函数补充和常见的内置函数
目录
函数
三元表达式
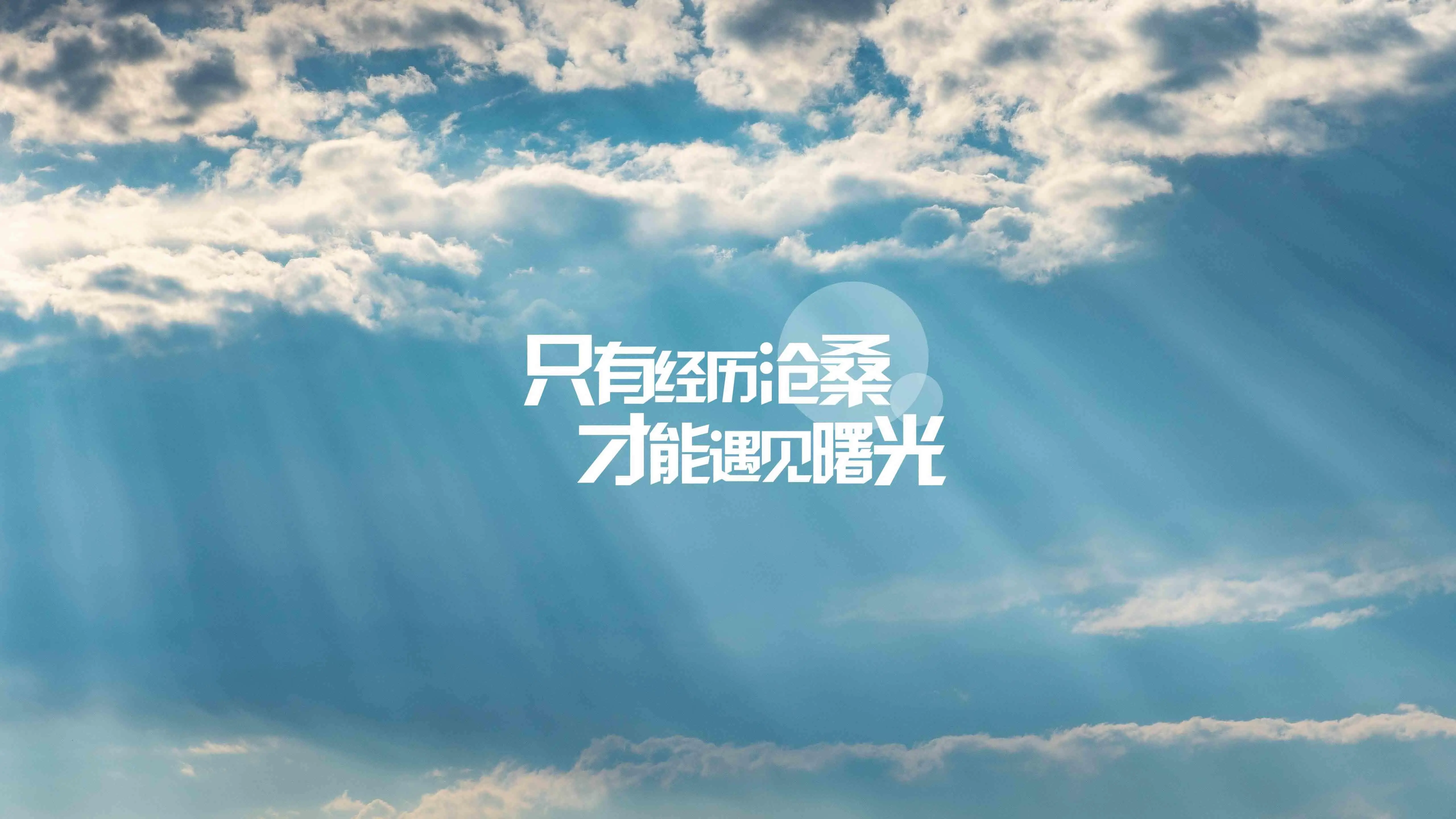
例子一
# 二选一的场景使用三元表达式更简洁
# 常用方法
nationality = input('请输入你的国籍>>>:')
if nationality == '中国':
print('龙的传人')
else:
print('外国友人')
# 三元表达式
nationality = input('请输入你的国籍>>>:')
res = '龙的传人' if nationality == '中国' else '外国友人'
print(res)
例子二
# 常用方法
def two_min(x, y):
if x > y:
print(y)
return y
else:
print(x)
return x
two_min(55, 66)
# 三元表达式
def two_min(x, y):
res = x if x < y else y
print(res)
two_min(88, 699)
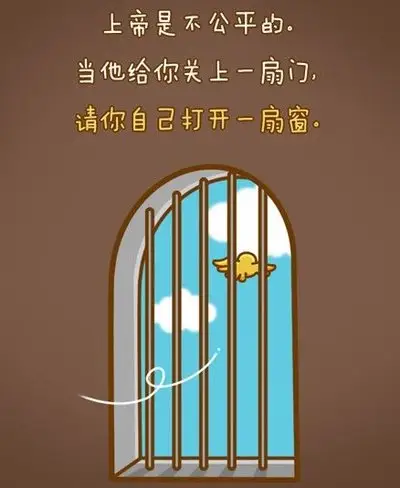
多种生成式
列表生成式
country_list = ['japan', 'italy', 'germany', 'france', 'canada', 'north korea']
'''要求:将列表中所有的国家名后面加上_capitalist'''
# 常见方式
# 创建一个空列表
new_list = []
# for循环列表获取每一个国家名
for item in country_list:
# 字符串末位拼接_capitalist
res = f'{item}_capitalist'
# 添加到新的列表中
new_list.append(res)
print(new_list)
# 列表生成式
# 列表生成式可以简化代码
res = [item+'_capitalist' for item in country_list]
print(res)
# 列表生成式还具备筛选能力
res = [item+'_capitalist' for item in country_list if item == 'germany']
print(res)
res = [item+'_capitalist' for item in country_list if item != 'north korea']
print(res)
字典生成式
l1 = ['name', 'age', 'height', 'hobby']
l2 = ['Jayce', 22, '188cm', 'swim']
# 要求:将上述两个列表分别制作成字典的键值
# 常规方法
# 定义一个空字典
new_dict = {}
# for循环取值
for i in range(len(l1)):
# 按照索引通过键添加
new_dict[l1[i]] = l2[i]
print(new_dict) # {'name': 'Jayce', 'age': 22, 'height': '188cm', 'hobby': 'swim'}
# 字典生成式
res = {l1[i]: l2[i] for i in range(len(l1))}
print(res) # {'name': 'Jayce', 'age': 22, 'height': '188cm', 'hobby': 'swim'}
res = {l1[i]: l2[i] for i in range(len(l1)) if i != 3}
print(res) # {'name': 'Jayce', 'age': 22, 'height': '188cm'}
集合生成式
res = {i for i in range(20)}
print(res, type(res)) # {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19} <class 'set'>
res = {i for i in range(20) if i % 3 != 0}
print(res, type(res)) # {1, 2, 4, 5, 7, 8, 10, 11, 13, 14, 16, 17, 19} <class 'set'>
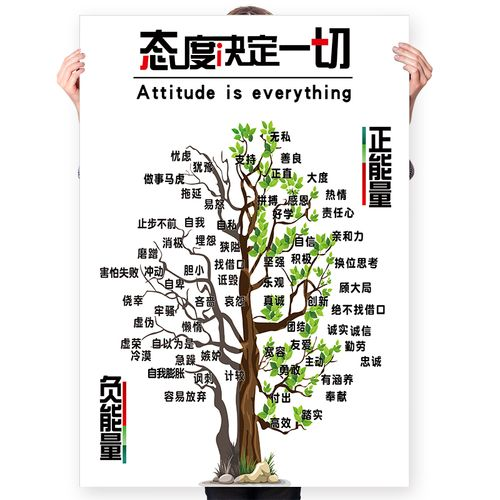
匿名函数
# 统计最大值
salary_info = {
'jack': 5000,
'pony': 4000,
'tom': 1000,
'zoe': 1500,
'annie': 2500
}
# 要求:统计出薪资最高的人的姓名
print(max(salary_info)) # zoe
'''
max底层可以看成是for循环依次比较,针对字典默认只能获取到k
获取到k之后如果是字符串的英文字母,就按照ASCII码表转成数字比较
A~Z:65-90
a~z:97-122
'''
def my_max(a):
return salary_info[a]
print(max(salary_info, key=my_max)) # jack
# 上述代码可以用匿名函数简写
print(max(salary_info, key=lambda key: salary_info[key])) # jack
常见的内置函数
常见重要内置函数
一、内置函数之map映射
l1 = [11, 22, 33, 44, 55, 66, 77]
# 需求:元素全部乘以5
# 方式1:列表生成式
l2 = [item * 5 for item in l1]
print(l2)
# 方式2:内置函数
def index(n):
return n * 5
res = map(index, l1)
print(res) # <map object at 0x000001AA3370A320>
print(list(res)) # [55, 110, 165, 220, 275, 330, 385]
res = map(lambda x: x * 5, l1)
print(list(res)) # [55, 110, 165, 220, 275, 330, 385]
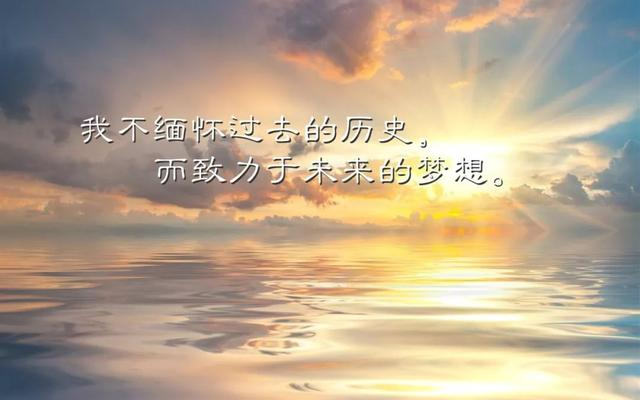
三、内置函数之zip拉链
l1 = ['001', '002', '003', '004']
l2 = ['tom', 'jack', 'sam', 'michael']
# 需求:将两个列表中的元素一一对应成对即可
res = zip(l1, l2) # <zip object at 0x000001E444599748>
print(res) # 用list可转换成列表
print(list(res)) # [('001', 'tom'), ('002', 'jack'), ('003', 'sam'), ('004', 'michael')]
'''zip可以整合多个数据集'''
l1 = ['001', '002', '003', '004']
l2 = ['tom', 'jack', 'sam', 'michael']
l3 = [1, 2, 3, 4]
l4 = [55, 66, 77, 88]
res = zip(l1, l2, l3, l4)
print(list(res)) # [('001', 'tom', 1, 55), ('002', 'jack', 2, 66), ('003', 'sam', 3, 77), ('004', 'michael', 4, 88)]
# 使用列表生成式
res1 = [(l1[i], l2[i], l3[i], l4[i]) for i in range(len(l1))]
print(res1) # [('001', 'tom', 1, 55), ('002', 'jack', 2, 66), ('003', 'sam', 3, 77), ('004', 'michael', 4, 88)]
'''zip在数据集之间个数不一致时,按照短的整合'''
l1 = ['001', '002', '003', '004']
l2 = ['tom', 'jack']
res = zip(l1, l2)
print(list(res)) # [('001', 'tom'), ('002', 'jack')]
三、内置函数之filter过滤
l1 = [111, 222, 333, 444, 555, 666]
'''需求:筛选出小于300的元素'''
# 方式1:列表生成式
# 方式2:内置函数
def index(x):
return x < 300
res = filter(index, l1)
print(list(res)) # [111, 222]
res = filter(lambda x: x < 300, l1)
print(list(res)) # [111, 222]
四、内置函数之reduce归总
from functools import reduce
l1 = [111, 222, 333, 444, 555]
# 需求:将列表中所有的元素相加
def index(x, y):
return x + y
res = reduce(index, l1)
print(res) # 1665
res = reduce(lambda x, y: x + y, l1)
print(res) # 1665
res = reduce(lambda x, y: x + y, l1, 666)
print(res) # 2331
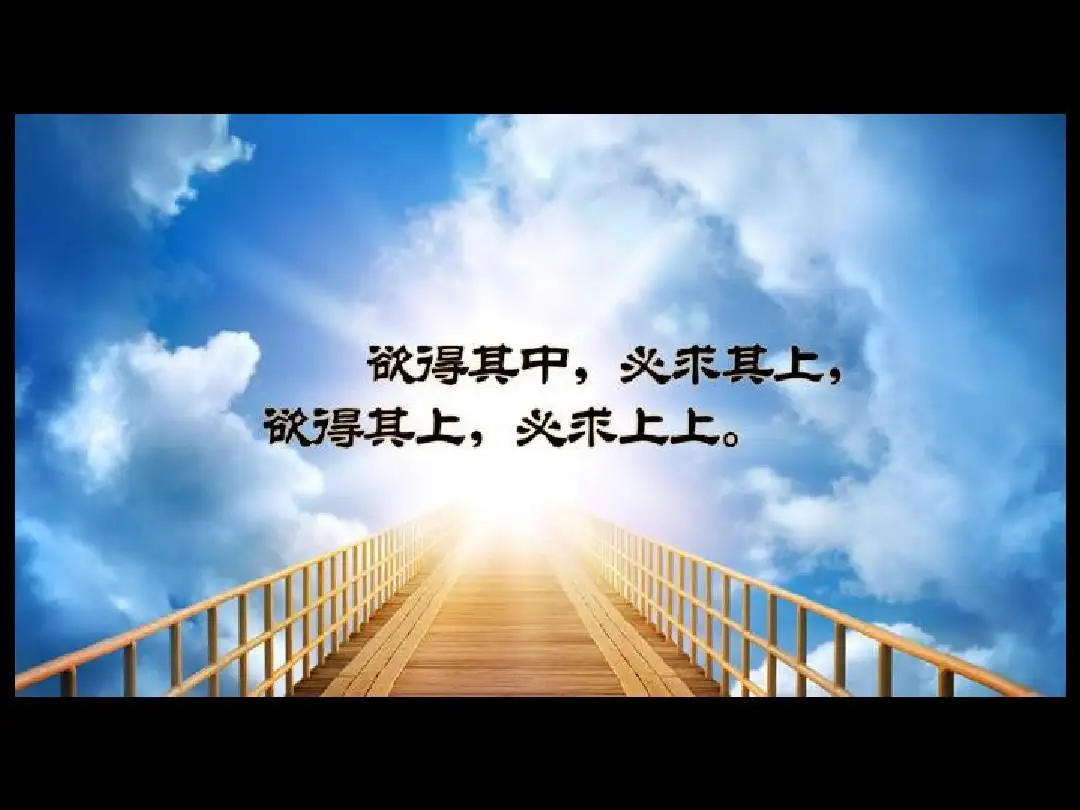
内置函数补充
# 1.abs() 获取绝对值(不考虑正负号)
print(abs(-123456))
print(abs(123456))
# 2.all()与any()
l1 = [0, 0, 111, 0, {}, (), True]
print(all(l1)) # False 数据集中必须所有的元素对应的布尔值为True返回的结果才是True
print(any(l1)) # True 数据集中只要所有的元素对应的布尔值有一个为True,返回的结果就是True
# 3.bin() oct() hex() 转换为对应的进制数
print(bin(1000)) # 0b1111101000
print(oct(1000)) # 0o1750
print(hex(1000)) # 0x3e8
# 4.bytes() 类型转换
s1 = 'One thing I know,that is I know nothing,我所知道的一件事就是我一无所知'
# 编码
res = bytes(s1, 'utf8')
print(
res) # b'One thing I know,that is I know nothing\xef\xbc\x8c\xe6\x88\x91\xe6\x89\x80\xe7\x9f\xa5\xe9\x81\x93\xe7\x9a\x84\xe4\xb8\x80\xe4\xbb\xb6\xe4\xba\x8b\xe5\xb0\xb1\xe6\x98\xaf\xe6\x88\x91\xe4\xb8\x80\xe6\x97\xa0\xe6\x89\x80\xe7\x9f\xa5'
# 解码
res1 = str(res, 'utf8')
print(res1) # One thing I know,that is I know nothing,我所知道的一件事就是我一无所知
# 5.callable() 判断当前对象是否可以加()调用
res = input('>>>:')
def index():
pass
print(callable(res)) # False 变量名不能加括号调用
print(callable(index)) # True 函数名可以加括号调用
# 6.chr()、ord() 字符与数字的对应转换
print(chr(98)) # b 根据数字转字符,依据ASCII码
print(ord('S')) # 83 根据字符转数字,依据ASCII码
# 7.dir() 查看数据类型可以调用的内置方法
print(dir(88.88))
print(dir('welcome'))
# 8.divmod()
print(divmod(808, 20)) # (40, 8)
print(divmod(800, 20)) # (40, 8)
print(divmod(799, 20)) # (39, 19)
print(divmod(800, 33)) # (24, 8)
def get_page_num(total_num, page_num):
num, others = divmod(total_num, page_num)
if others:
num += 1
print(f'一共需要{num}') # 一共需要46
get_page_num(999, 22)
# 9.enumerate() 枚举
name_list = ['jayce', 'tom', 'sam', 'johnson']
for item in name_list:
print(item)
for i, j in enumerate(name_list):
print(i, j) # i类似于是计数,默认从0开始
for i, j in enumerate(name_list, start=2):
print(i, j) # 第二个参数可以控制起始位置
# 10.eval() exec() 识别字符串中的python代码
print("input('>>>:')")
eval("input('>>>:')")
exec("input('>>>:')")
res = """
for i in range(5):
print(i * 5)
"""
exec(res)
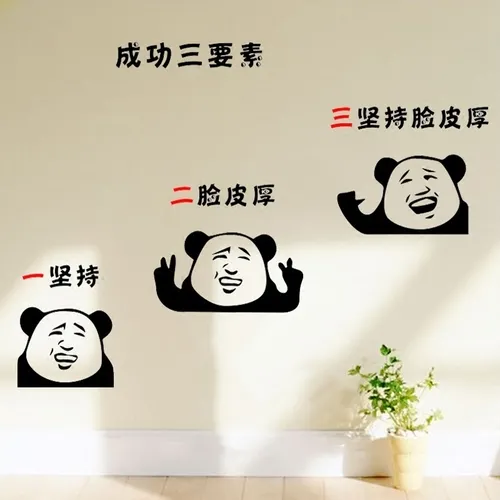