组件的注册
全局组件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="x-ua-compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Title</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.17/dist/vue.js"></script>
</head>
<body>
<div id="app1">
<!-- 全局组件是共用的 -->
<zjm></zjm>
</div>
<hr>
<div id="app2">
<!-- 多次调用 -->
<zjm></zjm>
<zjm></zjm>
</div>
<script>
// 全局组件 Vue.component("组件的名称",{template: ``})
Vue.component("zjm", {
template: `<div><h1>这是组件</h1></div>`,
});
const app = new Vue({ // 作用域1
el: "#app1",
})
const app2 = new Vue({ // 作用域2
el: "#app2"
})
</script>
</body>
</html>
局部注册
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <title>Document</title>
6 <script src="https://cdn.jsdelivr.net/npm/vue@2.5.17/dist/vue.js"></script>
7
8 </head>
9 <body>
10 <div id="app">
11 <duanyu></duanyu>
12 </div>
13 <script>
14 let xuyang = {
15 template:`<div>
16 <h1>{{name}}</h1>
17 <<button @click="my_click">点击</button>
18 </div>
19 `,
20 data:function(){
21 return{
22 name:"渣渣辉"
23 }
24 },
25 methods:{
26 my_click:function(){
27 alert(123)
28 }
29 }
30 };
31 const app = new Vue({
32 el:"#app",
33 components:{
34 duanyu:xuyang,
35 }
36 })
37
38 </script>
39 </body>
40 </html>
子组件注册
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta http-equiv="x-ua-compatible" content="IE=edge">
6 <meta name="viewport" content="width=device-width, initial-scale=1">
7 <title>Title</title>
8 <script src="https://cdn.jsdelivr.net/npm/vue@2.5.17/dist/vue.js"></script>
9 </head>
10 <body>
11 <div id="app">
12 <father></father>
13 </div>
14 <script>
15 let zzj = {
16 template: `<div><h2>这是子组件</h2></div>`,
17 };
18 let fzj = {
19 template: `<div>
20 <h1>这是父组件</h1>
21 <child></child>
22 </div>`,
23 components: {
24 child: zzj, // 注册子组件
25 }
26 };
27 const app = new Vue({
28 el: "#app",
29 components: {
30 father: fzj // 注册父组件
31 }
32 })
33 </script>
34 </body>
35 </html>
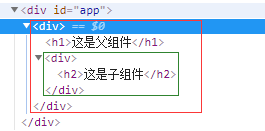
组件的通信
父子通信
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <title>Document</title>
6 <script src="https://cdn.jsdelivr.net/npm/vue@2.5.17/dist/vue.js"></script>
7 </head>
8 <body>
9 <div id="app">
10 <father></father>
11 </div>
12 <script>
13 let child = {
14 template:`<div>
15 <h2>这是子组件</h2>
16 <p>父亲给了{{money}}RMB</p>
17 </div>`,
18 props:['money']
19 };
20 let father = {
21 template:`<div>
22 <h1>这是父组件</h1>
23 <child :money="num"></child>
24 </div>`,
25 data:function(){
26 return{
27 num:100,
28 }
29 },
30 components:{
31 child:child
32 }
33 };
34
35 const app = new Vue({
36 el:"#app",
37 components:{
38 father:father
39 }
40 })
41 </script>
42 </body>
43 </html>
子父通信
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta http-equiv="x-ua-compatible" content="IE=edge">
6 <meta name="viewport" content="width=device-width, initial-scale=1">
7 <title>Title</title>
8 <script src="https://cdn.jsdelivr.net/npm/vue@2.5.17/dist/vue.js"></script>
9 </head>
10 <body>
11 <div id="app">
12 <father></father>
13 </div>
14 <script>
15 let child = {
16 template: `<div>
17 <h2>这是子组件</h2>
18 <button @click="my_click">点我给我父亲发信息</button>
19 </div>`,
20
21 methods: {
22 my_click: function () {
23 // 提交事件
24 this.$emit("son_say", "爸~你要当爷爷了~~");
25 }
26 }
27 };
28 let father = {
29 template: `<div>
30 <h1>这是父组件</h1>
31 <!--// 接收这个事件-->
32 <child @son_say="my_son_say"></child>
33 <p>老婆~~你儿子说~~{{mes}}</p>
34 </div>`,
35 components: {
36 child: child
37 },
38 data(){
39 return {
40 mes: ""
41 }
42 },
43 methods: {
44 my_son_say: function (data) {
45 console.log(data)
46 this.mes = data;
47 }
48 }
49 };
50
51 const app = new Vue({
52 el: "#app",
53 components: {
54 father: father
55 }
56 })
57 </script>
58 </body>
59 </html>
非父子通信
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <title>Document</title>
6 <script src="https://cdn.jsdelivr.net/npm/vue@2.5.17/dist/vue.js"></script>
7 </head>
8 <body>
9 <div id="app">
10 <gouxiaoke></gouxiaoke>
11 <sunpengfei></sunpengfei>
12 </div>
13 <script>
14
15 let xiaoxiong = new Vue();
16 let gouxiaoke = {
17 template:`<div>
18 <h1>这是刘小科</h1>
19 <button @click="my_click">点我给老孙发消息</button>
20 </div>`,
21 methods:{
22 my_click:function(){
23 //给中间调度器提交事件
24 //alert(123)
25 xiaoxiong.$emit("tonight","今晚洗白白")
26 }
27 }
28 };
29 let sunpengfei = {
30 template : `<div>
31 <h1>这是老孙</h1>
32 <p>刘小科跟我说{{say}}</p>
33 </div>`,
34 data(){
35 return{
36 say:""
37 }
38 },
39 //组件加载完成后去做的一些事情
40 mounted(){
41 let that = this;
42 xiaoxiong.$on("tonight",function(data){
43 console.log(data)
44 console.log(this)
45 that.say = data;
46 })
47 }
48
49 };
50
51 const app = new Vue({
52 el:"#app",
53 components:{
54 gouxiaoke:gouxiaoke,
55 sunpengfei:sunpengfei,
56 }
57 })
58
59 </script>
60
61 </body>
62 </html>