模拟QQ聊天系统-安卓源代码
利用课余时间随便写的一个小东西,都是一起学习。
先上图:
package com.example.nanchen.listviewdemo.adapter; import android.content.Context; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ArrayAdapter; import android.widget.LinearLayout; import android.widget.TextView; import com.example.nanchen.listviewdemo.adapter_content.Msg; import com.example.nanchen.listviewdemo.R; import java.util.List; /** * Created by nanchen on 2016/3/28. * */ public class MsgAdapter extends ArrayAdapter<Msg> { private int resourceId; public MsgAdapter(Context context, int resource, List<Msg> objects) { super(context, resource, objects); resourceId = resource; } @Override public View getView(int position, View convertView, ViewGroup parent) { Msg msg = getItem(position); viewHolder viewHolder; if(convertView == null){ convertView = LayoutInflater.from(getContext()).inflate(resourceId,null); viewHolder = new viewHolder(); viewHolder.leftLayout = (LinearLayout) convertView.findViewById(R.id.left_layout); viewHolder.rightLayout = (LinearLayout) convertView.findViewById(R.id.right_layout); viewHolder.leftMsg = (TextView) convertView.findViewById(R.id.left_msg); viewHolder.rightMsg = (TextView) convertView.findViewById(R.id.right_msg); convertView.setTag(viewHolder); }else{ viewHolder = (MsgAdapter.viewHolder) convertView.getTag(); } if (msg.getType() == Msg.TYPE_RECEIVED){//若是收到的消息,则隐藏右布局,显示左布局 viewHolder.leftLayout.setVisibility(View.VISIBLE); viewHolder.rightLayout.setVisibility(View.GONE); viewHolder.leftMsg.setText(msg.getContent()); }else if(msg.getType() == Msg.TYPE_SENT){ viewHolder.leftLayout.setVisibility(View.GONE); viewHolder.rightLayout.setVisibility(View.VISIBLE); viewHolder.rightMsg.setText(msg.getContent()); } return convertView; } class viewHolder{ LinearLayout leftLayout; LinearLayout rightLayout; TextView leftMsg; TextView rightMsg; } }
package com.example.nanchen.listviewdemo.adapter; import android.content.Context; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ArrayAdapter; import android.widget.ImageView; import android.widget.TextView; import com.example.nanchen.listviewdemo.adapter_content.Person; import com.example.nanchen.listviewdemo.R; import java.util.List; /** * * Created by nanchen on 2016/3/22. */ public class PersonAdapter extends ArrayAdapter<Person> { private int resourceId; public PersonAdapter(Context context, int textViewResourceId, List<Person> objects) { super(context, textViewResourceId, objects); resourceId = textViewResourceId; } @Override public View getView(int position, View convertView, ViewGroup parent) { Person person = getItem(position); ViewHolder viewHolder; if(convertView == null){ convertView = LayoutInflater.from(getContext()).inflate(resourceId ,null); viewHolder = new ViewHolder(); viewHolder.imageView_person = (ImageView) convertView.findViewById(R.id.imageView_person); viewHolder.textView_name = (TextView) convertView.findViewById(R.id.textView_name); convertView.setTag(viewHolder); }else{ // view = convertView; viewHolder = (ViewHolder) convertView.getTag(); } viewHolder.imageView_person.setImageResource(person.getImageId()); viewHolder.textView_name.setText(person.getName()); return convertView; } class ViewHolder{ ImageView imageView_person; TextView textView_name; } }
package com.example.nanchen.listviewdemo.adapter_content; /** * Created by nanchen on 2016/3/28. * 存储消息类型和内容的类 */ public class Msg { public static final int TYPE_RECEIVED = 0; public static final int TYPE_SENT = 1; private String content; private int type; public Msg(String content, int type) { this.content = content; this.type = type; } public String getContent() { return content; } public int getType() { return type; } }
package com.example.nanchen.listviewdemo.adapter_content; /** * Created by nanchen on 2016/3/22. */ public class Person { private String name; private int imageId; public Person(String name, int imageId) { this.name = name; this.imageId = imageId; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getImageId() { return imageId; } public void setImageId(int imageId) { this.imageId = imageId; } }
1 package com.example.nanchen.listviewdemo.data; 2 3 import android.os.Parcel; 4 import android.os.Parcelable; 5 6 /** 7 * 8 * Created by nanchen on 2016/3/22. 9 */ 10 public class User implements Parcelable { 11 12 private String userName; 13 private String pwd; 14 15 public String getPwd() { 16 return pwd; 17 } 18 19 public void setPwd(String pwd) { 20 this.pwd = pwd; 21 } 22 23 public String getUserName() { 24 return userName; 25 } 26 27 public void setUserName(String userName) { 28 this.userName = userName; 29 } 30 31 @Override 32 public int describeContents() { 33 return 0; 34 } 35 36 @Override 37 public void writeToParcel(Parcel dest, int flags) { 38 dest.writeString(userName); 39 dest.writeString(pwd); 40 } 41 42 //对象的创建器 43 public static final Parcelable.Creator<User> CREATOR = new Parcelable.Creator<User>(){ 44 45 46 @Override 47 public User createFromParcel(Parcel source) { 48 User user = new User(); 49 user.userName = source.readString(); 50 user.pwd = source.readString(); 51 return user; 52 } 53 54 @Override 55 public User[] newArray(int size) { 56 return new User[0]; 57 } 58 }; 59 }
1 package com.example.nanchen.listviewdemo; 2 3 import android.app.Activity; 4 5 import java.util.ArrayList; 6 import java.util.List; 7 8 /** 9 * Created by nanchen on 2016/3/28. 10 * 退出程序的最佳方案,写一个活动收集器 11 */ 12 public class ActivityCollector { 13 public static List<Activity> activities = new ArrayList<>(); 14 15 public static void addActivity(Activity activity){ 16 activities.add(activity); 17 } 18 19 public static void removeActivity(Activity activity){ 20 activities.remove(activity); 21 } 22 23 public static void finishAll(){ 24 for (Activity activity:activities){ 25 if (!activity.isFinishing()){ 26 activity.finish(); 27 } 28 } 29 } 30 }
1 package com.example.nanchen.listviewdemo; 2 3 import android.app.Activity; 4 import android.os.Bundle; 5 import android.util.Log; 6 7 /** 8 * Created by nanchen on 2016/3/28. 9 * 用于知晓当前是在哪一个活动 10 * 活动的最佳写法 11 */ 12 public class BaseActivity extends Activity { 13 @Override 14 protected void onCreate(Bundle savedInstanceState) { 15 super.onCreate(savedInstanceState); 16 Log.d("BaseActivity", getClass().getSimpleName()); 17 ActivityCollector.addActivity(this); 18 } 19 20 @Override 21 protected void onDestroy() { 22 super.onDestroy(); 23 ActivityCollector.removeActivity(this); 24 } 25 }
package com.example.nanchen.listviewdemo; import android.content.DialogInterface; import android.content.Intent; import android.os.Bundle; import android.support.v7.app.AlertDialog; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.ListView; import android.widget.TextView; import android.widget.Toast; import com.example.nanchen.listviewdemo.adapter.MsgAdapter; import com.example.nanchen.listviewdemo.adapter_content.Msg; import java.util.ArrayList; import java.util.List; public class ChatActivity extends BaseActivity { private ListView listView_msg; private EditText editText_input; private Button button_send,button_back,button_add; private MsgAdapter msgAdapter; private List<Msg> msgList = new ArrayList<>(); private TextView textView_person_name; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_chat); initMsg(); msgAdapter = new MsgAdapter(this,R.layout.msg_item,msgList); listView_msg = (ListView) findViewById(R.id.listView_msg); listView_msg.setAdapter(msgAdapter); editText_input = (EditText) findViewById(R.id.editText_send); editText_input.clearFocus(); button_send = (Button) findViewById(R.id.button_send); button_send.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { String content = editText_input.getText().toString(); if(content.isEmpty()){//用户输入消息为空,则弹出一个警告框 final AlertDialog.Builder builder = new AlertDialog.Builder(ChatActivity.this); builder.setTitle("警告"); builder.setMessage("输入的消息不能为空!"); builder.setPositiveButton("确定", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { dialog.dismiss(); } }); builder.show(); }else{ Msg msg = new Msg(content,Msg.TYPE_SENT); msgList.add(msg); msgAdapter.notifyDataSetChanged();//当有新消息时,刷新ListView中的显示 listView_msg.setSelection(msgList.size());//将ListView定位到最后一行 editText_input.setText("");//清空输入框中的内容 } } }); button_back = (Button) findViewById(R.id.button_back); button_back.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { finish(); } }); button_add = (Button) findViewById(R.id.button_add); button_add.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Toast.makeText(ChatActivity.this,"你点击了添加按钮!!!",Toast.LENGTH_SHORT).show(); } }); textView_person_name = (TextView) findViewById(R.id.textView_person_name); Intent intent = getIntent(); String person_name = intent.getStringExtra("PERSON_NAME"); textView_person_name.setText(person_name); } private void initMsg() { Msg msg = new Msg("Hello!",Msg.TYPE_SENT); msgList.add(msg); Msg msg1 = new Msg("Hello!How are you?",Msg.TYPE_RECEIVED); msgList.add(msg1); Msg msg2 = new Msg("I am fine,and you?",Msg.TYPE_SENT); msgList.add(msg2); Msg msg3 = new Msg("I am ok!",Msg.TYPE_RECEIVED); msgList.add(msg3); } }
package com.example.nanchen.listviewdemo; import android.content.Context; import android.content.Intent; import android.content.SharedPreferences; import android.os.Bundle; import android.text.TextUtils; import android.view.View; import android.widget.Button; import android.widget.CheckBox; import android.widget.CompoundButton; import android.widget.EditText; import android.widget.ImageButton; import android.widget.Toast; import com.example.nanchen.listviewdemo.data.User; public class LoginActivity extends BaseActivity { private EditText editText_userName,editText_pwd; private CheckBox checkBox_pwd,checkBox_autoLogin; private SharedPreferences sp; private ImageButton imageButton_exit; private Button button_login; private String userName,pwd; private Button button_regist; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_login); //获得实例对象 sp = this.getSharedPreferences("userInfo", Context.MODE_PRIVATE); editText_userName = (EditText) findViewById(R.id.editText_userName); editText_pwd = (EditText) findViewById(R.id.editText_pwd); imageButton_exit = (ImageButton) findViewById(R.id.ib_exit); button_login = (Button) findViewById(R.id.button_login); checkBox_autoLogin = (CheckBox) findViewById(R.id.checkBox_autoLogin); checkBox_pwd = (CheckBox) findViewById(R.id.checkBox_pwd); button_regist = (Button) findViewById(R.id.button_regist1); //判断记住密码框状态 if(sp.getBoolean("ISCHECK",false)){//false是默认值 //设置默认是选择状态 checkBox_pwd.setChecked(true); userName = sp.getString("USER_NAME",""); pwd = sp.getString("PWD",""); editText_userName.setText(userName); editText_pwd.setText(pwd); //判断自动登录复选框状态 if(sp.getBoolean("AUTO_ISCHECK",false)){ //设置自动登录框状态 checkBox_autoLogin.setChecked(true); Intent intent = new Intent(LoginActivity.this,MainActivity.class); startActivity(intent); } } button_login.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { userName = editText_userName.getText().toString(); pwd = editText_pwd.getText().toString(); if(TextUtils.isEmpty(userName) || TextUtils.isEmpty(pwd)){//如果用户名或者密码为空 Toast.makeText(LoginActivity.this, "用户名或密码不能为空!", Toast.LENGTH_SHORT).show(); }else { if(userName.equals("liushilin") && pwd.equals("luochunyan")){ Toast.makeText(LoginActivity.this,"登录成功!正在获取用户信息...",Toast.LENGTH_SHORT).show(); //如果用户选择记住密码,则把用户信息存在xml文件中 if(checkBox_pwd.isChecked()){ SharedPreferences.Editor editor = sp.edit(); editor.putString("USER_NAME",userName); editor.putString("PWD",pwd); editor.commit(); } //主界面的跳转 Intent intent = new Intent(LoginActivity.this,MainActivity.class); // intent.putExtra("USER_NAME",userName); // intent.putExtra("PWD",pwd); User user = new User(); user.setUserName(userName); user.setPwd(pwd); intent.putExtra("USER",user); startActivity(intent); }else{ Toast.makeText(LoginActivity.this,"用户名或密码不正确,请重新输入!",Toast.LENGTH_SHORT).show(); } } } }); //监听记住密码复选框的按钮事件 checkBox_pwd.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() { @Override public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) { SharedPreferences.Editor editor = sp.edit(); if(checkBox_pwd.isChecked()){ editor.putBoolean("ISCHECK", true).commit(); }else{ editor.putBoolean("ISCHECK",false).commit(); } } }); //监听自动登录按钮事件 checkBox_autoLogin.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() { @Override public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) { if(checkBox_autoLogin.isChecked()){ sp.edit().putBoolean("AUTO_ISCHECK",true).commit(); }else { sp.edit().putBoolean("AUTO_ISCHECK",false).commit(); } } }); //监听退出按钮事件 imageButton_exit.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // finish(); ActivityCollector.finishAll();//退出当前应用程序 } }); button_regist.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { startActivity(new Intent(LoginActivity.this,RegistActivity.class)); } }); } }
package com.example.nanchen.listviewdemo; import android.content.Intent; import android.os.Bundle; import android.os.Handler; import android.os.Message; import android.view.View; import android.widget.AbsListView; import android.widget.AdapterView; import android.widget.ListView; import com.example.nanchen.listviewdemo.adapter.PersonAdapter; import com.example.nanchen.listviewdemo.adapter_content.Person; import java.util.ArrayList; import java.util.List; /** * 使用线程机制模拟下拉加载数据的ListView控件使用 */ public class MainActivity extends BaseActivity implements AbsListView.OnScrollListener{ private static final int DATA_UPDATE = 0x1; private ListView listView; private List<Person> personList = new ArrayList<>(); private PersonAdapter personAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); listView = (ListView) findViewById(R.id.listView); listView.setOnScrollListener(this); View footerView = getLayoutInflater().inflate(R.layout.loading_layout,null); listView.addFooterView(footerView); initData(); personAdapter = new PersonAdapter(this,R.layout.list_item,personList); listView.setAdapter(personAdapter); listView.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { // Toast.makeText(MainActivity.this,"你选择了"+personList.get(position).getName(),Toast.LENGTH_SHORT).show(); Intent intent = new Intent(MainActivity.this,ChatActivity.class); intent.putExtra("PERSON_NAME",personList.get(position).getName()); startActivity(intent); } }); } /** * 初始化数据 */ private void initData(){ Person person1 = new Person("刘世麟",R.mipmap.a_1); personList.add(person1); Person person2 = new Person("罗春燕",R.mipmap.a_2); personList.add(person2); Person person3 = new Person("王足英",R.mipmap.a_3); personList.add(person3); Person person4 = new Person("蔡何",R.mipmap.a_4); personList.add(person4); Person person5 = new Person("李兴",R.mipmap.a_5); personList.add(person5); Person person6 = new Person("李强",R.mipmap.a_6); personList.add(person6); Person person7 = new Person("李添顺",R.mipmap.a_7); personList.add(person7); Person person8 = new Person("晏贵秀",R.mipmap.a_8); personList.add(person8); Person person9 = new Person("周芳",R.mipmap.a_9); personList.add(person9); Person person10 = new Person("韩思明",R.mipmap.a_10); personList.add(person10); Person person11 = new Person("晏蜀杰",R.mipmap.a_11); personList.add(person11); Person person12 = new Person("马巍乃",R.mipmap.a_12); personList.add(person12); } private int visibleLastIndex;//用来可显示的最后一条数据的索引 @Override public void onScrollStateChanged(AbsListView view, int scrollState) { if(personAdapter.getCount() == visibleLastIndex && scrollState == SCROLL_STATE_IDLE){ new LoadDataThread().start(); } } @Override public void onScroll(AbsListView view, int firstVisibleItem, int visibleItemCount, int totalItemCount) { visibleLastIndex = firstVisibleItem + visibleItemCount - 1;//减去最后一个加载中那条 } /** * 线程间通信的机制 */ private Handler handler = new Handler(){ @Override public void handleMessage(Message msg) { super.handleMessage(msg); switch (msg.what){ case DATA_UPDATE: personAdapter.notifyDataSetChanged(); break; } } }; /** * 模拟加载数据的线程 */ class LoadDataThread extends Thread{ @Override public void run() { initData(); try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } handler.sendEmptyMessage(DATA_UPDATE);//通过handler发送一个更新数据的标记 } } }
package com.example.nanchen.listviewdemo; import android.content.DialogInterface; import android.content.Intent; import android.os.Bundle; import android.support.v7.app.AlertDialog; import android.view.View; import android.widget.Button; public class RegistActivity extends BaseActivity { private Button button_regist; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_regist); button_regist = (Button) findViewById(R.id.button_regist); button_regist.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { AlertDialog.Builder builder = new AlertDialog.Builder(RegistActivity.this); builder.setTitle("注册"); builder.setMessage("确认注册吗?"); builder.setPositiveButton("确定", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { startActivity(new Intent(RegistActivity.this, LoginActivity.class)); } }); builder.setNegativeButton("取消", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { startActivity(new Intent(RegistActivity.this, LoginActivity.class)); } }); builder.show(); } }); } }
package com.example.nanchen.listviewdemo; import android.content.Intent; import android.os.Bundle; import android.os.Handler; public class SplashActivity extends BaseActivity { // private static final int STATR_ACTIVITY = 0x1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_splash); // requestWindowFeature(Window.FEATURE_NO_TITLE); // handler.sendEmptyMessageDelayed(STATR_ACTIVITY,2000); new Handler().postDelayed(new Runnable() { @Override public void run() { startActivity(new Intent(SplashActivity.this,LoginActivity.class)); } },3000); } // private Handler handler = new Handler(){ // @Override // public void handleMessage(Message msg) { // super.handleMessage(msg); // switch(msg.what){ // case STATR_ACTIVITY: // startActivity(new Intent(SplashActivity.this,LoginActivity.class)); // break; // } // } // }; }
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="com.example.nanchen.listviewdemo.ChatActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="0dp" android:orientation="horizontal" android:layout_weight="1"> <Button android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:text="Back" android:textAllCaps="false" android:id="@+id/button_back"/> <TextView android:layout_width="0dp" android:layout_height="wrap_content" android:id="@+id/textView_person_name" android:layout_weight="4" android:gravity="center" /> <Button android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:text="+" android:textSize="18dp" android:id="@+id/button_add"/> </LinearLayout> <ListView android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="7" android:id="@+id/listView_msg"> </ListView> <LinearLayout android:layout_weight="1" android:layout_width="match_parent" android:orientation="horizontal" android:layout_height="0dp"> <EditText android:layout_width="0dp" android:layout_weight="3" android:id="@+id/editText_send" android:hint="请输入你想输入的话.." android:layout_height="wrap_content"/> <Button android:layout_width="0dp" android:layout_weight="1" android:text="发送" android:textSize="20dp" android:id="@+id/button_send" android:layout_height="wrap_content"/> </LinearLayout> </LinearLayout>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:background="@mipmap/logo_bg" tools:context=".LoginActivity" android:weightSum="1"> <RelativeLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="0.53" > <ImageButton android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/ib_exit" android:layout_alignParentRight="true" android:src="@mipmap/quit"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="用 户 名:" android:textSize="18dp" android:paddingTop="18dp" android:id="@+id/textView" android:layout_alignParentTop="true" android:layout_alignParentStart="true"/> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/editText_userName" android:hint="请输入用户名..." android:layout_below="@+id/textView" android:layout_alignParentStart="true" android:layout_alignEnd="@+id/ib_exit"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="密 码:" android:textSize="18dp" android:id="@+id/textView2" android:layout_below="@+id/editText_userName" android:layout_alignParentStart="true"/> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="textPassword" android:hint="请输入密码..." android:ems="10" android:id="@+id/editText_pwd" android:layout_below="@+id/textView2" android:layout_alignParentStart="true" android:layout_alignParentEnd="true"/> <CheckBox android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="记住密码" android:id="@+id/checkBox_pwd" android:checked="false" android:layout_centerVertical="true" android:layout_alignParentStart="true" android:layout_marginStart="27dp"/> <CheckBox android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="自动登录" android:id="@+id/checkBox_autoLogin" android:checked="false" android:layout_below="@+id/checkBox_pwd" android:layout_alignStart="@+id/checkBox_pwd"/> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="登录" android:id="@+id/button_login" android:layout_alignBottom="@+id/checkBox_autoLogin" android:layout_toEndOf="@+id/checkBox_pwd" android:layout_marginStart="64dp"/> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="注册" android:id="@+id/button_regist1" android:textAllCaps="false" android:layout_alignTop="@+id/button_login" android:layout_toEndOf="@+id/button_login"/> </RelativeLayout> </LinearLayout>
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@mipmap/login_bg" tools:context="com.example.nanchen.listviewdemo.MainActivity"> <ListView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/listView" android:dividerHeight="3.1dp" android:layout_alignParentTop="true" android:layout_alignParentStart="true" /> </RelativeLayout>
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:background="@mipmap/login_bg" tools:context="com.example.nanchen.listviewdemo.RegistActivity"> <LinearLayout android:orientation="horizontal" android:paddingTop="50dp" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="113dp" android:layout_alignParentTop="true" android:layout_alignParentStart="true"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="20dp" android:text="用户名:" android:id="@+id/textView"/> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/editText_name" android:layout_weight="1"/> </LinearLayout> <LinearLayout android:orientation="horizontal" android:paddingTop="100dp" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="113dp" android:layout_alignParentTop="true" android:layout_alignParentStart="true" android:id="@+id/linearLayout"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="20dp" android:text="密 码:" android:id="@+id/textView3"/> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/editText" android:layout_weight="1"/> </LinearLayout> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="完成注册" android:id="@+id/button_regist" android:layout_marginTop="42dp" android:layout_below="@+id/linearLayout" android:layout_centerHorizontal="true"/> </RelativeLayout>
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@mipmap/app_start3" tools:context="com.example.nanchen.listviewdemo.SplashActivity"> </RelativeLayout>
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:padding="6dp" android:layout_height="match_parent"> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/imageView_person"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="New Text" android:id="@+id/textView_name"/> </LinearLayout>
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <ProgressBar style="?android:attr/progressBarStyleSmall" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/progressBar"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="正在玩命加载中..." android:id="@+id/textView"/> </LinearLayout>
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:padding="6dp" android:orientation="vertical" android:layout_height="match_parent"> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/left_layout" android:layout_gravity="left" android:background="#c000dd"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:id="@+id/left_msg" android:layout_margin="10dp" android:textColor="#fff" /> </LinearLayout> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/right_layout" android:layout_gravity="right" android:background="#00e6ff"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/right_msg" android:layout_gravity="center" android:layout_margin="10dp"/> </LinearLayout> </LinearLayout>
作 者:
南 尘
出 处: http://www.cnblogs.com/liushilin/
关于作者:专注于移动前端的项目开发。如有问题或建议,请多多赐教!欢迎加入Android交流群:118116509
版权声明:本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文链接。
特此声明:所有评论和私信都会在第一时间回复。也欢迎园子的大大们指正错误,共同进步。或者直接私信我
声援博主:如果您觉得文章对您有帮助,可以点击文章下部【推荐】或侧边【关注】。您的鼓励是作者坚持原创和持续写作的最大动力!
欢迎关注我的公众号,精讲面试、算法、Andrid、Java、Python,旨在打造全网最比心的公众号。
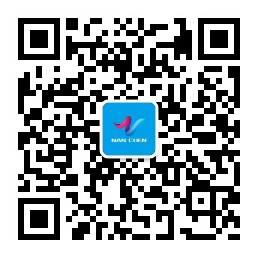