Overview |
|
Features of Groovy
Groovy has the following features −
- Support for both static and dynamic typing.
- Support for operator overloading.
- Native syntax for lists and associative arrays.
- Native support for regular expressions.
- Native support for various markup languages such as XML and HTML.
- Groovy is simple for Java developers since the syntax for Java and Groovy are very similar.
- You can use existing Java libraries.
- Groovy extends the java.lang.Object.
|
|
|
|
|
|
|
|
|
|
|
Environment |
|
|
|
|
Basic Syntax |
|
|
Import Statement |
By default, Groovy includes the following libraries in your code, so you don’t need to explicitly import them.
import java.lang.*
import java.util.*
import java.io.*
import java.net.*
import groovy.lang.*
import groovy.util.*
import java.math.BigInteger
import java.math.BigDecimal
|
Comment |
// single line comment
/*
multiple lines comment
*/
|
Semicolons |
not mandatory
|
Identifiers |
Identifiers are used to define variables, functions or other user defined variables. Identifiers start with a letter,
a dollar or an underscore. They cannot start with a number. Here are some examples of valid identifiers
|
|
|
Data Types |
Built-in Data Types |
byte |
This is used to represent a byte value. An example is 2.
|
short |
This is used to represent a short number. An example is 10. |
int |
This is used to represent whole numbers. An example is 1234. |
long |
This is used to represent a long number. An example is 10000090. |
float |
This is used to represent 32-bit floating point numbers. An example is 12.34. |
double |
This is used to represent 64-bit floating point numbers which are longer decimal number representations which may be required at times. An example is 12.3456565. |
char |
This defines a single character literal. An example is ‘a’. |
Boolean |
This represents a Boolean value which can either be true or false. |
String |
These are text literals which are represented in the form of chain of characters. For example “Hello World”. |
|
|
|
Class Numeric |
java.lang.Byte
java.lang.Short
java.lang.Integer
java.lang.Long
java.lang.Float
java.lang.Double
In addition, the following classes can be used for supporting arbitrary precision arithmetic
java.math.BigInteger
java.math.BigDecimal
|
|
|
|
|
|
|
|
|
Variables |
definition |
type v = ...
// or def
def v = ...
|
|
|
Operators |
Arithmetic Operators |
|
Relational operators |
==, !=
>, >=, <, <=
|
Logical Operators |
&&, ||, ! |
Bitwise Operators |
&, |, ^, ~ |
Assignment operators |
+=, -=, *=, /=, %= |
Range Operators |
|
Loops |
while |
while(condition) {
statement #1
statement #2
...
}
|
for |
for(variable declaration;expression;Increment) {
statement #1
statement #2
…
}
|
for-in |
for(variable in range) {
statement #1
statement #2
…
}
|
break |
|
continue |
|
|
|
Decision Making |
if |
if(condition) {
statement #1
statement #2
...
} else if(condition) {
statement #3
statement #4
} else {
statement #5
statement #6
}
|
switch |
switch(expression) {
case expression #1:
statement #1
...
case expression #2:
statement #2
...
case expression #N:
statement #N
...
default:
statement #Default
...
}
|
|
|
|
|
|
|
|
|
Methods |
|
|
A method is in Groovy is defined with a return type or with the def keyword.
def methodName() { // def can be substituted with data type
//Method code
}
def methodName(type p1, type p2 = v2, ...) { // type can be omitted
...
}
void methodName(...) { ... } // specifing the return type
|
|
Methods can receive any number of arguments. It’s not necessary that the types are explicitly defined when defining the arguments. |
|
Modifiers such as public, private and protected can be added. By default, if no visibility modifier is provided, the method is public.
|
public, private and protected |
|
default is public |
|
|
Instance methods |
|
Local and External Parameter Names |
|
this method for Properties |
this |
|
|
|
|
File I/O |
|
https://www.cnblogs.com/lancgg/p/14020133.html |
Reading files |
eachLine
class Example {
static void main(String[] args) {
new File("E:/Example.txt").eachLine {
line -> println "line : $line";
}
}
}
|
Reading the Contents of a File
as an Entire String
|
text
class Example {
static void main(String[] args) {
File file = new File("E:/Example.txt")
println file.text
}
}
|
Writing to Files |
class Example {
static void main(String[] args) {
new File('E:/','Example.txt').withWriter('utf-8') {
writer -> writer.writeLine 'Hello World'
}
}
}
|
Getting the Size of a File |
long length()
class Example {
static void main(String[] args) {
File file = new File("E:/Example.txt")
println "The file ${file.absolutePath} has ${file.length()} bytes"
}
}
|
Testing if a File is a Directory |
boolean isDirectory() |
Creating a Directory |
boolean mkdir()
boolean mkdirs()
|
Deleting a File |
boolean delete() |
Copying files |
class Example {
static void main(String[] args) {
def src = new File("E:/Example.txt")
def dst = new File("E:/Example1.txt")
dst << src.text
}
}
|
Getting Directory Contents |
File[] listRoots()
class Example {
static void main(String[] args) {
def rootFiles = new File("test").listRoots()
rootFiles.each {
file -> println file.absolutePath
}
}
}
eachFile
class Example {
static void main(String[] args) {
new File("E:/Temp").eachFile() {
file->println file.getAbsolutePath()
}
}
}
eachFileRecurse
class Example {
static void main(String[] args) {
new File("E:/temp").eachFileRecurse() {
file -> println file.getAbsolutePath()
}
}
}
|
|
|
|
|
Optionals |
|
In Groovy, optional typing is done via the ‘def ’ keyword. Following is an example of the usage of the def method
class Example {
static void main(String[] args) {
// Example of an Integer using def
def aint = 100;
println(aint);
// Example of an float using def
def bfloat = 100.10;
println(bfloat);
// Example of an Double using def
def cDouble = 100.101;
println(cDouble);
// Example of an String using def
def dString = "HelloWorld";
println(dString);
}
}
|
|
|
Numbers |
|
Number ------> Byte
------> Short
------> Integer
------> Long
------> Float
------> Double
|
methods |
https://www.tutorialspoint.com/groovy/groovy_numbers.htm |
|
|
|
|
Strings |
|
Groovy offers a variety of ways to denote a String literal. Strings in Groovy can be enclosed in single quotes (’), double quotes (“), or triple quotes (“””). Further, a Groovy String enclosed by triple quotes may span multiple lines.
class Example {
static void main(String[] args) {
String a = 'Hello Single';
String b = "Hello Double"; // interpolating values by ${...}
String str = '''
abc
efd
''';
println(a);
println(b);
println(c);
}
}
|
String Indexing |
def s = "123456789";
println(s[0..2]); // 123
println(s[-1]); // 9
println(s[-2..-1]); // 89
|
methods |
|
|
|
|
|
|
|
Ranges |
|
- 1..10 - An example of an inclusive Range
- 1..<10 - An example of an exclusive Range
- ‘a’..’x’ – Ranges can also consist of characters
- 10..1 – Ranges can also be in descending order
- ‘x’..’a’ – Ranges can also consist of characters and be in descending order.
|
methods |
boolean contains(Object obj)
|
|
Object get(int index)
|
|
Comparable getFrom()
Comparable getTo()
|
|
boolean isReverse()
|
|
int size()
|
|
List subList(int fromIndex, int toIndex)
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Lists |
|
- [11, 12, 13, 14] – A list of integer values
- [‘Angular’, ‘Groovy’, ‘Java’] – A list of Strings
- [1, 2, [3, 4], 5] – A nested list
- [‘Groovy’, 21, 2.11] – A heterogeneous list of object references
- [ ] – An empty list
|
methods |
boolean add(Object value)
void add(int index, Object value)
|
|
boolean contains(Object value)
|
|
Object get(int index)
|
|
boolean isEmpty()
|
|
List minus(Collection collection)
|
|
List plus(Collection collection)
|
|
Object pop()
|
|
Object remove(int index)
|
|
List reverse()
|
|
int size()
|
|
List sort()
|
|
|
|
|
|
|
|
|
|
|
Maps |
|
|
methods |
boolean containsKey(Object key)
|
|
Object get(Object key)
|
|
Object put(Object key, Object value)
|
|
int size()
|
|
Set keySet()
|
|
Collection values()
|
|
|
|
|
|
|
|
|
|
|
Dates&Times |
|
|
Regular Expressions |
|
def regex = ~'Groovy'
|
|
When the Groovy operator =~ appears as a predicate (expression returning a Boolean) in if and while statements (see Chapter 8), the String operand on the left is matched against the regular expression operand on the right. |
|
begining of a line |
^ |
end of a line |
$ |
quantifiers |
+ |
one or more times
1+
|
* |
zero or more occurrences
0+
|
? |
zero or once
0~1
|
The metacharacter { and } |
{n}
{n,m} n~m
|
|
. |
the wildcard character |
character classes
[]
|
https://www.cnblogs.com/lancgg/p/8281885.html
指定范围, 可用 - |
[AB], [ABC], [01A] |
[A-Z] |
[A-Za-z] |
[0-9] |
想指定"-"必须放开头或者结尾 [-...] [...-] |
[^...] 除去指定的 |
|
|
|
|
|
|
|
Exception Handling |
|
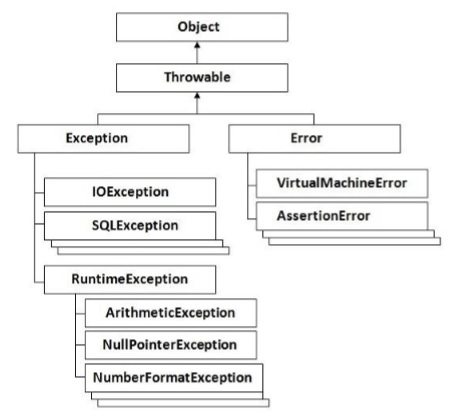
checked exceptions |
The classes that extend Throwable class except RuntimeException and Error are known as checked exceptions e.g.IOException, SQLException etc. Checked exceptions are checked at compile-time. |
unchecked exceptions |
The classes that extend RuntimeException are known as unchecked exceptions, e.g., ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException etc. Unchecked exceptions are not checked at compile-time rather they are checked at runtime. |
Error |
Error is irrecoverable e.g. OutOfMemoryError, VirtualMachineError, AssertionError etc. |
|
Catching Exceptions |
try {
//Protected code
} catch(ExceptionType1 e1) {
//Catch block
} catch(ExceptionType2 e2) {
//Catch block
} catch(ExceptionType3 e3) {
//Catch block
} finally {
//The finally block always executes.
}
|
Exception methods |
public String getMessage()
|
Returns a detailed message about the exception that has occurred. This message is initialized in the Throwable constructor. |
public Throwable getCause()
|
Returns the cause of the exception as represented by a Throwable object. |
public String toString()
|
Returns the name of the class concatenated with the result of getMessage() |
public void printStackTrace()
|
Prints the result of toString() along with the stack trace to System.err, the error output stream. |
public StackTraceElement [] getStackTrace()
|
Returns an array containing each element on the stack trace. The element at index 0 represents the top of the call stack, and the last element in the array represents the method at the bottom of the call stack. |
public Throwable fillInStackTrace()
|
Fills the stack trace of this Throwable object with the current stack trace, adding to any previous information in the stack trace. |
|
|
|
|
|
|
|
Obeject Oriented |
|
https://www.tutorialspoint.com/groovy/groovy_object_oriented.htm |
getter and setter Methods |
|
Instance Methods |
|
Inheritance |
|
Extends |
class Example {
static void main(String[] args) {
Student st = new Student();
st.StudentID = 1;
st.Marks1 = 10;
st.name = "Joe";
println(st.name);
}
}
class Person {
public String name;
public Person() {}
}
class Student extends Person {
int StudentID
int Marks1;
public Student() {
super();
}
}
|
Inner Classes |
Inner classes are defined within another classes. The enclosing class can use the inner class as usual. On the other side, a inner class can access members of its enclosing class, even if they are private. Classes other than the enclosing class are not allowed to access inner classes. |
Abstract Classes |
Abstract classes represent generic concepts, thus, they cannot be instantiated, being created to be subclassed. Their members include fields/properties and abstract or concrete methods. Abstract methods do not have implementation, and must be implemented by concrete subclasses. Abstract classes must be declared with abstract keyword. Abstract methods must also be declared with abstract keyword.
class Example {
static void main(String[] args) {
Student st = new Student();
st.StudentID = 1;
st.Marks1 = 10;
st.name="Joe";
println(st.name);
println(st.DisplayMarks());
}
}
abstract class Person {
public String name;
public Person() { }
abstract void DisplayMarks();
}
class Student extends Person {
int StudentID
int Marks1;
public Student() {
super();
}
void DisplayMarks() {
println(Marks1);
}
}
|
Interface |
An interface defines a contract that a class needs to conform to. An interface only defines a list of methods that need to be implemented, but does not define the methods implementation. An interface needs to be declared using the interface keyword. An interface only defines method signatures. Methods of an interface are always public. It is an error to use protected or private methods in interfaces.
class Example {
static void main(String[] args) {
Student st = new Student();
st.StudentID = 1;
st.Marks1 = 10;
println(st.DisplayMarks());
}
}
interface Marks {
void DisplayMarks();
}
class Student implements Marks {
int StudentID
int Marks1;
void DisplayMarks() {
println(Marks1);
}
}
|
Generics |
|
class Example {
static void main(String[] args) {
// Creating a generic List collection
ListType<String> lststr = new ListType<>();
lststr.set("First String");
println(lststr.get());
ListType<Integer> lstint = new ListType<>();
lstint.set(1);
println(lstint.get());
}
}
public class ListType<T> {
private T localt;
public T get() {
return this.localt;
}
public void set(T plocal) {
this.localt = plocal;
}
}
|
|
|
|
|
|
|
|
|
|
|
Traits |
Implementing Interfaces |
|
Properties |
A trait may define properties.
lass Example {
static void main(String[] args) {
Student st = new Student();
st.StudentID = 1;
println(st.DisplayMarks());
println(st.DisplayTotal());
}
interface Total {
void DisplayTotal()
}
trait Marks implements Total {
int Marks1;
void DisplayMarks() {
this.Marks1 = 10;
println(this.Marks1);
}
void DisplayTotal() {
println("Display Total");
}
}
class Student implements Marks {
int StudentID
}
}
|
Extending Traits |
Traits may extend another trait, in which case you must use the extends keyword. |
|
|
|
|
|
|
Closures |
|
class Example {
static void main(String[] args) {
def clos = {println "Hello World"};
clos.call();
}
}
The code block referenced by this identifier can be executed with the call statement.
|
Formal parameters in closures |
More formally, closures can refer to variables at the time the closure is defined.
class Example {
static void main(String[] args) {
def clos = {println "Hello ${it}"};
clos.call("World");
}
}
|
Using Closures in Methods |
Closures can also be used as parameters to methods. In Groovy, a lot of the inbuilt methods for data types such as Lists and collections have closures as a parameter type. |
methods |
Object find(Closure closure)
|
class Example {
static void main(String[] args) {
def lst = [1,2,3,4];
def value;
value = lst.find {element -> element > 2}
println(value);
}
}
|
List findAll(Closure closure)
|
class Example {
static void main(String[] args) {
def lst = [1,2,3,4];
def value;
value = lst.findAll{element -> element > 2}
value.each {println it}
}
}
|
boolean any(Closure closure)
boolean every(Closure closure)
|
|
List collect(Closure closure)
|
class Example {
static void main(String[] args) {
def lst = [1,2,3,4];
def newlst = [];
newlst = lst.collect {element -> return element * element}
println(newlst);
}
}
|
|
|
|
|
|
|
|
|
|
Annotations |
|
https://groovy-lang.org/objectorientation.html#_annotations |
|
|
|
|
|
|
|
|
|
|
XML |
|
|
|
|
|
|
|
|
|
|
|
|
JMX |
|
|
|
|
|
|
|
|
|
|
|
|
JSON |
JsonSlurper |
JsonSlurper is a class that parses JSON text or reader content into Groovy data
Structures such as maps, lists and primitive types like Integer, Double, Boolean and String.
|
JsonOutput |
This method is responsible for serialising Groovy objects into JSON strings. |
|
|
|
|
|
|
|
|
DSLS |
|
|
|
|
|
|
|
|
|
|
|
|
Database |
|
|
|
|
|
|
|
|
|
|
|
|
Builders |
|
|
|
|
|
|
|
|
|
|
|
|
Commind Line |
|
|
|
|
|
|
|
|
|
|
|
|
Unit Test |
|
|
|
|
|
|
|
|
|
|
|
|
Template Engine |
|
|
|
|
|
|
|
|
|
|
|
|
Meta Object Programming |
|
|
|
|
|
|
|
|
|
|
|
|