// 判断是否是手机
function isMobile() {
var browser = {
versions: function () {
var u = navigator.userAgent, app = navigator.appVersion;
return { //移动终端浏览器版本信息
trident: u.indexOf('Trident') > -1, //IE内核
presto: u.indexOf('Presto') > -1, //opera内核
webKit: u.indexOf('AppleWebKit') > -1, //苹果、谷歌内核
gecko: u.indexOf('Gecko') > -1 && u.indexOf('KHTML') == -1, //火狐内核
mobile: !!u.match(/AppleWebKit.*Mobile.*/), //是否为移动终端
ios: !!u.match(/\(i[^;]+;( U;)? CPU.+Mac OS X/), //ios终端
android: u.indexOf('Android') > -1 || u.indexOf('Linux') > -1, //android终端或uc浏览器
iPhone: u.indexOf('iPhone') > -1, //是否为iPhone或者QQHD浏览器
iPad: u.indexOf('iPad') > -1, //是否iPad
webApp: u.indexOf('Safari') == -1 //是否web应该程序,没有头部与底部
};
}(),
language: (navigator.browserLanguage || navigator.language).toLowerCase()
}
if (browser.versions.mobile) {
return true;
} else {
return false;
}
}
// 动态加载css
function includeLinkStyle(url) {
var link = document.createElement("link");
link.rel = "stylesheet";
link.type = "text/css";
link.href = url;
document.getElementsByTagName('body')[0].appendChild(link);
}
// 合并数组对象
var merge = tableTunnel.map((item) => {
let location = data.find(data => data.tuniface === item.tuniface);
return { ...item, ip: location.ip, address: location.address }
});
/**
* @desc 函数防抖
* @param fn 函数
* @param delay 延迟执行毫秒数 默认0.5s
*/
export function debounce(fn, delay) {
var delay = delay || 700;
var timer;
return function () {
console.log('调用了debounce方法')
let args = arguments;
if (timer) {
clearTimeout(timer);
}
timer = setTimeout(() => {
timer = null;
fn.apply(this, args);
}, delay);
}
}
/**
* @desc 函数节流
* @param fn 函数
* @param interval 函数执行间隔时间毫秒数 默认1s
*/
export function throttle(fn, interval) {
var last;
var timer;
var interval = interval || 1000;
return function () {
console.log('调用了throttle方法')
var th = this;
var args = arguments;
var now = +new Date();
if (last && now - last < interval) {
clearTimeout(timer);
timer = setTimeout(function () {
last = now;
fn.apply(th, args);
}, interval);
} else {
last = now;
fn.apply(th, args);
}
}
}
let attr = ["name",'age']
let data = [{name:'zhangsan',age:18, gender:'男'},{name:'李四',age:19, gender:'女'},{name:'王二',age:28, gender:'男'}]
console.log(data.map(v=>attr.map(j=>v[j])));
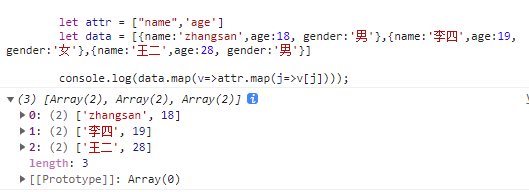