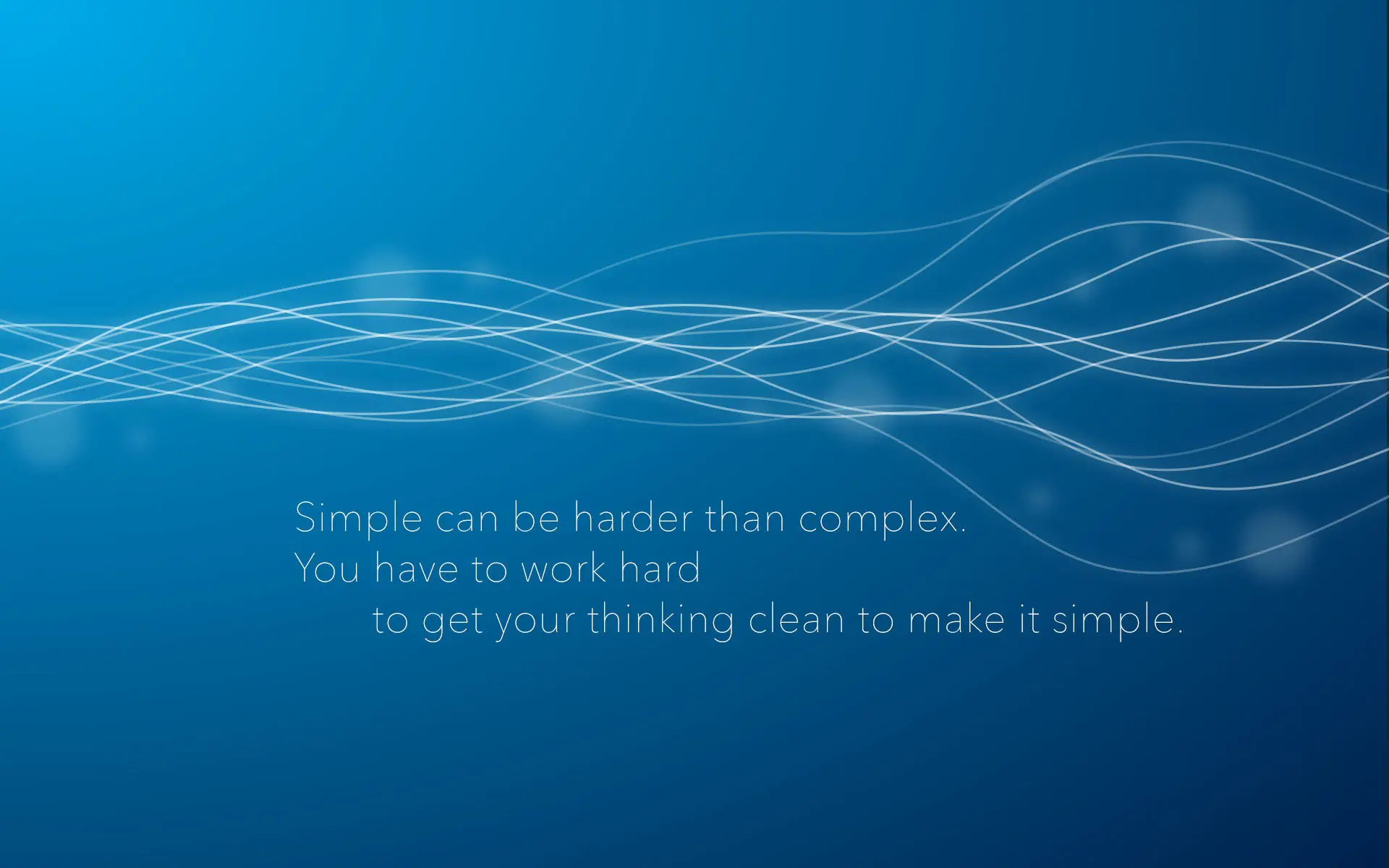