基本数据类型内置方法补充
目录
字符串的其他内置方法
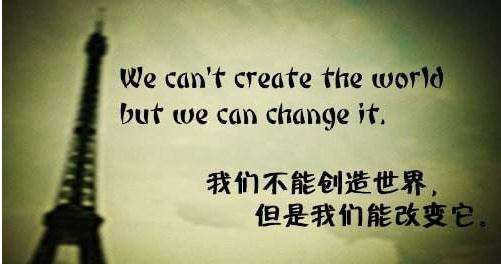
常见操作
1.移除字符串首尾的指定字符,可以自定义方向
str1 = '^^^^^trump^^^^^'
print(str1.strip('^')) # trump
print(str1.lstrip('^')) # trump^^^^^,移除字符串左边的指定字符
print(str1.rstrip('^')) # ^^^^^trump,移除字符串左边的指定字符
2.大小写相关操作
str2 = 'WlcOmETOChInA999'
print(str2.lower()) # 将所有的英文字母转化为小写wlcometochina999
print(str2.upper()) # 将所有的英文字母转化为大写WLCOMETOCHINA999
print(str2.isupper()) # 判断字符串中所有的英文字母是否是纯大写,布尔值为False
print(str2.islower()) # 判断字符串中所有的英文字母是否是纯小写,布尔值为False
# 验证码忽略大小写
verification_code = 'Q7w8' # 设定验证码
print('验证码是:{}'.format(verification_code)) # 把验证码显示给用户看
inp_user_code = input('请输入您看见的图片验证码>>>:').strip()
if verification_code.upper() == inp_user_code: # 验证码统一转大写或者小写都可以
print('验证码正确')
3.判断字符串的开头或者结尾是否是我们指定的字符
str3 = 'From small beginnings comes great things'
print(str3.startswith('f')) # 布尔值为False
print(str3.startswith('From')) # 布尔值为True
print(str3.startswith('small')) # 布尔值为False
print(str3.endswith('thing')) # 布尔值为False
print(str3.endswith('things')) # 布尔值为True
print(str3.endswith('comes')) # 布尔值为False
4.格式化输出
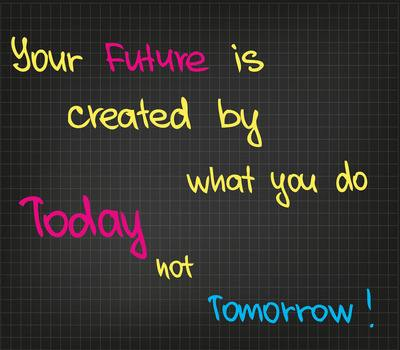
- fomat用法1:跟占位符相似,区别在于使用的{}占位
str4 = "American president's name is {}, his age is {}" # 定义格式化输入模板
print(str4.format('biden', 83)) # 关键字替换
- fomat用法2:根据索引取值,可以反复使用
str5 = "American president's name is {0} {0}, his {0} age is {1}" # 定义格式化输入模板
print(str5.format('biden', 83)) # 根据索引关键字替换
- 根据关键字的名字替换
str6 = "American president's name is {name} {name}, his {name} age is {age}" # 定义格式化输入模板
print(str6.format(name='biden', age=83)) # 根据关键字的名字替换
- 根据出现已经定义过的变量替换
name = 'biden' # 定义名字变量
age = 83 # 定义年龄变量
print(f"American president's name is {age} {name} {name}, his {name} age is {age}") # 根据出现已经定义过的变量替换
5. 拼接字符串
str7 = '仙路尽头谁为峰 一见无始道成空'
str8 = '沧海成尘几万秋 道尽黄发长生愁'
print(str7 + str8) # 仙路尽头谁为峰 一见无始道成空沧海成尘几万秋 道尽黄发长生愁
print(str8 * 5) # 打印五次
print('|'.join(str7)) # 仙|路|尽|头|谁|为|峰| |一|见|无|始|道|成|空
print('………'.join(['仙路尽头谁为峰', '一见无始道成空'])) # 仙路尽头谁为峰………一见无始道成空
print('^'.join([str8, str7])) # 沧海成尘几万秋 道尽黄发长生愁^仙路尽头谁为峰 一见无始道成空
6. 替换字符串中指定的字符
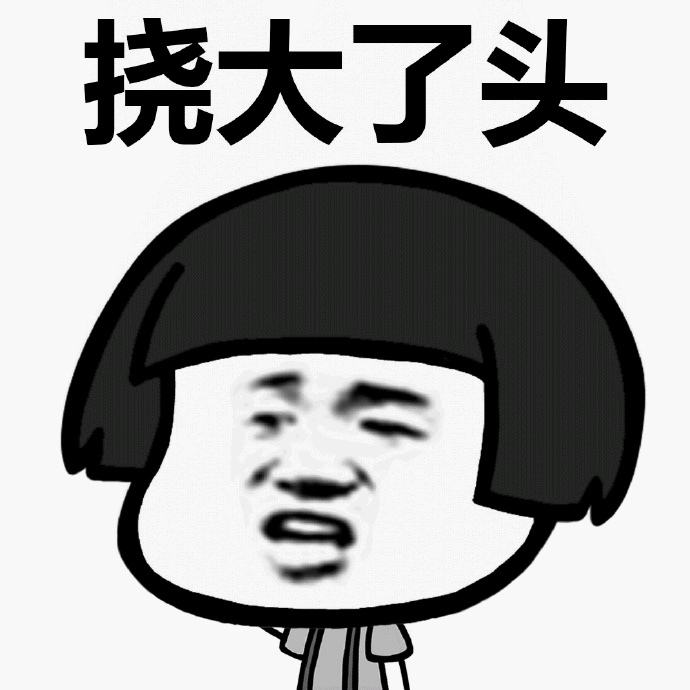
# 将money替换成power
print(str9.replace('money', 'power')) # 不指定替换的个数时,默认一次性替换所有
# 指定替换的个数
print(str9.replace('money', 'power', 2)) # 还可以通过第三个数字控制替换的个数,方向是从左往右
7. 判断字符串中是否是整数(整型)
str10 = 'sleepjoe111' # 定义变量
str11 = '110.120' # 定义变量
str12 = '8888888' # 定义变量
print(str10.isdigit()) # 判断结果为False
print(str11.isdigit()) # 判断结果为False
print(str12.isdigit()) # 判断结果为True
# 学生成绩输入系统
stu_score = input('please enter your exam score>>>:') # 输入成绩
if stu_score.isdigit() and 0 <= int(stu_score) <= 100: # 判断字符串是否为整数和在0-100之间
stu_score = int(stu_score) # 字符串转化为整数
print('你的成绩是', stu_score, '分') # 打印成绩
else: # if条件不成立,执行else子代码
print('胡乱填写,下次考试卷面扣十分')
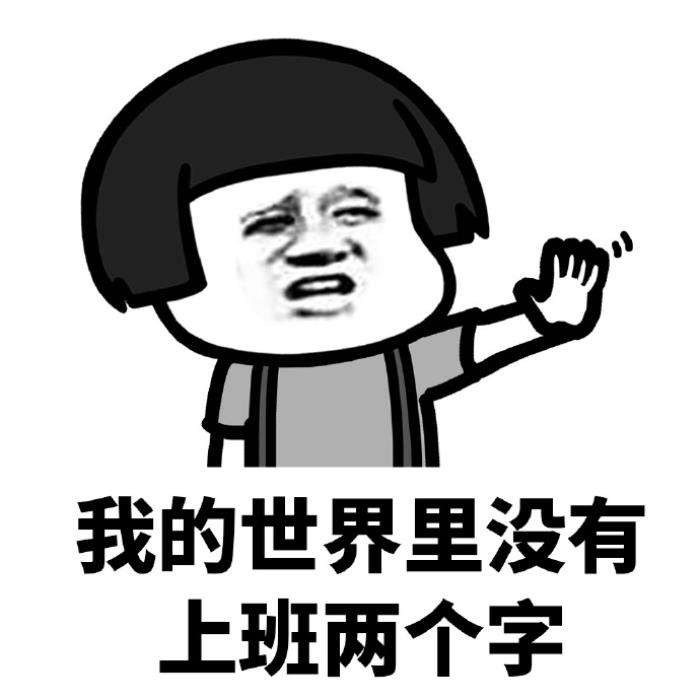
需要了解的操作
1.查找指定字符对应的索引值
str1 = 'Nothing is impossible to a willing heart'
print(str1.find('g')) # 结果为6,从左往右查找,查找一个就结束
print(str1.find('0', 1, 9)) # 结果是-1,意思是没找不到
print(str1.index('w')) # 结果为27
print(str1.index('i', 1, 9)) # 结果为4,找不到会报错,不推荐使用
2.文本位置改变
str2 = 'success'
print(str2.center(15, '^')) # ^^^^success^^^^
print(str2.ljust(15, '%')) # success%%%%%%%%
print(str2.rjust(15, '-')) # --------success
print(str2.zfill(15)) # 00000000success
3.特殊符号
print('Noth\aing i\rs impo\nssible') # s impo 会换行ssible,斜杠与一些英文字母的组合会产生特殊的含义
print(r'Noth\aing i\rs impo\nssible') # Noth\aing i\rs impo\nssible,在字符串的前面加一个字母r会取消它们的特殊含义
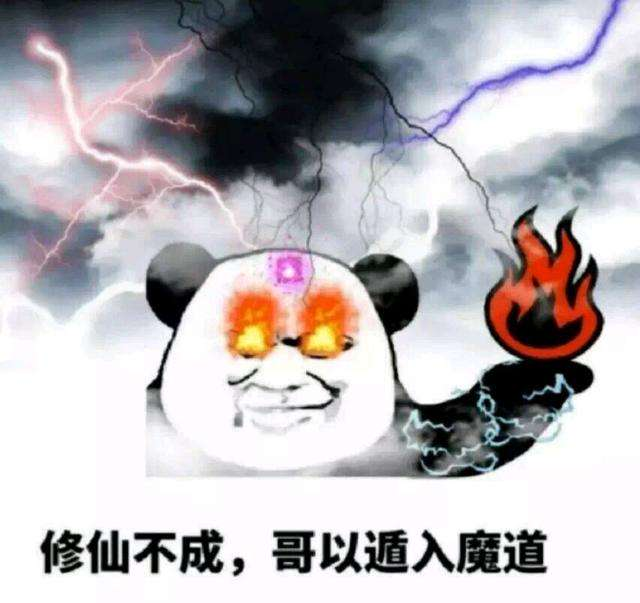
4.首字母大写,大小写翻转,每个单词的首字母大写
# 1.captalize:首字母大写
sentence = 'by falling we learn to go safely!'
sentence.capitalize()
print(sentence)
# 2.swapcase:大小写翻转
sentence2 = 'by falling we learn to go safely!'
print(sentence2.swapcase()) # BY FALLING WE LEARN TO GO SAFELY!
# 3.title:每个单词的首字母大写
sentence3 = 'by falling we learn to go safely!'
print(sentence3.title()) # By Falling We Learn To Go Safely!
列表内置方法
一.类型转换
# print(list(11)) # 报错
# print(list(11.11)) # 报错
# print(list(True)) # 报错
print(list('success')) # ['s', 'u', 'c', 'c', 'e', 's', 's']
print(list({'height': '175cm', 'weight': '55kg'})) # ['height', 'weight']
print(list((88, 72, 63, 54, 43))) # [88, 72, 63, 54, 43]
print(list({88, 72, 63, 54, 43})) # [72, 43, 54, 88, 63]
# list可以转换支持for循环的数据类型,包括字符串,字典,元组,集合
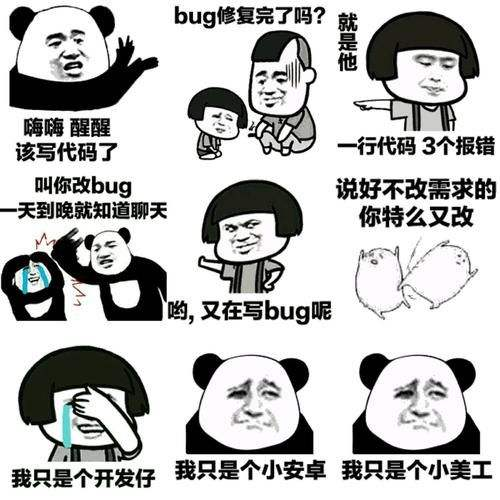
二、常见操作
1.索引取值
print(student_name_list[0]) # vive
print(student_name_list[-1]) # maly
2.切片操作
print(student_name_list[1:4]) # ['jack', 'michael', 'maly']
print(student_name_list[-4:-1]) # ['vive', 'jack', 'michael']
print(student_name_list[-1:-4:-1]) # ['maly', 'michael', 'jack']
3.间隔
print(student_name_list[0:4:1]) # ['vive', 'jack', 'michael', 'maly']
print(student_name_list[0:4:2]) # ['vive', 'michael']
print(student_name_list[-1:-4:-1]) # ['maly', 'michael', 'jack']
4.统计列表中元素的个数
print(len(student_name_list)) # 4
5.成员运算
print('j' in student_name_list) # False,注意最小判断单位是元素不是元素里面的单个字符
print('jack' in student_name_list) # True
6.列表添加元素的方式
1.尾部追加'单个'元素
student_name_list = ['vive', 'jack', 'michael', 'maly', 'annie']
student_name_list.append('老王')
print(student_name_list) # ['vive', 'jack', 'michael', 'maly', 'annie', '老王']
student_name_list.append(['小王', '张三', '胖虎'])
print(student_name_list) # ['vive', 'jack', 'michael', 'maly', 'annie', '老王', ['小王', '张三', '胖虎']]
2.指定位置插入'单个'元素
student_name_list.insert(4, '大熊')
student_name_list.insert(3, 'OBAMA')
student_name_list.insert(3, ['熊大', '熊二'])
print(student_name_list) # ['vive', 'jack', 'michael', ['熊大', '熊二'], 'OBAMA', 'maly', '大熊', 'annie', '老王', ['小王', '张三', '胖虎']]
3.合并列表
# 方式一
student_name_list.extend(['悟空', '八戒']) # extend其实可以看成是for循环+append
print(student_name_list) # ['vive', 'jack', 'michael', ['熊大', '熊二'], 'OBAMA', 'maly', '大熊', 'annie', '老王', ['小王', '张三', '胖虎'], '悟空', '八戒']
# 方式二
for i in ['悟空', '八戒']:
student_name_list.append(i)
print(student_name_list) # ['vive', 'jack', 'michael', ['熊大', '熊二'], 'OBAMA', 'maly', '大熊', 'annie', '老王', ['小王', '张三', '胖虎'], '悟空', '八戒', '悟空', '八戒'],如果把方式一代码注释,结果就一样
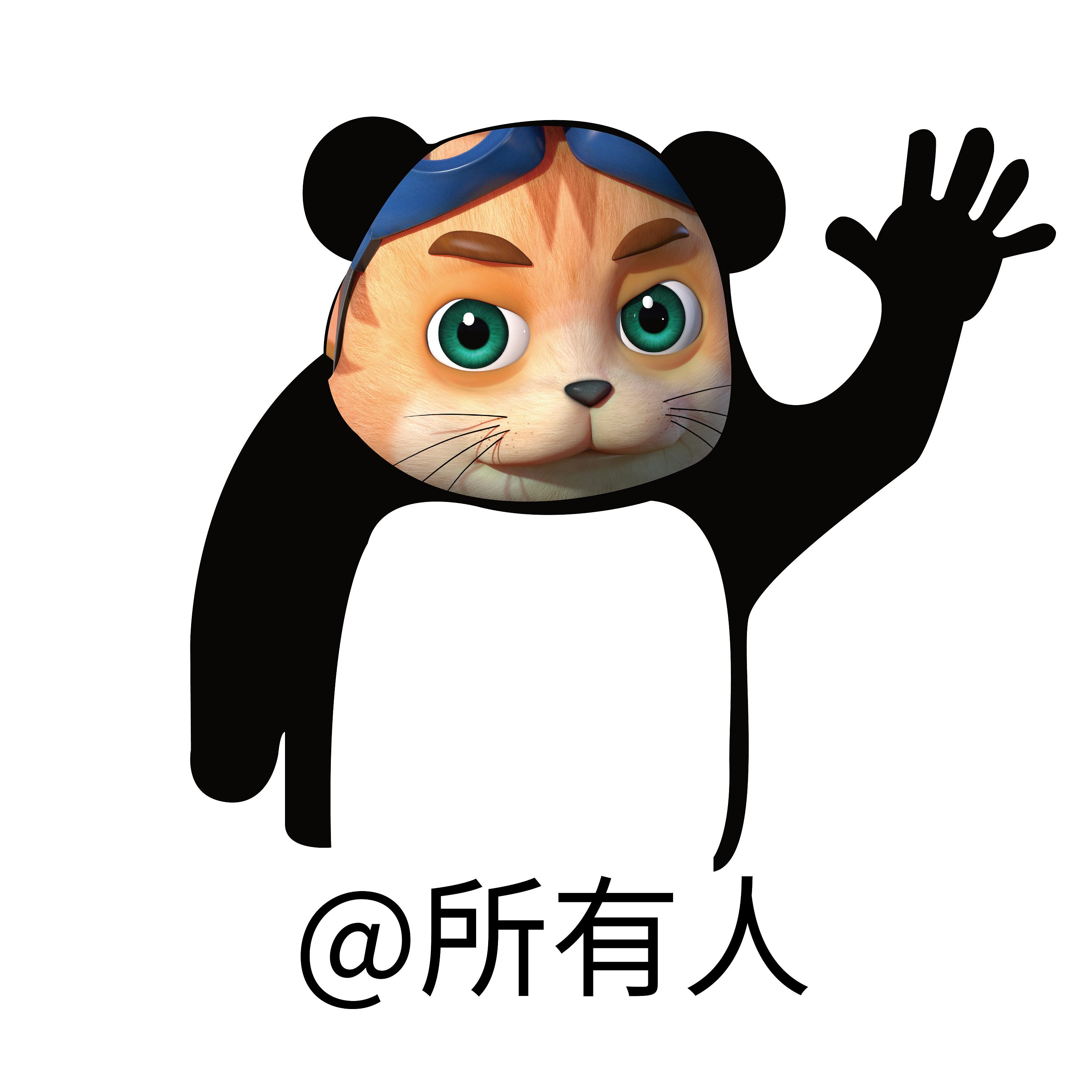
7.删除元素
# 1.通用的删除方式
student_name_list = ['vive', 'jack', 'michael', 'maly', 'annie']
del student_name_list[0]
print(student_name_list) # ['jack', 'michael', 'maly', 'annie']
# 2.就地删除
print(student_name_list.remove('maly')) # None,按照元素名称直接删除某个元素
print(student_name_list) # ['jack', 'michael', 'annie']
# 3.延迟删除
print(student_name_list.pop()) # annie,默认延迟删除尾部元素
print(student_name_list) # ['jack', 'michael']
print(student_name_list.pop(1)) # michael,可以指定索引值
print(student_name_list) # ['jack']
8.修改列表元素
student_name_list = ['vive', 'jack', 'michael', 'maly', 'annie']
print(id(student_name_list[0])) # 2232612999720
student_name_list[3] = 'trump'
print(id(student_name_list[0])) # 2232612999720
print(student_name_list) # ['vive', 'jack', 'michael', 'trump', 'annie']
9.排序
list1 = [555, 415, 122, 222, 66, 99, 44, 2222, 335]
list1.sort() # 默认是升序
print(list1) # [44, 66, 99, 122, 222, 335, 415, 555, 2222]
list1.sort(reverse=True) # 可以修改为降序
print(list1) # [2222, 555, 415, 335, 222, 122, 99, 66, 44]
10.翻转
list1 = [555, 415, 122, 222, 66, 99, 44, 2222, 335]
list1.reverse() # 前后颠倒
print(list1) # list1 = [555, 415, 122, 222, 66, 99, 44, 2222, 335]
11.比较运算
list1 = [555, 415, 122, 222, 66, 99, 44, 2222, 335]
list2 = [888, 446, 120]
print(list1 > list2) # False,列表在做比较的时候,比的是对应索引位置上的元素
s1 = ['D', 'C', 'B', 'A']
s2 = ['d', 'a']
print(s1 > s2) # False
print(list1.index(2222)) # 7
12.统计列表中某个元素出现的次数
list1 = [555, 415, 122, 222, 66, 99, 44, 2222, 335]
print(list1.count(222)) # 统计元素222出现的次数,次数为1
list1.clear() # 清空列表
print(list1) # []
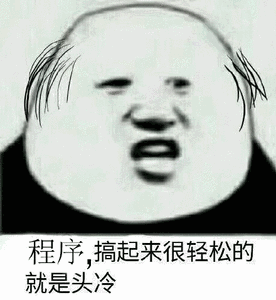