说明:首先在实体类中定义Attribute对象(主要包括表、字段信息),然后通过反射读取该对象信息,再进行数据处理
一、类关系图如下:
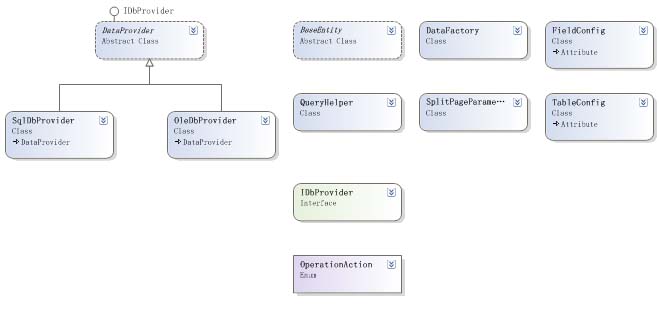
二、各类用途说明
BaseEntity:实体类的基类,实体类必须继承该类。
TableConfig/FieldConfig:描述表及字段配置信息,其中FieldConfig包括FieldName(字段名称)、DataType(数据类型)、Size(字段大小)、IsInsert(是否Insert操作)、IsUpdate(是否Update操作)五个字段信息。
QueryHelper:查询对象。
SplitPageParameter:分页对象。
IDbProvider:数据处理接口。
OperationAction:数据操作枚举。
DataProvider:实现IDbProvider接口,并提供基础数据操作。
SqlDbProvider/OleDbProvider:继承自DataProvider并提供相应操作。
DataFactory:向外部提供IDbProvider服务。
三、配置文件需提供如下信息:

Code
<add key="CNT" value="Data Source=192.168.1.4;User ID=sa;Password=pw;Initial Catalog=DB;Pooling=true"/>
<add key="DBTYPE" value="MSSQL"/>
<add key="EntityFile" value="App.Entity,version=1.0.0.0"/>
CNT:数据连接字符串
DBTYPE:数据库类型(目前只支持MSSQL/OLEDB)
EntityFile:实体类程序集信息
四、接口说明:
void Save(BaseEntity info):保存实体类
void Update(BaseEntity info, QueryHelper query):根据条件更新实体类
void Delete(QueryHelper query):根据条件删除数据
BaseEntity Find(QueryHelper query):根据条件读取一条数据
List<BaseEntity> Finds(QueryHelper query):根据条件读取多条数据
List<BaseEntity> Finds(SplitPageParameter sp):根据条件分页读取数据
void UpdateFields(string tableName, string[] fieldNames, object[] fieldValues, QueryHelper query):根据条件更新指定表的指定字段
五、核心函数说明
(1)、DataProvider.cs

Code
1
/**//// <summary>
2
/// 准备生成DbCommand命令
3
/// </summary>
4
/// <param name="o">实体类对象</param>
5
/// <param name="type">数据类型</param>
6
/// <param name="action">数据操作动作</param>
7
/// <param name="query">条件对象</param>
8
/// <returns></returns>
9
protected IDbCommand PrepareCommand(object o, Type type, OperationAction action, QueryHelper query)
10
{
11
IDbCommand cmd = Factory.CreateCommand();
12
13
//获取当前类型的表配置信息
14
TableConfig configInfo = null;
15
if (type == null)
16
{
17
type = query.TypeInfo;
18
}
19
configInfo = getTableConfigByType(type);
20
21
FieldInfo fldInfo = null;
22
23
IDataParameter parameter = null;
24
25
if (action == OperationAction.Insert)
26
{
27
insert config#region insert config
28
29
StringBuilder sqlInsert = new StringBuilder();
30
StringBuilder sqlValues = new StringBuilder();
31
32
for (int i = 0; i < configInfo.Fields.Count; ++i)
33
{
34
if (!configInfo.Fields[i].IsInsert)
35
continue;
36
if (sqlInsert.Length > 0)
37
{
38
sqlInsert.Append(",");
39
sqlValues.Append(",");
40
}
41
sqlInsert.Append(configInfo.Fields[i].FieldName);
42
sqlValues.Append("@").Append(configInfo.Fields[i].FieldName);
43
44
parameter = MakeParameter(configInfo.Fields[i]);
45
46
//获取字段信息
47
fldInfo = type.GetField(configInfo.Fields[i].FieldName);
48
//从对象中获取该字段的值
49
if (fldInfo != null)
50
{
51
parameter.Value = fldInfo.GetValue(o);
52
}
53
cmd.Parameters.Add(parameter);
54
}
55
//设置Command的命令文本
56
cmd.CommandText = string.Format("INSERT INTO {0}({1}) VALUES({2})", configInfo.TableName, sqlInsert.ToString(), sqlValues.ToString());
57
58
#endregion
59
}
60
else if (action == OperationAction.Update)
61
{
62
update config#region update config
63
64
StringBuilder sqlUpdate = new StringBuilder();
65
66
for (int i = 0; i < configInfo.Fields.Count; ++i)
67
{
68
if (!configInfo.Fields[i].IsUpdate)
69
continue;
70
if (sqlUpdate.Length > 0)
71
{
72
sqlUpdate.Append(",");
73
}
74
sqlUpdate.Append(string.Format(" {0}=@{0}", configInfo.Fields[i].FieldName));
75
parameter = MakeParameter(configInfo.Fields[i]);
76
//获取字段信息
77
fldInfo = type.GetField(configInfo.Fields[i].FieldName);
78
//从对象中获取该字段的值
79
if (fldInfo != null)
80
{
81
parameter.Value = fldInfo.GetValue(o);
82
}
83
cmd.Parameters.Add(parameter);
84
}
85
//设置Command的命令文本
86
cmd.CommandText = string.Format("UPDATE {0} SET {1} ", configInfo.TableName, sqlUpdate.ToString());
87
88
if (query != null)
89
cmd.CommandText += string.Format(" WHERE 1=1 {0}", query.getSQLString);
90
91
#endregion
92
}
93
else if (action == OperationAction.Delete)
94
{
95
delete config#region delete config
96
97
cmd.CommandText = string.Format("DELETE {0}", configInfo.TableName);
98
99
if (query != null)
100
cmd.CommandText += string.Format(" WHERE 1=1 {0}", query.getSQLString);
101
102
#endregion
103
}
104
else if (action == OperationAction.Select)
105
{
106
select config#region select config
107
if (query != null)
108
{
109
if (query.Top > 0)
110
{
111
cmd.CommandText = string.Format("SELECT TOP {0} * FROM {1} WHERE 1=1 {2}", query.Top, configInfo.TableName, query.getSQLString);
112
}
113
else
114
{
115
cmd.CommandText = string.Format("SELECT * FROM {0} WHERE 1=1 {1}", configInfo.TableName, query.getSQLString);
116
}
117
}
118
else
119
{
120
cmd.CommandText = string.Format("SELECT * FROM {0}", configInfo.TableName);
121
}
122
123
#endregion
124
}
125
return cmd;
126
}
此函数根据对象类型,通过反射获取实体类信息及实体类字段值,然后组织SQL语句为IDbCommand赋值
源码下载
应用场景:
适合数据处理较小的应用
使用:

Code
/// <summary>
/// 用户表标识
/// </summary>
[TableConfig("ml_user","用户表标识")]
public class user : BaseEntity
{
/// <summary>
/// 用户表标识
/// </summary>
[FieldConfig("PID",(DbType)SqlDbType.Int,4,false,true)]
public int PID = 0;
/// <summary>
/// 用户登陆名称
/// </summary>
[FieldConfig("UserID",(DbType)SqlDbType.NVarChar,16,true,true)]
public string UserID = String.Empty;
/// <summary>
/// 用户密码
/// </summary>
[FieldConfig("UserPW",(DbType)SqlDbType.NVarChar,16,true,true)]
public string UserPW = String.Empty;
/// <summary>
/// 邮件地址
/// </summary>
[FieldConfig("Mail",(DbType)SqlDbType.NVarChar,32,true,true)]
public string Mail = String.Empty;
/// <summary>
/// 姓名
/// </summary>
[FieldConfig("TrueName",(DbType)SqlDbType.NVarChar,50,true,true)]
public string TrueName = String.Empty;
}
//调用
public class Demo
{
void Demo()
{
//插入
user info = new user();
info.TrueName = "demo";
DataFactory.getDbProvider.Save(info);
//更新
DataFactory.getDbProvider.Update(info,new QueryHelper(string.Format("AND PID={0}",info.PID),typeof(user)));
//删除
DataFactory.getDbProvider.Delete(new QueryHelper("AND PID=1",typeof(user)));
//读取一条数据
DataFactory.getDbProvider.Find(new QueryHelper("AND PID=1",typeof(user)));
}
}
欢迎大家拍砖