题目
只用位运算不用算术运算实现整数的加减乘除运算
java代码
package com.lizhouwei.chapter7;
/**
* @Description: 只用位运算不用算术运算实现整数的加减乘除运算
* @Author: lizhouwei
* @CreateDate: 2018/4/28 20:40
* @Modify by:
* @ModifyDate:
*/
public class Chapter7_3 {
//加法
public int add(int a, int b) {
int sum = a;
while (b != 0) {
sum = a ^ b;//无相位相加
b = (a & b) << 1;//只考虑进位
a = sum;
}
return sum;
}
//减法
public int sub(int a ,int b){
return add(a,negNum(b));
}
//乘法
public int muti(int a ,int b){
int res=0;
while (b!=0){
if((b&1)!=0){
res= add(res, a);
}
a=a<<1;
b = b>>>=1;
}
return res;
}
//取负数
public int negNum(int n){
return ~n+1;
}
//测试
public static void main(String[] args) {
Chapter7_3 chapter = new Chapter7_3();
int reslt = chapter.add(4, 5);
System.out.println("4+5=" + reslt);
reslt = chapter.sub(4, 5);
System.out.println("4-5=" + reslt);
reslt = chapter.muti(4,5);
System.out.println("4*5=" + reslt);
}
}
结果
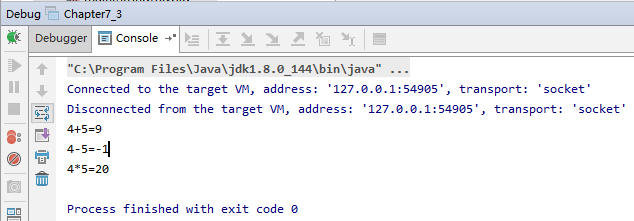