多线程(2)-线程同步互斥锁Mutex
在 Linux 多线程编程中,互斥锁(Mutex)是一种常用的同步机制,用于保护共享资源,防止多个线程同时访问导致的竞争条件。在 POSIX 线程库中,互斥锁通常通过 pthread_mutex_t 类型表示,相关的函数包括 pthread_mutex_init、pthread_mutex_lock、pthread_mutex_unlock 等。
下面为一个demo,举例说明在多线程中使用互斥锁:
#include <stdio.h> #include <pthread.h> #define NUM_THREADS 5 // 共享资源 int shared_resource = 0; // 互斥锁 pthread_mutex_t mutex; // 线程函数 void *thread_function(void *arg) { int thread_id = *((int *)arg); // 加锁 pthread_mutex_lock(&mutex); // 访问共享资源 printf("Thread %d: Shared resource before modification: %d\n", thread_id, shared_resource); sleep(1); shared_resource++; printf("Thread %d: Shared resource after modification: %d\n", thread_id, shared_resource); // 解锁 pthread_mutex_unlock(&mutex); pthread_exit(NULL); } int main() { pthread_t threads[NUM_THREADS]; int thread_args[NUM_THREADS]; int i; // 初始化互斥锁 pthread_mutex_init(&mutex, NULL); // 创建多个线程 for (i = 0; i < NUM_THREADS; ++i) { thread_args[i] = i; pthread_create(&threads[i], NULL, thread_function, (void *)&thread_args[i]); } // 等待所有线程完成 for (i = 0; i < NUM_THREADS; ++i) { pthread_join(threads[i], NULL); } // 销毁互斥锁 pthread_mutex_destroy(&mutex); return 0; }
执行结果如下:
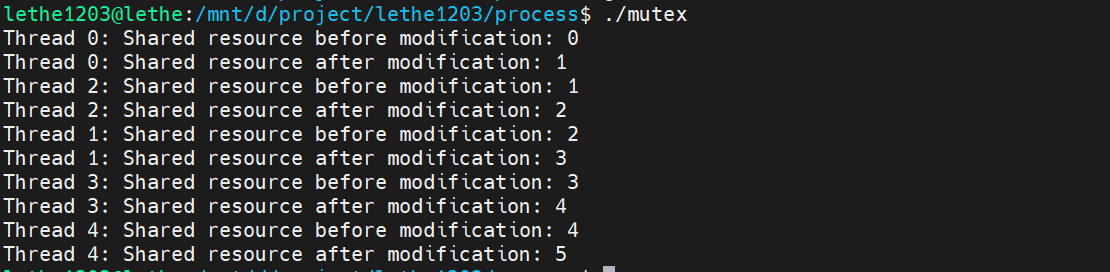