http://blog.csdn.net/cheyo/article/details/6595955
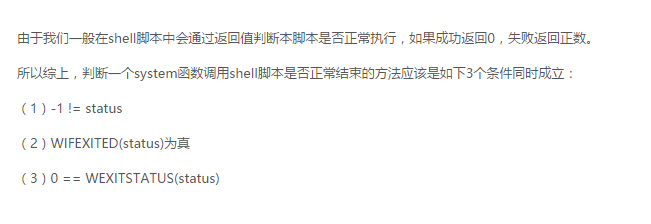
1 #include <stdio.h> 2 #include <stdlib.h> 3 #include <sys/wait.h> 4 #include <sys/types.h> 5 6 int main() 7 { 8 pid_t status; 9 10 status = system("./test.sh"); 11 if (-1 == status) 12 { 13 printf("system error!"); 14 } 15 else 16 { 17 printf("exit status value = [0x%x]\n", status); 18 if (WIFEXITED(status)) 19 { 20 if (0 == WEXITSTATUS(status)) 21 { 22 printf("run shell script successfully.\n"); 23 } 24 else 25 { 26 printf("run shell script fail, script exit code: %d\n", WEXITSTATUS(status)); 27 } 28 } 29 else 30 { 31 printf("exit status = [%d]\n", WEXITSTATUS(status)); 32 } 33 } 34 35 return 0; 36 } 37 38 status