`
include
using namespace std;
const int MOD(1000000000 + 7);
const int Max_N(1000050);
namespace io
{
const int SIZE = (1 << 21) + 1;
char ibuf[SIZE], *iS, *iT, obuf[SIZE], *oS = obuf, *oT = oS + SIZE - 1, c, qu[55]; int f, qr;
#define gc() (iS == iT ? (iT = (iS = ibuf) + fread (ibuf, 1, SIZE, stdin), (iS == iT ? EOF : *iS ++)) : iS ++)
inline void flush (){fwrite (obuf, 1, oS - obuf, stdout);oS = obuf;}
inline void putc (char x){oS ++ = x;if (oS == oT) flush ();}
template
inline void gi (I &x) {for (f = 1, c = gc(); c < '0' || c > '9'; c = gc()) if (c == '-') f = -1;
for (x = 0; c <= '9' && c >= '0'; c = gc()) x = x * 10 + (c & 15); x *= f;}
template
inline void print (I x){
if (!x) putc ('0'); if (x < 0) putc ('-'), x = -x;while(x) qu[++ qr] = x % 10 + '0', x /= 10;while (qr) putc (qu[qr--]);}
struct Flusher_ {~Flusher_(){flush();}}io_flusher_;
}
using io::gi;
using io::putc;
using io::print;
constexpr int Add(int a, int b)
{
return a + b >= MOD ? a + b - MOD : a + b;
}
constexpr int Mult(int a, int b)
{
return a * 1LL * b % MOD;
}
void exgcd(int a, int b, int &x, int &y)
{
if (b == 0)
x = 1, y = 0;
else
exgcd(b, a % b, y, x), y -= x * (a / b);
}
inline int inverse(int a)
{
int invx, invy;
exgcd(a, MOD, invx, invy);
return (invx % MOD + MOD) % MOD;
}
int N, Head[Max_N], Next[Max_N], Size[Max_N], Fac[Max_N], Inv[Max_N], Ans;
inline void Add_Edge(int s, int t)
{
Next[t] = Head[s], Head[s] = t;
}
void dfs(int u)
{
Size[u] = 1;
for (int v = Head[u];v;v = Next[v])
dfs(v), Size[u] += Size[v];
Ans = Add(Ans, Mult(Inv[Size[u]], Fac[Size[u] - 1]));
}
int main()
{
gi(N);
for (int u = 2, fa;u <= N;++u)
gi(fa), Add_Edge(fa, u);
Fac[0] = 1;
for (int i = 1;i <= N;++i)
Fac[i] = Mult(Fac[i - 1], i);
Inv[N] = inverse(Fac[N]);
for (int i = N - 1;i >= 0;--i)
Inv[i] = Mult(Inv[i + 1], i + 1);
dfs(1);
print(Ans);
return 0;
}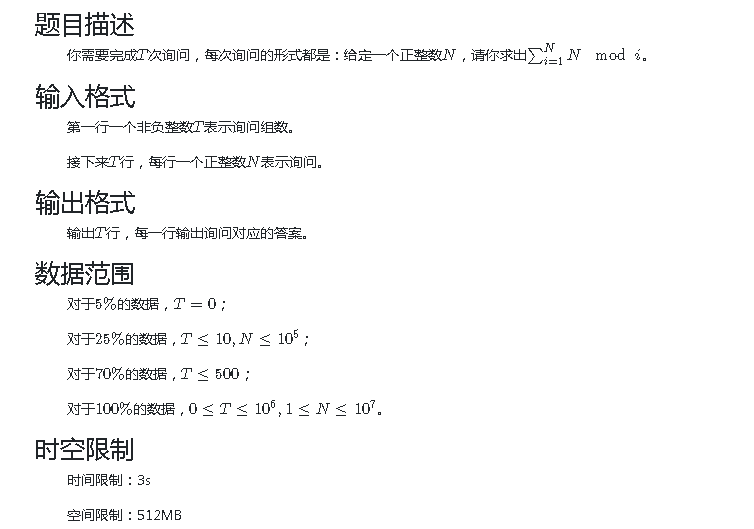
include
include
using namespace std;
const int Max_N(10000000);
typedef long long int LL;
bool Prime[Max_N + 5];
int ps, P[Max_N + 5];
LL mp1[Max_N + 5], d1[Max_N + 5], d1o[Max_N + 5], Pred1[Max_N + 5];
namespace io {
const int SIZE = (1 << 21) + 1;
char ibuf[SIZE], *iS, *iT, obuf[SIZE], *oS = obuf, *oT = oS + SIZE - 1, c, qu[55]; int f, qr;
// getchar
#define gc() (iS == iT ? (iT = (iS = ibuf) + fread (ibuf, 1, SIZE, stdin), (iS == iT ? EOF : *iS ++)) : *iS ++)
// print the remaining part
inline void flush () {
fwrite (obuf, 1, oS - obuf, stdout);
oS = obuf;
}
// putchar
inline void putc (char x) {
*oS ++ = x;
if (oS == oT) flush ();
}
// input a signed integer
template
inline void gi (I &x) {
for (f = 1, c = gc(); c < '0' || c > '9'; c = gc()) if (c == '-') f = -1;
for (x = 0; c <= '9' && c >= '0'; c = gc()) x = x * 10 + (c & 15); x *= f;
}
// print a signed integer
template
inline void print (I x) {
if (!x) putc ('0'); if (x < 0) putc ('-'), x = -x;
while (x) qu[++ qr] = x % 10 + '0', x /= 10;
while (qr) putc (qu[qr --]);
}
//no need to call flush at the end manually!
struct Flusher_ {~Flusher_(){flush();}}io_flusher_;
}
using io :: gi;
using io :: putc;
using io :: print;
int main()
{
memset(Prime, true, sizeof(Prime)), Prime[0] = Prime[1] = false;
mp1[1] = d1[1] = d1o[1] = 1LL;
for (int i = 2;i <= Max_N;++i)
{
if (Prime[i])
{
P[++ps] = i;
mp1[i] = i * 1LL, d1[i] = 1 + i * 1LL, d1o[i] = 1LL;
}
for (int j = 1, x;j <= ps && i * P[j] <= Max_N;++j)
{
Prime[x = i * P[j]] = false;
if (i % P[j])
{
mp1[x] = P[j];
d1[x] = d1[i] * d1[P[j]], d1o[x] = d1[i];
}
else
{
mp1[x] = mp1[i] * P[j];
d1[x] = d1[i] + d1o[i] * mp1[x], d1o[x] = d1o[i];
break;
}
}
}
for (int i = 1;i <= Max_N;++i)
Pred1[i] = Pred1[i - 1] + d1[i];
int T, N;
gi(T);
while (T--)
gi(N), print((N * 1LL) * (N * 1LL) - Pred1[N]), putc('\n');;
return 0;
}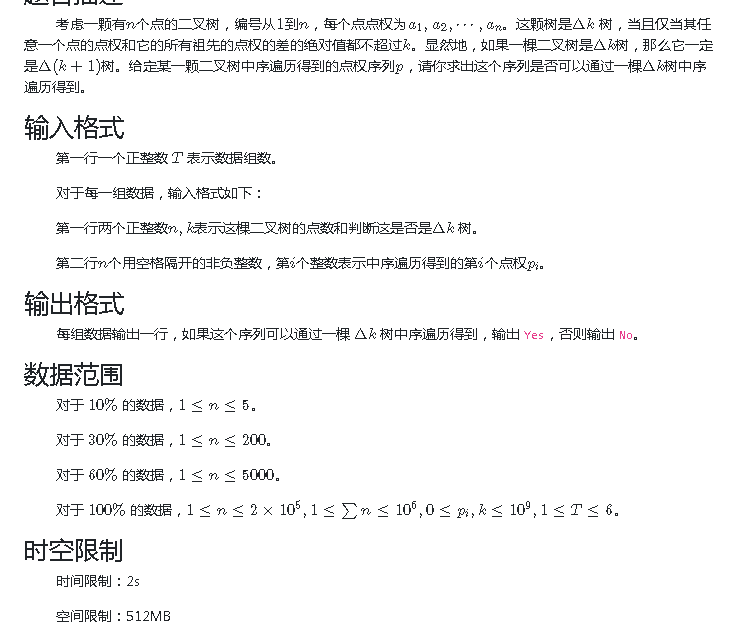
#include
include
const int maxN = 200005;
int N, K, A[maxN];
int STmin[18][maxN], STmax[18][maxN], Lg[maxN];
inline int getMin(int l, int r)
{
int d = Lg[r - l + 1];
return std::min(STmin[d][l], STmin[d][r - (1 << d) + 1]);
}
inline int getMax(int l, int r)
{
int d = Lg[r - l + 1];
return std::max(STmax[d][l], STmax[d][r - (1 << d) + 1]);
}
bool solve(int l, int r)
{
if (l >= r)
return true;
int mn = getMin(l, r), mx = getMax(l, r);
for (int i = 0; l + i <= r - i; ++i)
{
if (A[l + i] - K <= mn && A[l + i] + K >= mx)
return solve(l, l + i - 1) && solve(l + i + 1, r);
if (A[r - i] - K <= mn && A[r - i] + K >= mx)
return solve(l, r - i - 1) && solve(r - i + 1, r);
}
return false;
}
void work()
{
scanf("%d%d", &N, &K);
for (int i = 1; i <= N; ++i)
scanf("%d", A + i);
Lg[0] = -1;
for (int i = 1; i <= N; ++i)
{
Lg[i] = Lg[i >> 1] + 1;
STmin[0][i] = A[i], STmax[0][i] = A[i];
}
for (int i = 1; i <= Lg[N]; ++i)
for (int j = 1; j + (1 << i) - 1 <= N; ++j)
{
STmin[i][j] = std::min(STmin[i - 1][j], STmin[i - 1][j + (1 << i - 1)]);
STmax[i][j] = std::max(STmax[i - 1][j], STmax[i - 1][j + (1 << i - 1)]);
}
puts(solve(1, N) ? "Yes" : "No");
}
int main()
{
int T;
for (scanf("%d", &T); T--😉
work();
return 0;
}`