目录
1、安装
# 全局安装
npm i -g jsdoc
# 本地安装
npm i -D jsdoc
2、基本使用
/**
* 这是一个变量的声明
* @type {number}
*/
var sampleVariable = 123
/**
* 这是一个函数示例
* @param {string} arg1 - 第一个参数,字符串类型
* @param {number} arg2 - 第二个参数,数字类型
* @return {boolean} 函数返回值,布尔类型
*/
function sampleFunction(arg1, arg2) {
return true
}
/**
* 这是一个自定义类型的声明
* @typedef {Object} Person
* @property {string} name - 用户姓名
* @property {number} age - 用户年龄
* @property {boolean} isStudent - 用户是否学生
* @property {string[]} courses - 用户注册的课程
*/
/**
* @param {Person} person - greet函数传递的参数
*/
function greet(person) {
console.log(`Hello, ${person.name}!`);
}
/**
* 这是一个类的声明
* @class
* @classdesc 这是类的描述信息
*/
class SampleClass {
constructor() {
}
}
/**
* 这是一个构造函数的声明
* @constructor
* @param {string} arg1 - 第一个参数,字符串类型
* @param {number} arg2 - 第二个参数,数字类型
*/
function SampleConstructor(arg1, arg2) {
}
3、生成文档
# 单文件
jsdoc ./index.js
# 指定目录
jsdoc ./src
# jsdoc --help
# -d或--destination:文档生成的目录
# -c或--configure:指定配置文件路径
# -t或--template:使用的模板
# -u或--tutorials:指定教程文件路径
# -p或--package:指定package.json文件路径
# -R或--readme:指定README文件路径
# -r或--recurse:是否递归处理子目录
4、自定义配置生成文档
- 步骤
# 生成package.json
npm init -y
# 安装jsdoc
npm i -D jsdoc
# 项目根目录新建jsdoc.config.json(jsdoc官方文档:https://jsdoc.app/)
# package.json添加
- jsdoc.config.json
{
"source": {
"include": [
"./src/"
],
"includePattern": ".+\\.js(doc)?$",
"exclude": [
"./docs"
],
"excludePattern": "(node_modules|test|dist|coverage|^|\\/|\\\\)_"
},
"opts": {
"encoding": "utf8",
"destination": "./docs/",
"recurse": true
},
"plugins": [
"plugins/markdown"
],
"templates": {
"cleverLinks": false,
"monospaceLinks": false
},
"metadata": {
"title": "我的Javascript项目"
},
"tags": {
"allowUnknownTags": true,
"dictionaries": [
"jsdoc",
"closure"
]
}
}
- package.json
{
"scripts": {
"docs": "jsdoc -c jsdoc.config.json"
}
}
5、主题模板应用
- 步骤
# 列举几款优秀的模板,可一同安装并逐一测试
npm i -D taffydb
npm i -D better-docs
npm i -D clean-jsdoc-theme
npm i -D daybrush-jsdoc-template
npm i -D docdash
# jsdoc.config.json添加和修改
# 修改模板的特定自定义属性
- jsdoc.config.json
{
"opts": {
"template": "node_modules/clean-jsdoc-theme",
"theme_opts": {
"default_theme": "light",
"homepageTitle": "Clean JSDoc theme",
"title": "JSDoc文档自动生成",
"footer": "copyright by Vane.",
"menu": [
{
"title": "尚硅谷",
"link": "http://www.atguigu.cn",
"target": "_blank"
}
]
}
}
}
6、自定义主题模板
- 步骤
# 复制默认模板文件(node_modules/jsdoc/templates/default)至项目根目录下
# jsdoc.config.json修改(删除opts.theme_opts属性,修改opts.template属性)
- jsdoc.config.json
{
"opts": {
"template": "./default"
}
}
7、markdown插件的应用
- 步骤
# 编辑测试用js注释
# jsdoc.config.json添加
- src/function.js
/**
* 这是一个函数示例
*
* # Markdown的1号标题
* - Markdown的无序列表
* - Markdown的无序列表
* - Markdown的无序列表
* - [Markdown的链接](http://www.atguigu.cn)
* - Markdown的图片 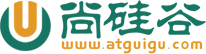
*
* @param {string} arg1 - 第一个参数,字符串类型
* @param {number} arg2 - 第二个参数,数字类型
* @return {boolean} 函数返回值,布尔类型
*/
function sampleFunction(arg1, arg2) {
return true
}
- jsdoc.config.json
{
"plugins": [
"plugins/markdown"
]
}
8、eslint检查注释语法
- 步骤
# 安装eslint
npm i -D eslint
# 项目根目录新建.eslintrc.js(webstorm需设置Automatic ESLint configuration。此时js有语法检查,但是js注释没有)
# 安装eslint-plugin-jsdoc
npm i -D eslint-plugin-jsdoc
# .eslintrc.js添加(添加extends、plugins属性,修改rules属性)(报ESLint: SyntaxError: xxx可提高nodejs版本)
# eslint自动修复命令
eslint --fix ./src
- .eslintrc.js
module.exports = {
extends: ['eslint:recommended'],
plugins: ['jsdoc'],
rules: {
'no-undef': 'error',
'no-unused-vars': 'error',
// js注释的检查规范
'jsdoc/newline-after-description': 1,
'jsdoc/require-param': 1,
'jsdoc/require-param-description': 1,
'jsdoc/require-param-name': 1,
'jsdoc/require-param-type': 1,
'jsdoc/require-property': 1,
'jsdoc/require-property-description': 1,
'jsdoc/require-property-name': 1,
'jsdoc/require-property-type': 1,
'jsdoc/require-returns': 1,
'jsdoc/require-returns-check': 1
}
}
9、prettier规范注释语法
- 步骤
# 安装
npm i -D prettier prettier-plugin-jsdoc
# 项目根目录新建.prettierrc.js
# prettier格式化命令
prettier --write "src/*/*.js"
# 格式化注释自定义配置
- .prettierrc.js
module.exports = {
plugins: ['prettier-plugin-jsdoc'],
jsdocSpaces: 2,
jsdocDescriptionWithDot: true,
jsdocDescriptionTag: true,
jsdocVerticalAlignment: true,
jsdocKeepUnParseAbleExampleIndent: true,
jsdocSeparateReturnsFromParam: true,
jsdocSeparateTagGroups: true,
jsdocPreferCodeFences: true
}
10、实现静态类型检测
- src/index.js
// @ts-check
// 项目根目录新建tsconfig.json(需配置allowJs: true,否则@ts-check不生效)
/**
* @description Name
* @type {string}
*/
const name = 123
/**
* @description Number
* @type {number}
*/
const number = "100"
/**
* @description Array
* @type {number[]}
*/
const myArray = [10, 132.12, 100, "Hi", true]
// jsdoc输出文档时控制台会报错,原因是对象的数据类型定义使用了复杂的表达式,需将对象的类型注释拆解开来
// /**
// * @description Object
// * @type {{
// * readonly id: number,
// * name: string,
// * age: number | string,
// * address?: string
// * }}
// */
// const user = {
// id: 1,
// name: "Vane",
// age: "44",
// address: "上海"
// }
// user.id = 2
/** @constant */
const user = {
/** @type {number} */
/** @readonly */
id: 1,
/** @type {string} */
name: "Vane",
/** @type {string | number} */
age: "44",
/** @type {string | null} */
address: "上海"
}
user.id = 2
/**
* @description Calculate age
* @param {number} current Current year
* @param {number} yearOfBirth Year of birth
* @return {string} Age
*/
const calculateAge = (current, yearOfBirth) => {
return `${current - yearOfBirth}`
}
console.log(calculateAge("2021", 2019))
/**
* @typedef {Object} User
* @property {number} id
* @property {string} name
* @property {number | string} age
* @property {boolean} [isMale]
*/
/** @type {User} */
const man = {
id: 1,
name: "Vane",
age: 44,
isMale: 1
}
- tsconfig.json
{
"compilerOptions": {
"module": "commonjs",
"target": "es5",
"sourceMap": true,
"allowJs": true
},
"exclude": [
"node_modules"
]
}