实训作业4
public class Person {
private String name;
private int age;
// 无参构造方法
public Person(){
}
//初始化姓名
public Person(String name){
this.name=name;
}
//初始化年龄
public Person(int age){
this.age=age;
}
//初始化姓名和年龄
public Person(String name,int age){
this.name=name;
this.age=age;
}
//获取年龄
public String getName(){
return name;
}
//设置姓名
public void setName(String name){
this.name=name;
}
//获取年龄
public int getAge(){
return age;
}
//设置年龄
public void setAge(int age){
if(age<0){ //年龄不能为负
this.age=0;
}
else{
this.age=age;
}
}
//打印信息
public void printMessage(){
System.out.println("name="+name+",age="+age);
}
//过了一年年龄加一
public void addAge(){
age++;
}
}
测试
3.1 电费
private int count2;
public Cost1(){
}
public Cost1(int count1){
this.count1=count1;
}
public Cost1(int count2){
this.count2=count2;
}
public Cost1(int count1,int count2){
this.count1=count1;
this.count2=count2;
}
public int getCount1(){
return count1;
}
public void setCount1(int count1){
this.count1=count1;
}
public int getCount2(){
return count2;
}
public void setCount2(int count2){
this.count2=count2;
}
public void printMessage(){
System.out.println("count1="+count1+",count2="+count2);}
double money(double money1,int count2){
money1=money1*count2;
System.out.println("电费为:"+money1);
}
}
Cost1 c1=new Cost1(1000,1200);
c1.getCount1();
c1.printMessage();
double money1 = 1.2;
Cost1 c2=new Cost1(1200,1500);
c2.getCount2();
c2.printMessage();
Person p3=new Person();
}
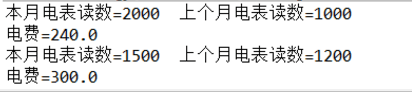
public static void main(String[] args) {
// TODO Auto-generated method stub
Person p1=new Person("小明",8);
p1.addAge();
p1.printMessage();
Person p2=new Person("小红");
p2.setAge(20);
p2.addAge();
p2.printMessage();
Person p3=new Person();
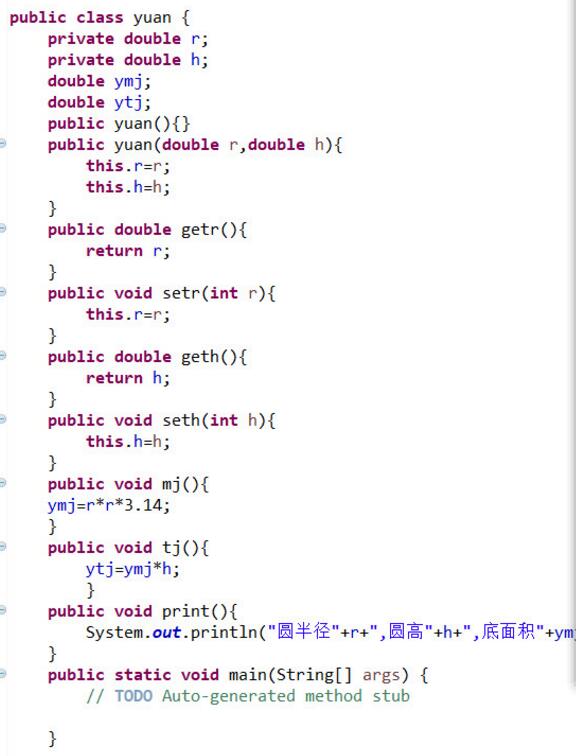
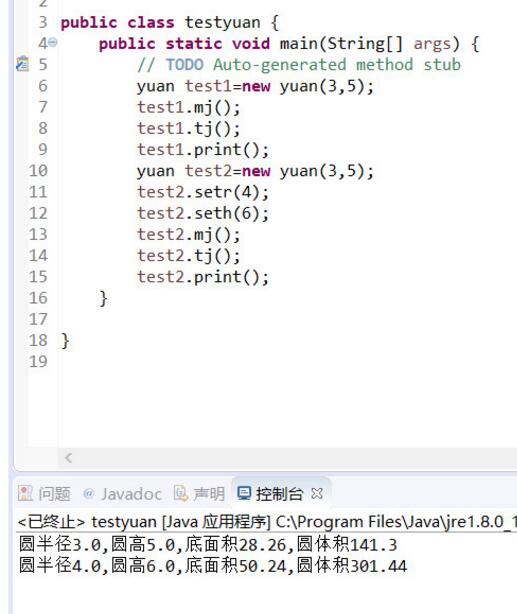
4、编写“四则运算类”及其测试类。
4.1 应用场景
- 计算器。能实现简单的四则运算,要求:只进行一次运算。
4.1 “四则运算”类
- 私有属性:操作数一、操作数二、操作符
- 构造方法:带两个参数
- 构造方法:带三个参数
- 方法一:对两个操作数做加运算
- 方法二:对两个操作数做减运算
- 方法三:对两个操作数做乘运算
- 方法四:对两个操作数做除运算
4.2 测试类
- 从键盘输入两个操作数和一个操作符,计算之后,输出运算结果。
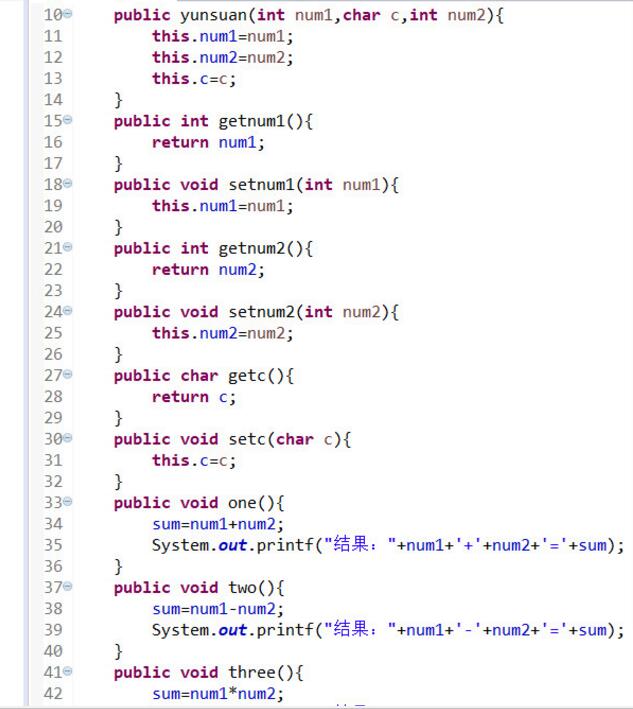
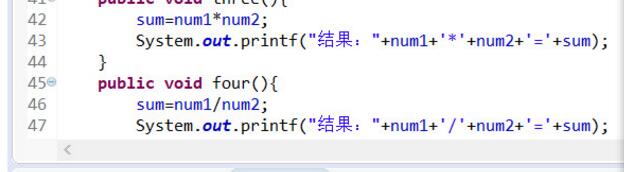
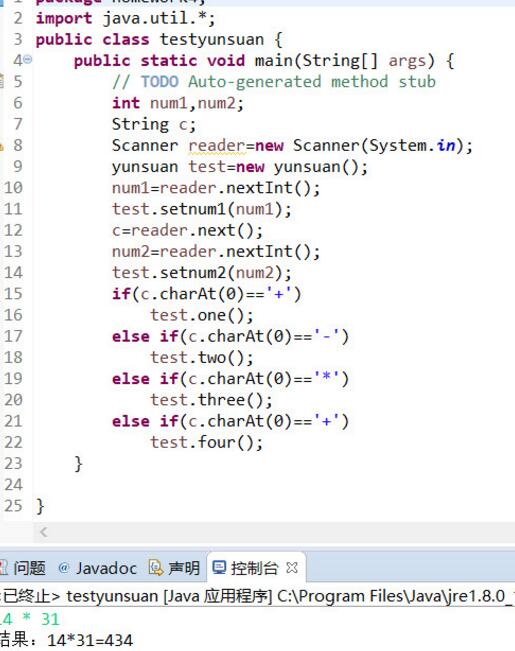