iOS_2022_动画
总结的不错:CALayer动画 ❤️❤️❤️❤️❤️
iOS CALayer仿射变换与3D变换(CGAffineTransform、 CATransform3D)
一、基础知识
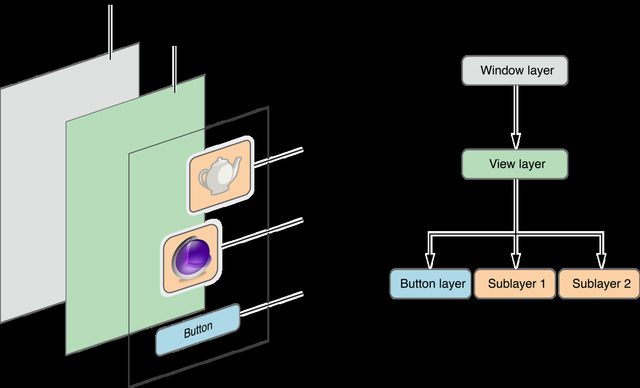
CALayer能做什么
-
图形阴影,边框,圆角等。
-
仿射变换。
-
3D变换。
-
透明遮罩,多级非线性动画...
1、基于UIView实现动画
1.1、简单的block动画
[UIView animateWithDuration:0.2 animations:^{ }];
[UIView animateWithDuration:0.5f animations:^{ } completion:^(BOOL finished) { // }];
1.2 、UIView [begin commit]
_demoView.frame = CGRectMake(0, SCREEN_HEIGHT/2-50, 50, 50);
[UIView beginAnimations:nil context:nil];
[UIView setAnimationDuration:1.0f];
_demoView.frame = CGRectMake(SCREEN_WIDTH, SCREEN_HEIGHT/2-50, 50, 50);
[UIView commitAnimations];
1.3、弹性动画
//参数:持续时间,延迟时间,阻尼系数,初始速度
[UIView animateWithDuration:0.8 delay:0 usingSpringWithDamping:0.5 initialSpringVelocity:6 options:UIViewAnimationOptionCurveEaseOut animations:^{ //
} completion:^(BOOL finished) {
}];
1.4、关键帧动画(中间可以添加合适多的帧来做不同的衔接动画)
[UIView animateKeyframesWithDuration:3.f delay:0.0 options:UIViewKeyframeAnimationOptionCalculationModeLinear animations:^{
[UIView addKeyframeWithRelativeStartTime:0.0 relativeDuration:0.8 animations:^{
//
}];
[UIView addKeyframeWithRelativeStartTime:0.8 relativeDuration:1.6 animations:^{
//
}];
} completion:^(BOOL finished) {
//
}];
2、CALayer动画
值得注意的是 CASpringAnimation 是 CABasicAnimation 的子类用于实现弹性动画的效果。
常用属性:duration : 动画的持续时间
beginTime : 动画的开始时间
repeatCount : 动画的重复次数
autoreverses : 执行的动画按照原动画返回执行
timingFunction : 控制动画的显示节奏系统提供五种值选择,
kCAMediaTimingFunctionLinear 线性动画
kCAMediaTimingFunctionEaseIn 先慢后快(慢进快出)
kCAMediaTimingFunctionEaseOut
kCAMediaTimingFunctionEaseInEaseOut
kCAMediaTimingFunctionDefault
path:关键帧动画中的执行路径
type : 过渡动画的动画类型,系统提供了四种过渡动画。
kCATransitionFade 渐变效果
kCATransitionMoveIn 进入覆盖效果
kCATransitionPush
kCATransitionReveal
subtype : 过渡动画的动画方向
kCATransitionFromRight 从右侧进入
kCATransitionFromLeft 从左侧进入
kCATransitionFromTop
kCATransitionFromBottom
2.1、基础动画(CABaseAnimation)
CAKeyframeAnimation和CABaseAnimation都属于CAPropertyAnimatin的子类。CABaseAnimation只能从一个数值(fromValue)变换成另一个数值(toValue),而CAKeyframeAnimation则会使用一个NSArray保存一组关键帧。 `
基础动画主要提供了对于CALayer对象中的可变属性进行简单动画的操作。比如:位移、透明度、缩放、旋转、背景色等等。
重要属性
fromValue : keyPath对应的初始值
toValue : keyPath对应的结束值
CABasicAnimation* rotationAnimation = [CABasicAnimation animationWithKeyPath:@"transform.rotation.z"];
rotationAnimation.duration = 0.3f;
rotationAnimation.repeatCount = 1;
rotationAnimation.autoreverses = NO;
rotationAnimation.fromValue = [NSNumber numberWithFloat: beginValue*M_PI];
rotationAnimation.toValue = [NSNumber numberWithFloat:endValue*M_PI];
rotationAnimation.removedOnCompletion = NO;
rotationAnimation.fillMode = kCAFillModeForwards;
rotationAnimation.timingFunction = [CAMediaTimingFunction functionWithName:kCAMediaTimingFunctionEaseInEaseOut];
[pinLayer addAnimation:rotationAnimation forKey:@"revItUpAnimation"];
2.2、关键帧动画(CAKeyframeAnimation)
CAKeyframeAnimation和CABaseAnimation都属于CAPropertyAnimatin的子类。`CABaseAnimation只能从一个数值(fromValue)变换成另一个数值(toValue),而CAKeyframeAnimation则会使用一个NSArray保存一组关键帧。 `
重要属性
values : 就是上述的NSArray对象。里面的元素称为”关键帧”(keyframe)。动画对象会在指定的时间(duration)内,依次显示values数组中的每一个关键帧
path : 可以设置一个CGPathRef\CGMutablePathRef,让层跟着路径移动。path只对CALayer的anchorPoint和position起作用。如果你设置了path,那么values将被忽略。
keyTimes : 可以为对应的关键帧指定对应的时间点,其取值范围为0到1.0,keyTimes中的每一个时间值都对应values中的每一帧.当keyTimes没有设置的时候,各个关键帧的时间是平分的。
calculationMode:
const kCAAnimationLinear//线性,默认
const kCAAnimationDiscrete//离散,无中间过程(没有中间圆滑的过渡),但keyTimes设置的时间依旧生效,物体跳跃地出现在各个关键帧上
const kCAAnimationPaced//平均,keyTimes跟timeFunctions失效 const kCAAnimationCubic//平均,同上
const kCAAnimationCubicPaced//平均,同上
2.3、组合动画
CAKeyframeAnimation *anima1 = [CAKeyframeAnimation animationWithKeyPath:@"position"];
NSValue *value0 = [NSValue valueWithCGPoint:CGPointMake(0, SCREEN_HEIGHT/2-50)];
NSValue *value1 = [NSValue valueWithCGPoint:CGPointMake(SCREEN_WIDTH/3, SCREEN_HEIGHT/2-50)];
NSValue *value2 = [NSValue valueWithCGPoint:CGPointMake(SCREEN_WIDTH/3, SCREEN_HEIGHT/2+50)];
NSValue *value3 = [NSValue valueWithCGPoint:CGPointMake(SCREEN_WIDTH*2/3, SCREEN_HEIGHT/2+50)];
NSValue *value4 = [NSValue valueWithCGPoint:CGPointMake(SCREEN_WIDTH*2/3, SCREEN_HEIGHT/2-50)];
NSValue *value5 = [NSValue valueWithCGPoint:CGPointMake(SCREEN_WIDTH, SCREEN_HEIGHT/2-50)];
anima1.values = [NSArray arrayWithObjects:value0,value1,value2,value3,value4,value5, nil];
//缩放动画
CABasicAnimation *anima2 = [CABasicAnimation animationWithKeyPath:@"transform.scale"];
anima2.fromValue = [NSNumber numberWithFloat:0.8f];
anima2.toValue = [NSNumber numberWithFloat:2.0f];
//旋转动画
CABasicAnimation *anima3 = [CABasicAnimation animationWithKeyPath:@"transform.rotation"];
anima3.toValue = [NSNumber numberWithFloat:M_PI*4];
//组动画
CAAnimationGroup *groupAnimation = [CAAnimationGroup animation];
groupAnimation.animations = [NSArray arrayWithObjects:anima1,anima2,anima3, nil];
groupAnimation.duration = 4.0f;
[_demoView.layer addAnimation:groupAnimation forKey:@"groupAnimation"];
三、仿射变换CGAffineTransform和CATransform3D
CGAffineTransform和CATransform3D
CGAffineTransform | CATransform3D |
An affine transformation matrix is used to rotate, scale, translate, or skew the objects you draw in a graphics context. The CGAffineTransform type provides functions for creating, concatenating, and applying affine transformations. 仿射变换矩阵用于旋转、缩放、平移或倾斜您在图形上下文中绘制的对象。 CGAffineTransform 类型提供用于创建、连接和应用仿射变换的函数。 |
The transform matrix is used to rotate, scale, translate, skew, and project the layer content. Functions are provided for creating, concatenating, and modifying CATransform3D data. 变换矩阵用于旋转、缩放、平移、倾斜和投影图层内容。提供了用于创建、连接和修改 CATransform3D 数据的函数。 |
CGAffineTransform是作用于View的主要为2D变换, | CGAffineTransform是作用于View的主要为2D变换, |
UIView的transform属性 @property(nonatomic) CGAffineTransform transform; @property(nonatomic) CATransform3D transform3D; |
CALayer的属性 @property CATransform3D transform; - (CGAffineTransform)affineTransform; - (void)setAffineTransform:(CGAffineTransform)m; |
ios transform 平移 缩放 旋转 CGAffineTransform |
CGAffineTransform
/// 用来连接两个变换效果并返回。返回的t = t1 * t2
CGAffineTransformConcat(CGAffineTransform t1, CGAffineTransform t2)
/// 矩阵初始值。[ 1 0 0 1 0 0 ]
CGAffineTransformIdentity
/// 自定义矩阵变换,需要掌握矩阵变换的知识才知道怎么用。参照上面推荐的原理链接
CGAffineTransformMake(CGFloat a, CGFloat b, CGFloat c, CGFloat d, CGFloat tx, CGFloat ty)
/// 旋转视图。传入参数为 角度 * (M_PI / 180)。等同于 CGAffineTransformRotate(self.transform, angle)
CGAffineTransformMakeRotation(CGFloat angle)
CGAffineTransformRotate(CGAffineTransform t, CGFloat angle)
/// 缩放视图。等同于CGAffineTransformScale(self.transform, sx, sy)
CGAffineTransformMakeScale(CGFloat sx, CGFloat sy)
CGAffineTransformScale(CGAffineTransform t, CGFloat sx, CGFloat sy)
/// 缩放视图。等同于CGAffineTransformTranslate(self.transform, tx, ty)
CGAffineTransformMakeTranslation(CGFloat tx, CGFloat ty)
CGAffineTransformTranslate(CGAffineTransform t, CGFloat tx, CGFloat ty)
//特殊地,transform属性默认值为CGAffineTransformIdentity,可以在形变之后设置该值以还原到最初状态
self.demoImageView.transform = CGAffineTransformIdentity;//样例
四、关于CAPropertyAnimation的构造函数animationWithKeyPath的参数
以StrokeStart和StrokeEnd作为KeyPath的动画:StrokeEnd && StrokeStart
一些常用的animationWithKeyPath值的总结(都是 CAShapeLayer 或者 CALayer 的属性)
#都是 CAShapeLayer 或者 CALayer 的属性
strokeStart #一条Path的起始
strokeEnd #一条Path的终止的位置
transform.scale 比例转化 @(0.8)
transform.scale.x 宽的比例 @(0.8)
transform.scale.y 高的比例 @(0.8)
transform.translation.x 往x轴方向移动
transform.translation.y 往y轴方向移动
transform.rotation.x 围绕x轴旋转 @(M_PI)
transform.rotation.y 围绕y轴旋转 @(M_PI)
transform.rotation.z 围绕z轴旋转 @(M_PI)
cornerRadius 圆角的设置 @(50)
backgroundColor 背景颜色的变化 (id)[UIColor purpleColor].CGColor
bounds 大小,中心不变 [NSValue valueWithCGRect:CGRectMake(0, 0, 200, 200)];
position 位置(中心点的改变) [NSValue valueWithCGPoint:CGPointMake(300, 300)];
contents 内容,比如UIImageView的图片 imageAnima.toValue = (id)[UIImage imageNamed:@"to"].CGImage;
opacity 透明度 @(0.7)
contentsRect.size.width 横向拉伸缩放 @(0.4)最好是0~1之间的