rest_framework认证组件 [ BaseAuthentication ]及全局使用/局部使用/局部禁用,rest_framework权限组件[ BasePermission ]及全局使用/局部使用/局部禁用,rest_framework自带的频率组件[ SimpleRateThrottle ]全局使用和配置/局部使用/局部禁用,rest_framework自定义频率组件
rest_framework认证组件 [ BaseAuthentication ]
from rest_framework.authentication import BaseAuthentication from rest_framework.exceptions import AuthenticationFailed from app01 import models # 登陆认证 class Loign_auth(BaseAuthentication): # 写一个类继承BaseAuthentication def authenticate(self, request): # 函数名字不能变,从继承的BaseAuthentication类的找函数名 token = request.META.get("HTTP_TOKEN") # 请求头里的数据在 key 前加 HTTP ret = models.User_Token.objects.filter(token=token).first() # 验证token if ret: # 认证通过 return ret.user,ret # 返回两个数据,这样以后的用户在request.user里 else: print("认证失败") raise AuthenticationFailed("认证失败") # 认证失败抛rest_framework认证失败的错误信息
rest_framework认证组件全局使用
# settings里认证组件全局控制 REST_FRAMEWORK = { # key值固定不变 : value 为列表,里面的元素为自己的写的认证组件 "DEFAULT_AUTHENTICATION_CLASSES": ["app01.check_model.Login_auth", ], # 认证组件全局使用(控制)
}
rest_framework认证组件局部使用
class Books(APIView): # authentication_classes 变量名不能变 = [ "认证组件类的名字",] authentication_classes = [ Authentication ] # 哪里需要认证写上这一行 def get(self, request): return Response("您已经登陆了")
rest_framework认证组件局部禁用
class Books(APIView): # authentication_classes 变量名不能变 = [ ] # 等于空列表 authentication_classes = [ ] # 哪里需要禁用写上这一行,列表为空 def get(self, request): return Response("您已经登陆了")
rest_framework权限组件 (权限认证一定要注册 rest_framework APP模块)
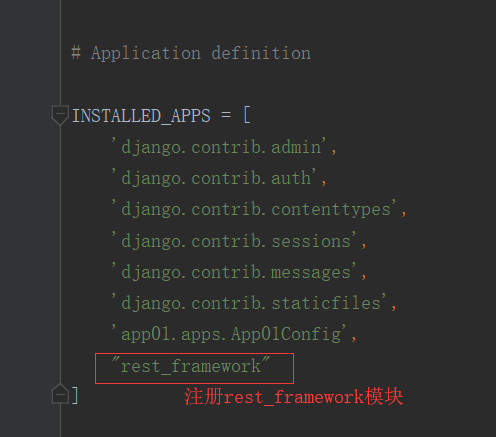
权限组件
from rest_framework.permissions import BasePermission class User_Permisson(BasePermission): # 写一个类继承 BasePermission def has_permission(self, request, view): # 函数名字不能变,从继承的BasePermission类里找函数名 if request.user.type == 3: # 权限开始认证,认证组件通过已将用户赋值给 request.user print("权限认证通过") return True # 权限认证通过返回True else: print("权限认证失败") return False # 权限认证没通过返回False
rest_framework权限组件全局使用
REST_FRAMEWORK = { # key值固定不变 : value 为列表,里面的元素为自己的写的认证组件 "DEFAULT_AUTHENTICATION_CLASSES": ["app01.check_module.Login_auth"], # 认证组件全局配置(使用) "DEFAULT_PERMISSION_CLASSES": ["app01.check_module.User_Permisson"], # 权限组件全局配置(使用) }
rest_framework权限组件局部使用
class Books(APIView): # 认证组件局部使用 变量名不能变 # authentication_classes = [Login_auth,] # 权限组件局部使用 permission_classes 变量名不能变 permission_classes = [User_Permisson, ] # 哪里需要认证写上这一行,认证组件通过,在走权限组件 def get(self, request): return Response("OK")
rest_framework权限组件局部禁用
rest_framework权限组件局部禁用 class Books(APIView): # permission_classes变量名不能变 = [] 列表为空表示权限组件禁用 permission_classes = [] # 哪里需要权限认证写上这一行 [ 认证组件通过,在走权限组件 ] def get(self, request): return Response("OK")
rest_framework自带的频率组件
class User_throttle(SimpleRateThrottle): # 写一个类继承 BasePermission scope = "login_path" # 这个名字随便写,全局配置信息根据scope来设置频率次数 3/m def get_cache_key(self, request, view): # 函数名字不能变,从继承的BasePermission类的找函数名 print(request.META) request.META.get("PATH_INFO") return request.path # 限制什么频率,就返回什么,比如这里限制访问路径
rest_framework频率组件全局使用和配置
REST_FRAMEWORK = { "DEFAULT_THROTTLE_CLASSES": ["app01.check_module.User_throttle"], # 频率组件全局使用 "DEFAULT_THROTTLE_RATES": { # "scope变量名":m/n eg: m/n为{'s': 1, 'm': 60, 'h': 3600, 'd': 86400}里的任一元素 "login_path": "3/m", # 频率组件全局配置 key:value key为自定义频率组件里的scope变量,value为{'s': 1, 'm': 60, 'h': 3600, 'd': 86400}里的任一元素 } }
rest_framework频率组件局部使用
class Books(APIView):
# 权限组件局部使用throttle_classes变量名不能变
throttle_classes = [User_throttle,] # 频率组件局部使用,哪里需要频率校验写上这一行 [ 认证组件通过,在走频率组件 ]
def get(self, request):
return Response("OK")
rest_framework频率组件局部禁用
class Books(APIView): # throttle_classes变量名不能变 = [] 空列表表示权限组件禁用 throttle_classes = [] # 频率组件局部禁用
def get(self, request): return Response("OK")
from rest_framework.exceptions import Throttled
# 错误信息显示成中文 def throttled(self, request, wait): class MyThrottled(exceptions.Throttled): default_detail = '傻逼' extra_detail_singular = '还剩 {wait} 秒.' extra_detail_plural = '还剩 {wait} 秒' raise MyThrottled(wait)
rest_framework自定义频率组件
from rest_framework.throttling import BaseThrottle, SimpleRateThrottle import time # 设置一分钟只能访问三次 class MyThrottle(BaseThrottle): visitor_dic = {} def __init__(self): self.history = None def allow_request(self, request, view): # META:请求所有的东西的字典 # 拿出ip地址 ip = request.META.get('REMOTE_ADDR') ctime = time.time() # 判断ip在不在字典里,不再说明是第一次访问,往字典里添加时间 if ip not in self.visitor_dic: self.visitor_dic[ip] = [ctime, ] return True # ip在字典里,取出ip对应的访问时间列表 history = self.visitor_dic[ip] self.history = history # 当字典里有值和列表里当前时间减去第一次的时间差大于60s,超过了一分钟就不让访问, # 则把最后一次时间删掉 while history and ctime - history[-1] > 60: history.pop() # 判断列表里时间元素,是不是大于3,,大于3直接False if len(history) < 3: # 把当前时间放在列表第0位 history.insert(0, ctime) return True # 大于3直接False return False
# 设置等待时间 def wait(self): ctime = time.time() # ctime - self.history[-1] 表示当前时间超了多长时间 print(self.history[-1]) # 当前时间减去第一次访问时间 return 60 - (ctime - self.history[-1])
# 错误信息显示成中文 def throttled(self, request, wait): class MyThrottled(exceptions.Throttled): default_detail = '傻逼' extra_detail_singular = '还剩 {wait} 秒.' extra_detail_plural = '还剩 {wait} 秒' raise MyThrottled(wait)