redis存放对象
一般会在Redis中存放键值对,那么如何存放一个对象呢?
1 import redis.clients.RedisClinet; 2 import redis.clients.SerializeUtil; 3 import redis.clients.jedis.Jedis; 4 5 public class Test { 6 7 /** 8 * Administrator 9 * @param args 10 */ 11 public static void main(String[] args) { 12 13 // 操作单独的文本串 14 Jedis redis= new Jedis( "10.2.31.38", 6379); 15 16 redis.set( "key", "value"); 17 System. out.println(redis.get( "key")); 18 System. out.println(redis.del( "key")); 19 20 // 操作实体类对象 21 Goods good= new Goods(); // 这个Goods实体我就不写了啊 22 good.setName( "洗衣机" ); 23 good.setNum(400); 24 good.setPrice(19l); 25 redis.set( "good".getBytes(), SerializeUtil. serialize(good)); 26 byte[] value = redis.get( "good".getBytes()); 27 Object object = SerializeUtil. unserialize(value); 28 if(object!= null){ 29 Goods goods=(Goods) object; 30 System. out.println(goods.getName()); 31 System. out.println(goods.getNum()); 32 System. out.println(goods.getPrice()); 33 } 34 System. out.println(redis.del( "good".getBytes())); 35 36 // 操作实体类对象2(实际上和上面是一样的) 37 String key= "goods-key"; 38 Goods g= new Goods(); 39 g.setName( "电风扇--d" ); 40 g.setNum(200); 41 String temp=RedisClinet. getInstance().set(g, key); 42 System. out.println(temp); 43 44 Object o=RedisClinet. getInstance().get(key); 45 if(o!= null) 46 { 47 Goods g1=(Goods)o; 48 System. out.println(g1.getName()); 49 System. out.println(g1.getNum()); 50 } 51 System. out.println(RedisClinet. getInstance().del(key)); 52 53 } 54 } 55 56 57 2 RedisClinet 客户端类 58 package redis.clients; 59 import redis.clients.jedis.Jedis; 60 /** 61 * 62 * @author ajun 63 * 64 */ 65 public class RedisClinet { 66 private static final String ip= "10.2.31.38"; 67 private static final int port=6379; 68 protected static RedisClinet redis = new RedisClinet (); 69 protected static Jedis jedis = new Jedis( ip, port);; 70 static { 71 72 } 73 protected RedisClinet(){ 74 System. out.println( " init Redis "); 75 } 76 public static RedisClinet getInstance() 77 { 78 return redis; 79 } 80 81 /**set Object*/ 82 public String set(Object object,String key) 83 { 84 return jedis.set(key.getBytes(), SerializeUtil.serialize(object)); 85 } 86 87 /**get Object*/ 88 public Object get(String key) 89 { 90 byte[] value = jedis.get(key.getBytes()); 91 return SerializeUtil. unserialize(value); 92 } 93 94 /**delete a key**/ 95 public boolean del(String key) 96 { 97 return jedis.del(key.getBytes())>0; 98 } 99 100 } 101 102 103 3 序列化工具类 104 105 /** 106 * 107 */ 108 package redis.clients; 109 110 import java.io.ByteArrayInputStream; 111 import java.io.ByteArrayOutputStream; 112 import java.io.ObjectInputStream; 113 import java.io.ObjectOutputStream; 114 115 /** 116 * @author Administrator 117 * 118 */ 119 public class SerializeUtil { 120 public static byte[] serialize(Object object) { 121 ObjectOutputStream oos = null; 122 ByteArrayOutputStream baos = null; 123 try { 124 // 序列化 125 baos = new ByteArrayOutputStream(); 126 oos = new ObjectOutputStream(baos); 127 oos.writeObject(object); 128 byte[] bytes = baos.toByteArray(); 129 return bytes; 130 } catch (Exception e) { 131 132 } 133 return null; 134 } 135 136 public static Object unserialize( byte[] bytes) { 137 ByteArrayInputStream bais = null; 138 try { 139 // 反序列化 140 bais = new ByteArrayInputStream(bytes); 141 ObjectInputStream ois = new ObjectInputStream(bais); 142 return ois.readObject(); 143 } catch (Exception e) { 144 145 } 146 return null; 147 } 148 }
喜欢请赞赏一下啦^_^
微信赞赏
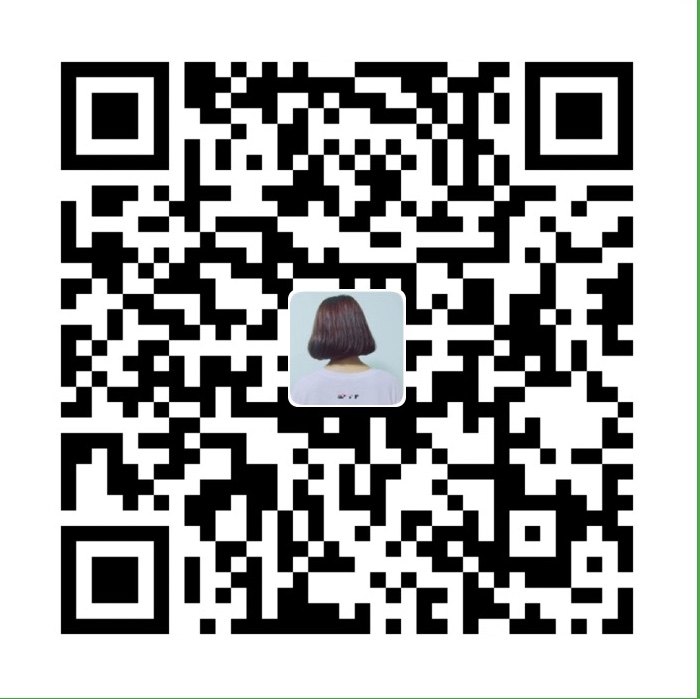
支付宝赞赏
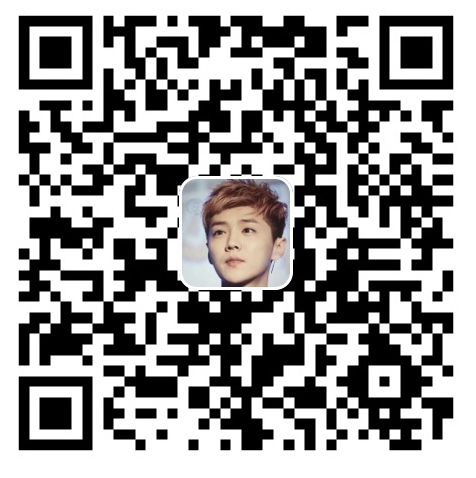