1、x 的平方根
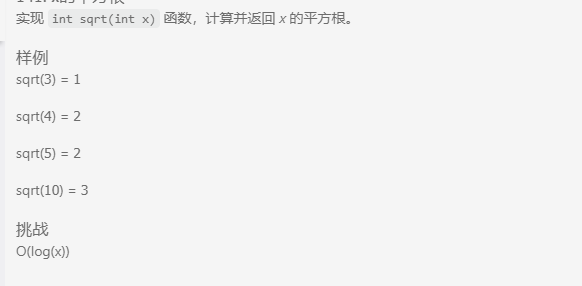
class Solution:
"""
@param x: An integer
@return: The sqrt of x
"""
def sqrt(self, x):
# write your code here
left, right = 0, 100000
while left < right:
mid = (left + right) // 2
if mid * mid == x:
return mid
elif mid * mid < x:
left = mid + 1
else:
right = mid - 1
if left * left > x:
return left-1
return left
2、搜索插入位置
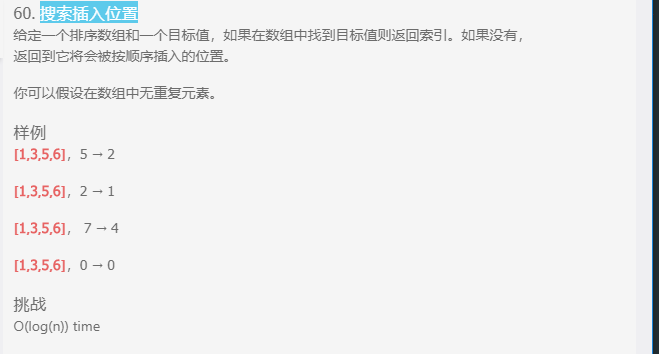
class Solution:
"""
@param A: an integer sorted array
@param target: an integer to be inserted
@return: An integer
"""
def searchInsert(self, A, target):
# write your code here
if not A: return 0
left, right = 0, len(A) - 1
while left != right:
mid = (left + right) // 2
if A[mid] == target:
return mid
elif A[mid] < target:
left = mid +1
else:
right = mid
if A[left] < target:
return left + 1
else:
return left
3、搜索二维矩阵
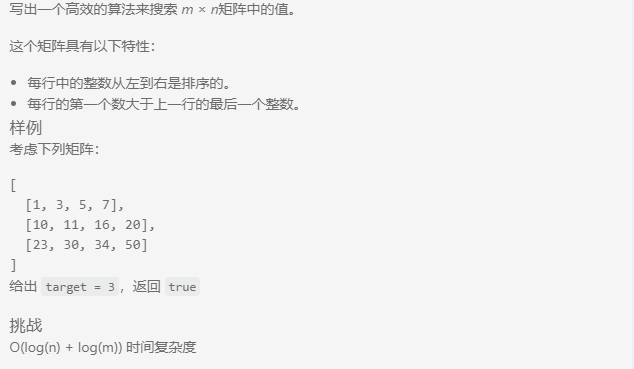
class Solution:
"""
@param matrix: matrix, a list of lists of integers
@param target: An integer
@return: a boolean, indicate whether matrix contains target
"""
def searchMatrix(self, matrix, target):
# write your code here
if not matrix:return False
m = len(matrix)
n = len(matrix[0])
left, right = 0, m * n - 1
while left <= right:
mid = (left + right) // 2
if matrix[mid // n][mid % n] == target:
return True
elif matrix[mid // n][mid % n] < target:
left = mid + 1
else:
right -= 1
return False
4、二分查找
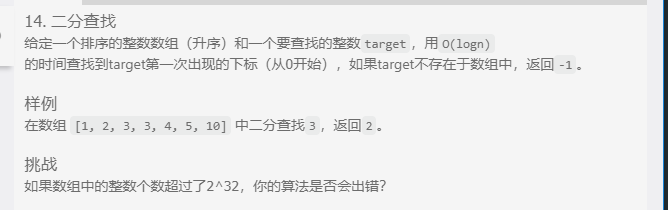
class Solution:
"""
@param nums: The integer array.
@param target: Target to find.
@return: The first position of target. Position starts from 0.
"""
def binarySearch(self, nums, target):
# write your code here
left, right = 0, len(nums) - 1
while left <= right:
mid = (left + right) // 2
if nums[mid] == target:
if mid == 0 or nums[mid - 1] != target:
return mid
else:
right = mid
elif nums[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
5、寻找旋转排序数组中的最小值
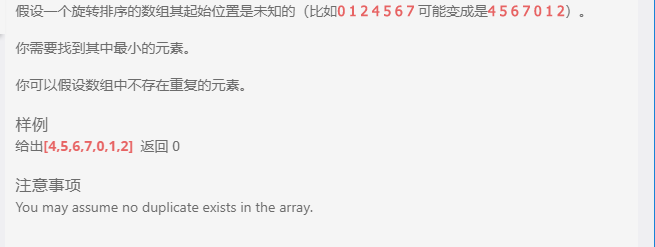
思路:一样左右向中间用二分法,每次都保证左边数值大于右边的数值,当没法保证的时候,就是边界了。
class Solution:
"""
@param nums: a rotated sorted array
@return: the minimum number in the array
"""
def findMin(self, nums):
# write your code here
if len(nums) == 1: return nums[0]
left, right = 0, len(nums) - 1
while right - left > 1:
if nums[left] < nums[right]: return nums[left]
mid = (left + right) // 2
if nums[mid] > nums[left]:
left = mid
else:
right = mid
return min(nums[left], nums[right])
6、木材加工
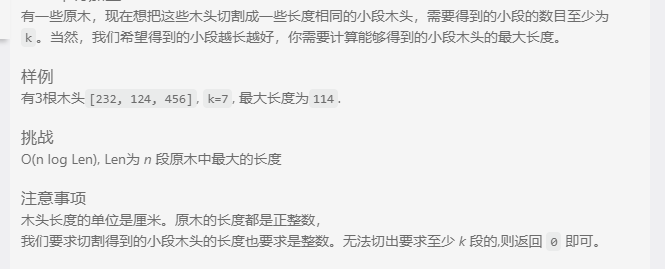
思路:二分法想不出来,哎。只用用暴力解法。
class Solution:
"""
@param L: Given n pieces of wood with length L[i]
@param k: An integer
@return: The maximum length of the small pieces
"""
def woodCut(self, l, k):
# write your code here
if not l:return 0
max_len = sum(l) // k
while max_len>0:
now_k = 0
for i in l:
now_k += i // max_len
if now_k >= k:
return max_len
max_len -= 1
return 0