from fastapi import FastAPI, Body
from pydantic import BaseModel
app = FastAPI()
'''
使用Body接收请求体数据
{
"user": {
"username": "Tom",
"password": "123465789"
},
"item": {
"name": "book",
"description": "python",
"price": 42.0,
"tax": 3.2
},
"importance":5
}
'''
class User(BaseModel):
username: str
password: str
class Item(BaseModel):
name: str
description: str
price: float
tax: float
@app.post("/login")
def login(user: User, item: Item, importance: int = Body()):
return {"user": user,
"item": item,
"importance": importance
}
'''
# 通过 Body(embed=True) 获取嵌套请求体
{
"item": {
"name": "book",
"description": "python",
"price": 42.0,
"tax": 3.2
}
}
'''
@app.post("/items")
def items(item : Item = Body(embed=True)):
return item
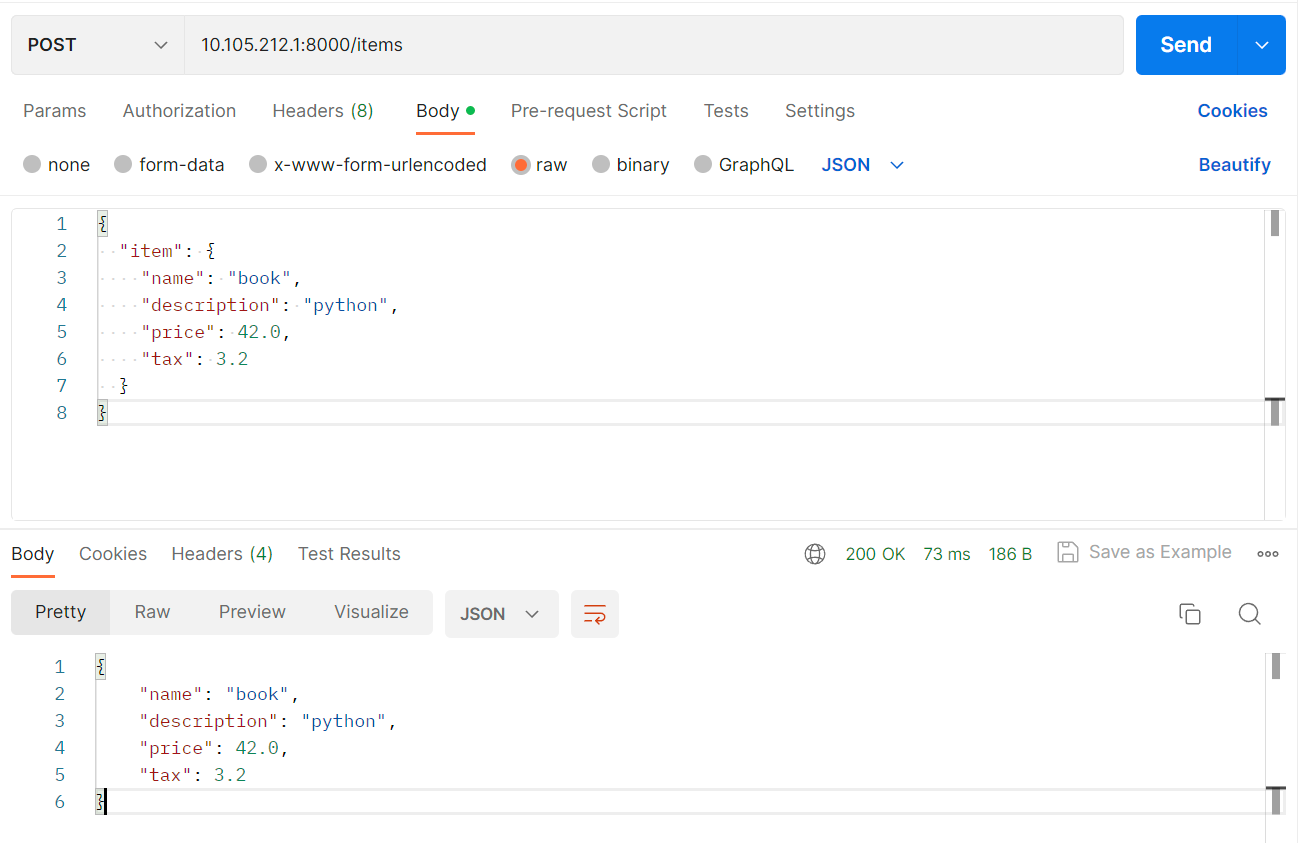