c#中的多义关键字
new
new用三种场景,最常用的实例化,泛型参数时的实例化约束,第三种是显式隐藏继承成员。
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using static System.Console;
namespace KeyWordsDemo
{
class NewDemo : IDemo
{
public void Run()
{
//new 1
var table = new DataTable();
//new 2
Show<Parent>(new Child());
//new 3
Parent parent = new Child();
parent.Print();
}
//new 2
static void Show<T>(T t) where T : new()
{
WriteLine(t.GetType());
}
#region new 3
public class Parent
{
public virtual void Print()
{
WriteLine("Parent Print");
}
public virtual void View()
{
}
}
public class Child : Parent
{
//now 3
public new void Print()
{
WriteLine("Child Print");
}
public override void View()
{
}
}
#endregion
}
}
default
default有两种用法,一是返回类型的默认值,二是在switch中作为非选项值。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using static System.Console;
namespace KeyWordsDemo
{
class DefaultDemo : IDemo
{
public void Run()
{
//default 1
WriteLine(default(int));
WriteLine(default(bool));
WriteLine(default(DateTime));
WriteLine(default(string));
switch (default(string))
{
case "":
WriteLine("空字符串");
break;
case null:
WriteLine("null");
break;
//default 2
default:
WriteLine("其他");
break;
}
}
}
}
out
out有两种用法,一是作为方法输出参数,从方法内部返回数据,二是用在接口和委托的协变中,如案例中的interface IParent<out R>
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using static System.Console;
namespace KeyWordsDemo
{
class OutDemo : IDemo
{
public void Run()
{
//out 1
Computer(out int result);
WriteLine(result);
WriteLine("------------");
IParent<object> p1 = new Child<object>();
IParent<object> p2 = new Child<string>();
WriteLine(p1.GetType());
WriteLine(p2.GetType());
p1.Print();
p2.Print();
p1 = p2;
p1.Print();
}
//out 1
public void Computer(out int result)
{
result = 10;
}
//out 2
interface IParent<out R>
{
R Print();
}
class Child<R> : IParent<R>
{
public R Print()
{
var r = default(R);
WriteLine($"{typeof(R).Name}");
return r;
}
}
}
}
想要更快更方便的了解相关知识,可以关注微信公众号
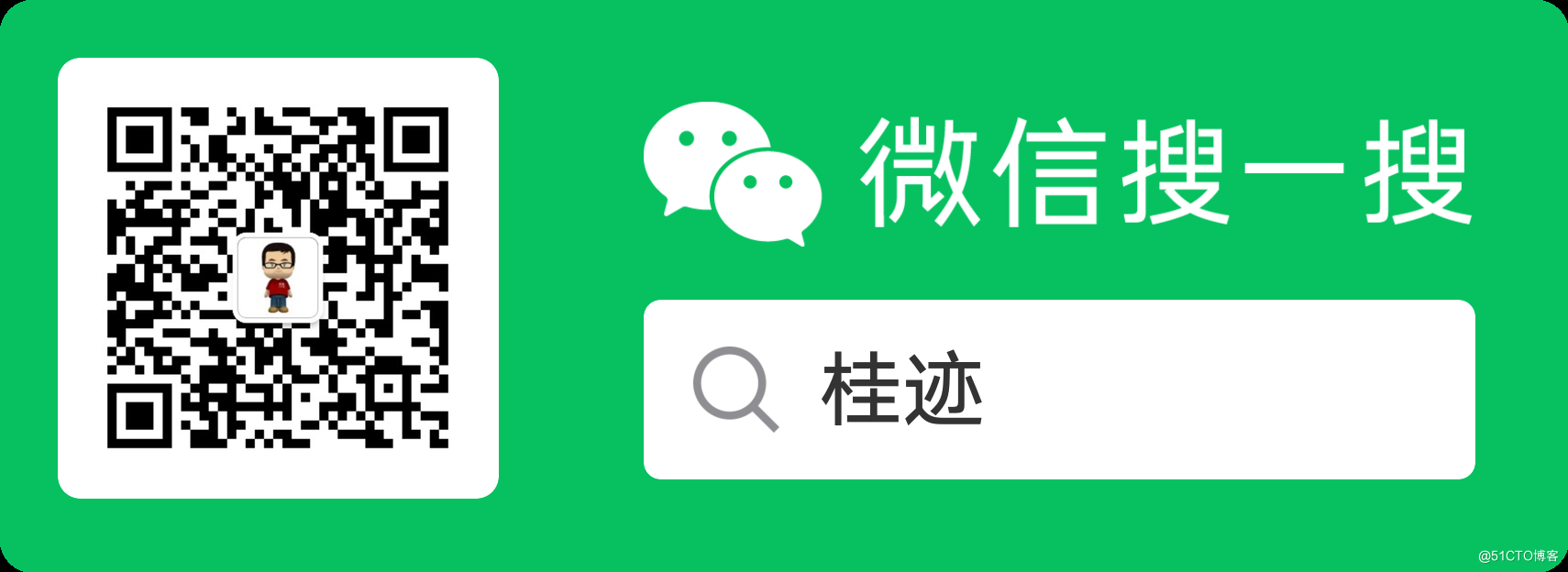