一、使用VS Code编写C++代码基础
参考:VScode在Windows下配置C++开发环境
https://zhuanlan.zhihu.com/p/96819625
一、
安装VS Code并安装插件C\C++,Cpptools【下载链接cpptools下载链接】
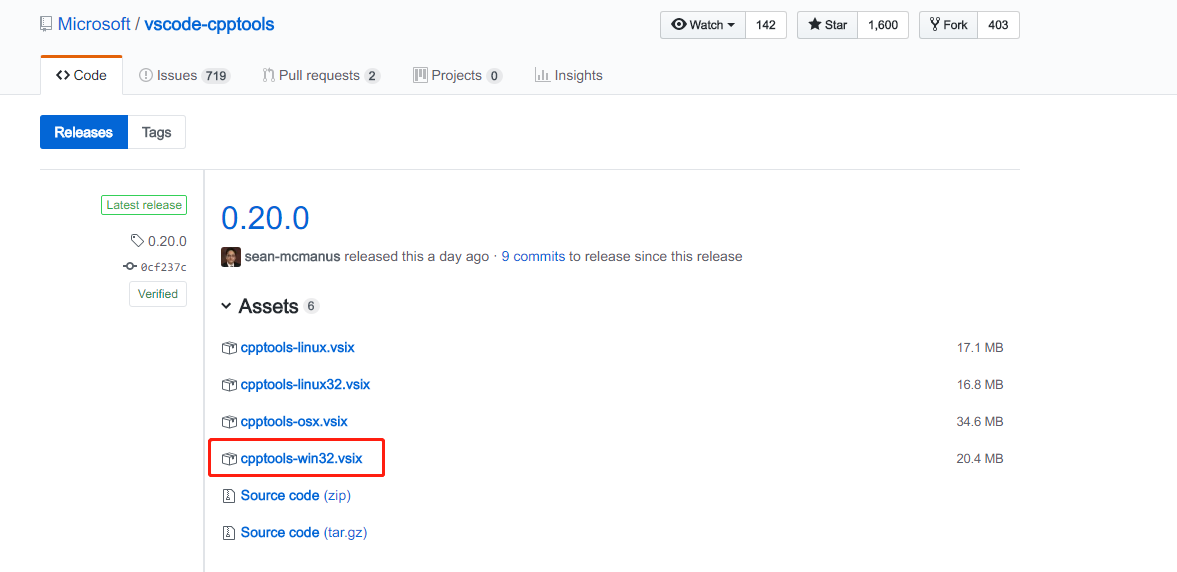
二、
新建工程目录如C++
在工程目录下面新建一个文件夹.vscode
在工程目录下面新建项目文件夹,如chapter
在chapter目录下面新建一个文件夹bin,用来存放编译生成的exe文件(可以不建bin目录,为了更好地管理文件,将.exe文件全放在chapter/bin目录下面)
在.vscode目录下加入3个json配置文件,
分别为c_cpp_properties.json、tasks.json和launch.json
c_cpp_properties.json内容如下所示:
{
"configurations": [
{
"name": "Win32",
"includePath": [
"${workspaceFolder}/**"
],
"defines": [
"_DEBUG",
"UNICODE",
"_UNICODE"
],
"compilerPath": "D:/application/minGW/mingw64/bin/g++.exe",
"cStandard": "gnu17",
"cppStandard": "gnu++14",
"intelliSenseMode": "windows-gcc-x64"
}
],
"version": 4
}
该文件主要说明编译器的位置等信息。
tasks.json文件内容如下:
{
"version": "2.0.0",
"tasks": [
{
"type": "cppbuild",
"label": "C/C++: cpp.exe 生成活动文件",
"command": "D:\\application\\minGW\\mingw64\\bin\\cpp.exe",
"args": [
"-fdiagnostics-color=always",
"-g",
"${file}",
"-o",
"${fileDirname}\\bin\\${fileBasenameNoExtension}.exe"
],
"options": {
"cwd": "${fileDirname}"
},
"problemMatcher": [
"$gcc"
],
"group": "build",
"detail": "编译器: D:\\application\\minGW\\mingw64\\bin\\cpp.exe"
},
{
"type": "cppbuild",
"label": "C/C++: g++.exe 生成活动文件",
"command": "D:/application/minGW/mingw64/bin/g++.exe",
"args": [
"-fdiagnostics-color=always",
"-g",
"${file}",
"-o",
"${fileDirname}\\bin\\${fileBasenameNoExtension}.exe"
],
"options": {
"cwd": "D:/application/minGW/mingw64/bin"
},
"problemMatcher": [
"$gcc"
],
"group": {
"kind": "build",
"isDefault": true
},
"detail": "调试器生成的任务。"
}
]
}
该文件主要说明将生成的exe文件放在什么地方。args参数中表明,将生成的exe文件放在 ${fileDirname}\bin 目录下。
launch.json 文件内容如下:
{
// 使用 IntelliSense 了解相关属性。
// 悬停以查看现有属性的描述。
// 欲了解更多信息,请访问: https://go.microsoft.com/fwlink/?linkid=830387
"version": "0.2.0",
"configurations": [
{
"name": "g++.exe - 生成和调试活动文件",
"type": "cppdbg",
"request": "launch",
"program": "${fileDirname}\\bin\\${fileBasenameNoExtension}.exe",
"args": [],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": true,
"MIMode": "gdb",
"miDebuggerPath": "D:\\application\\minGW\\mingw64\\bin\\gdb.exe",
"setupCommands": [
{
"description": "为 gdb 启用整齐打印",
"text": "-enable-pretty-printing",
"ignoreFailures": true
},
{
"description": "将反汇编风格设置为 Intel",
"text": "-gdb-set disassembly-flavor intel",
"ignoreFailures": true
}
],
"preLaunchTask": "C/C++: g++.exe 生成活动文件" // 调试前执行的任务,一般为编译程序。与tasks.json的label相对应
}
]
}
该文件主要用来指定运行exe文件所在的位置,是否在vs code 终端输出还是外部的cmd窗口输出。
编写单个的CPP文件:
在项目目录chapter目录下编写一个Hello.cpp文件。
#include <iostream>
using namespace std;
class Person{
public:
Person(int age,string name);
void showInfo();
~Person();
private:
int m_Age;
string m_Name;
};
void Person::showInfo(){
cout << "姓名:" <<this->m_Name << "年龄" << this ->m_Age << endl;
}
Person::Person(int age,string name){
this->m_Age = age;
this->m_Name = name;
cout << "调用Person的构造器" << endl;
}
Person::~Person(){
cout << "调用Person的析构函数" << endl;
}
void test01(){
Person p(10,"张三");
p.showInfo();
}
int main(int argc, char const *argv[])
{
// cout << "Hello World!" << endl;
test01();
system("pause");
return 0;
}
按F5 启动调试,即可输出想要的运行结果。
补充: 按F5运行后出现中文 乱码问题的解决方法。
问题不在于编译器和IDE工具,而在于cmd和powershell窗口。合理的解决方法是改 CMD/PowerShell 的输出编码为UTF-8。
针对Power Shell:
在 Win 图标处右键,打开 Windows PowerShell (管理员),执行命令
Set-ExecutionPolicy Unrestricted
新建文档 profile.ps1
用记事本编辑,粘贴以下代码并保存
$OutputEncoding = [console]::InputEncoding = [console]::OutputEncoding = New-Object System.Text.UTF8Encoding
把 profile.ps1 保存到以下路径:
C:\Windows\System32\WindowsPowerShell\v1.0
完成。
检测是否有效:
打开 PowerShell,执行:chcp
结果如下:Active code page: 65001,说明设置成功了
cmd 中文乱码解决:
https://www.cnblogs.com/vincezeng/p/6632878.html