Springboot配置多数据源
步骤一:SpringBootMainApplication 代码配置。
package com.xxx.springbootmain;
import com.spring4all.mongodb.EnableMongoPlus;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration;
import org.springframework.boot.autoconfigure.orm.jpa.HibernateJpaAutoConfiguration;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.boot.web.support.SpringBootServletInitializer;
import org.springframework.scheduling.annotation.EnableScheduling;
import org.springframework.transaction.annotation.EnableTransactionManagement;
@EnableMongoPlus
@EnableTransactionManagement
@SpringBootApplication(exclude = {DataSourceAutoConfiguration.class,HibernateJpaAutoConfiguration.class},
scanBasePackages = {"com.ikey.springbootmain",
"com.ikey.springbootmodule",
"com.ikey.springbootviolation"})
@EnableScheduling
public class SpringBootMainApplication extends SpringBootServletInitializer {
@Override
protected SpringApplicationBuilder configure(SpringApplicationBuilder builder) {
return builder.sources(SpringBootMainApplication.class);
}
public static void main(String[] args) {
SpringApplication.run(SpringBootMainApplication.class, args);
}
}
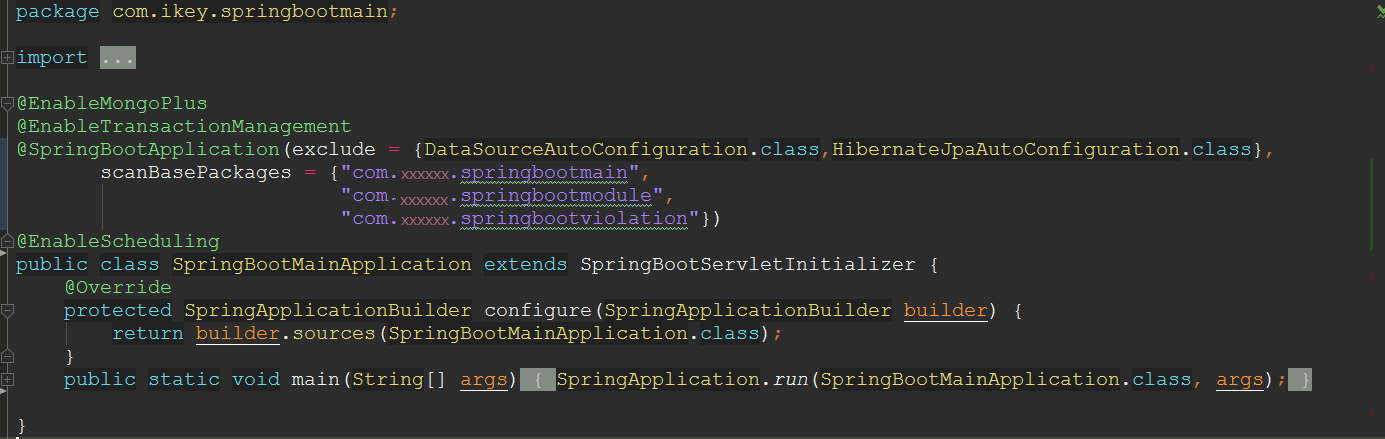
步骤二:数据源配置文件
spring:
datasource:
druid:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://xxxxxx
username: root
password:
filters: mergeStat,wall
initial-size: 10
max-active: 200
min-idle: 10
max-wait: 6000
test-on-borrow: true
test-on-return: true
test-while-idle: true
time-between-eviction-runs-millis: 60000
min-evictable-idle-time-millis: 300000
web-stat-filter:
enabled: true
url-pattern: /*
exclusions: '*.js,*.gif,*.jpg,*.bmp,*.png,*.css,/druid/*'
stat-view-servlet:
enabled: true
url-pattern: /druid/*
reset-enable: false
login-password: xxxx
login-username: xxxxx
pool-prepared-statements: true
max-open-prepared-statements: 20
#oracle数据源--共享单车投诉
oracleDataSource:
driverClassName: oracle.jdbc.OracleDriver
url: jdbc:oracle:thin:@xxxxx
username: outerviewer
password:
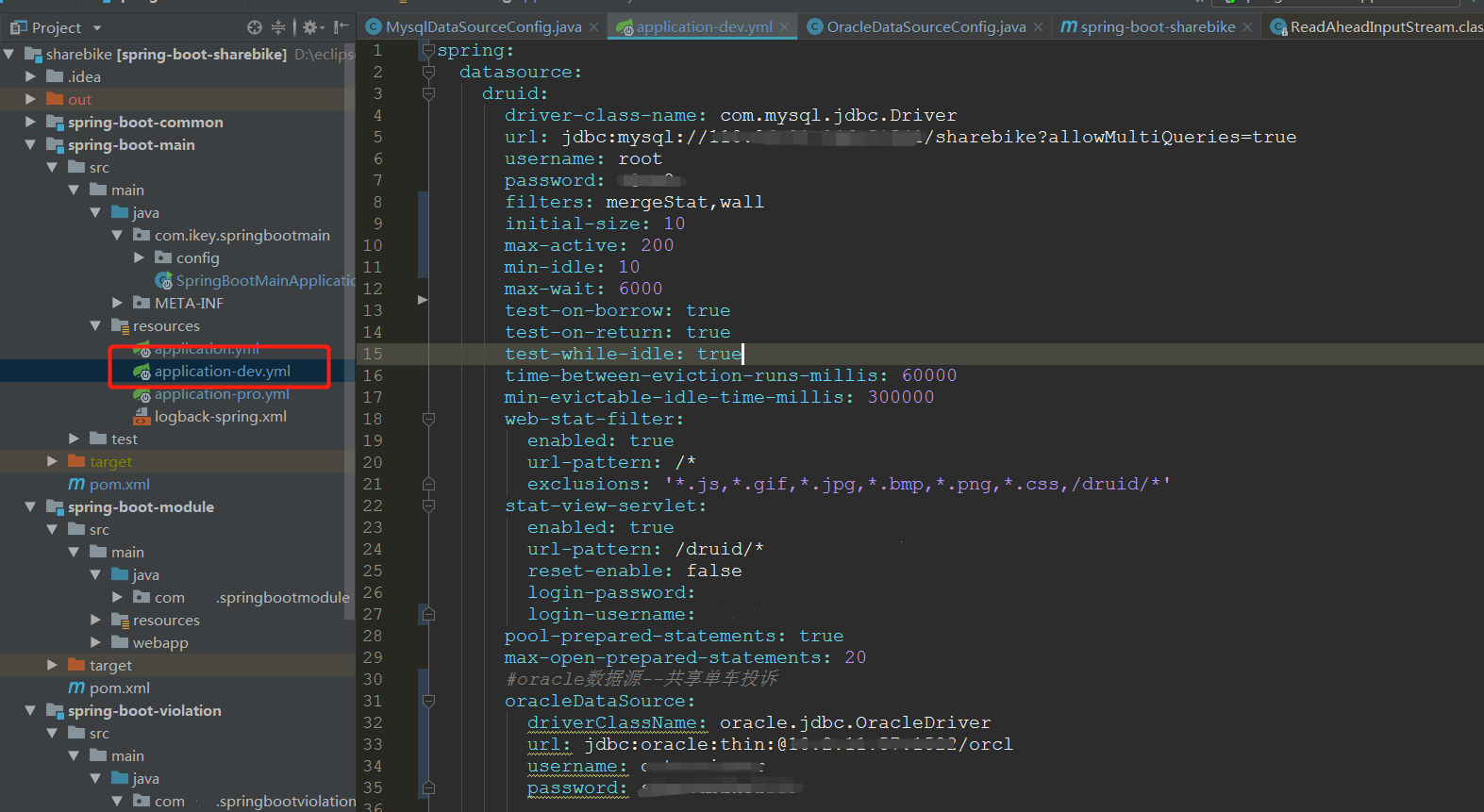
步骤三:MysqlDataSourceConfig
package com.xxxx.springbootmodule.config;
import com.alibaba.druid.spring.boot.autoconfigure.DruidDataSourceBuilder;
import com.baomidou.mybatisplus.plugins.PaginationInterceptor;
import com.baomidou.mybatisplus.spring.MybatisSqlSessionFactoryBean;
import org.apache.ibatis.plugin.Interceptor;
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionTemplate;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import org.springframework.jdbc.datasource.DataSourceTransactionManager;
import javax.sql.DataSource;
import java.sql.SQLException;
/**
* @Author: Laughing
* @Date: 2018-11-26 18:00
* @Description: mysql数据源配置
*/
@Configuration
@MapperScan(basePackages = "com.xxxx.springbootmodule.mapper",sqlSessionTemplateRef = "mysqlSqlSessionTemplate")
public class MysqlDataSourceConfig {
@ConfigurationProperties(prefix = "spring.dataSource.druid")
@Bean(name = "mysqlDataSource")
@Primary
public DataSource mysqlDataSource() throws SQLException {
return DruidDataSourceBuilder.create().build();
}
@Bean(name = "mysqlSessionFactory")
@Primary
public SqlSessionFactory mysqlSessionFactory(@Qualifier(value = "mysqlDataSource") DataSource dataSource,
@Qualifier(value = "mysqlPaginationInterceptor")PaginationInterceptor paginationInterceptor
) throws Exception {
MybatisSqlSessionFactoryBean bean = new MybatisSqlSessionFactoryBean();
bean.setDataSource(dataSource);
bean.setMapperLocations(new PathMatchingResourcePatternResolver().getResources("classpath:mapper/*.xml"));
Interceptor[] interceptors = new Interceptor[]{paginationInterceptor};
bean.setPlugins(interceptors);
return bean.getObject();
}
@Bean(name = "mysqlTransactionManager")
@Primary
public DataSourceTransactionManager dataSourceTransactionManager1(@Qualifier("mysqlDataSource") DataSource dataSource) {
return new DataSourceTransactionManager(dataSource);
}
@Bean(name = "mysqlSqlSessionTemplate")
@Primary
public SqlSessionTemplate testSqlSessionTemplate(@Qualifier("mysqlSessionFactory") SqlSessionFactory sqlSessionFactory) throws Exception {
return new SqlSessionTemplate(sqlSessionFactory);
}
}
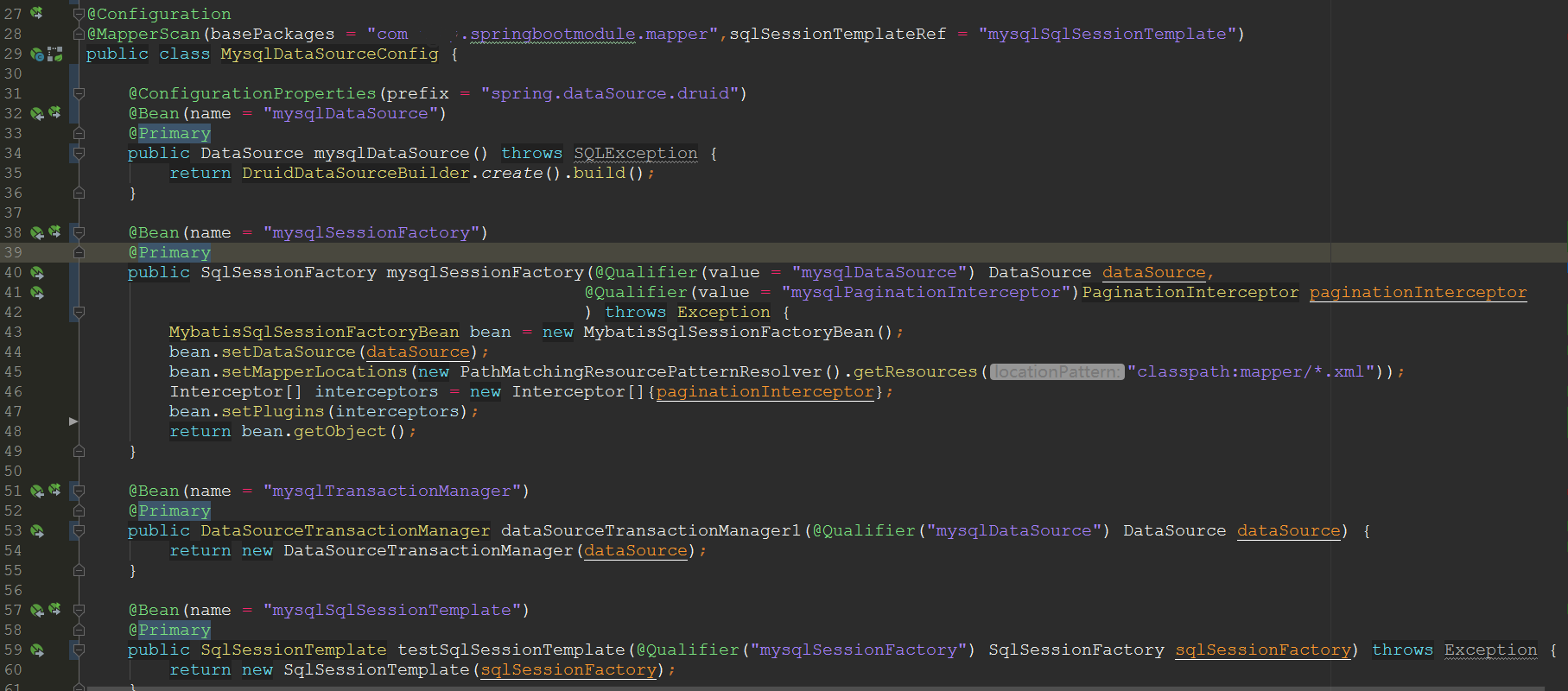
步骤四:OracleDataSourceConfig
package com.xxxx.springbootviolation.config;
import com.alibaba.druid.spring.boot.autoconfigure.DruidDataSourceBuilder;
import com.baomidou.mybatisplus.entity.GlobalConfiguration;
import com.baomidou.mybatisplus.plugins.PaginationInterceptor;
import com.baomidou.mybatisplus.spring.MybatisSqlSessionFactoryBean;
import org.apache.ibatis.plugin.Interceptor;
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.mybatis.spring.SqlSessionTemplate;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import org.springframework.jdbc.datasource.DataSourceTransactionManager;
import javax.sql.DataSource;
import java.sql.SQLException;
/**
* @Author: Laughing
* @Date: 2018-11-26 19:18
* @Description: oracle数据源配置
*/
@Configuration
@MapperScan(basePackages = "com.xxxx.springbootviolation.mapper",sqlSessionTemplateRef = "oracleSqlSessionTemplate")
public class OracleDataSourceConfig {
@ConfigurationProperties(prefix = "spring.datasource.druid.oracleDataSource")
@Bean(name = "oracleDataSource")
@Primary
public DataSource oracleDataSource() throws SQLException {
return DruidDataSourceBuilder.create().build();
}
@Bean(name = "oracleSessionFactory")
@Primary
public SqlSessionFactory sqlSessionFactory1(@Qualifier(value = "oracleDataSource") DataSource dataSource,
@Qualifier(value = "oraclePaginationInterceptor")PaginationInterceptor paginationInterceptor
) throws Exception {
MybatisSqlSessionFactoryBean bean = new MybatisSqlSessionFactoryBean();
bean.setDataSource(dataSource);
bean.setMapperLocations(new PathMatchingResourcePatternResolver().getResources("classpath:oracleMapper/*Mapper.xml"));
Interceptor[] interceptors = new Interceptor[]{paginationInterceptor};
bean.setPlugins(interceptors);
return bean.getObject();
}
@Bean(name = "oracleTransactionManager")
@Primary
public DataSourceTransactionManager dataSourceTransactionManager1(@Qualifier("oracleDataSource") DataSource dataSource) {
return new DataSourceTransactionManager(dataSource);
}
@Bean(name = "oracleSqlSessionTemplate")
@Primary
public SqlSessionTemplate testSqlSessionTemplate(@Qualifier("oracleSessionFactory") SqlSessionFactory sqlSessionFactory) throws Exception {
return new SqlSessionTemplate(sqlSessionFactory);
}
}
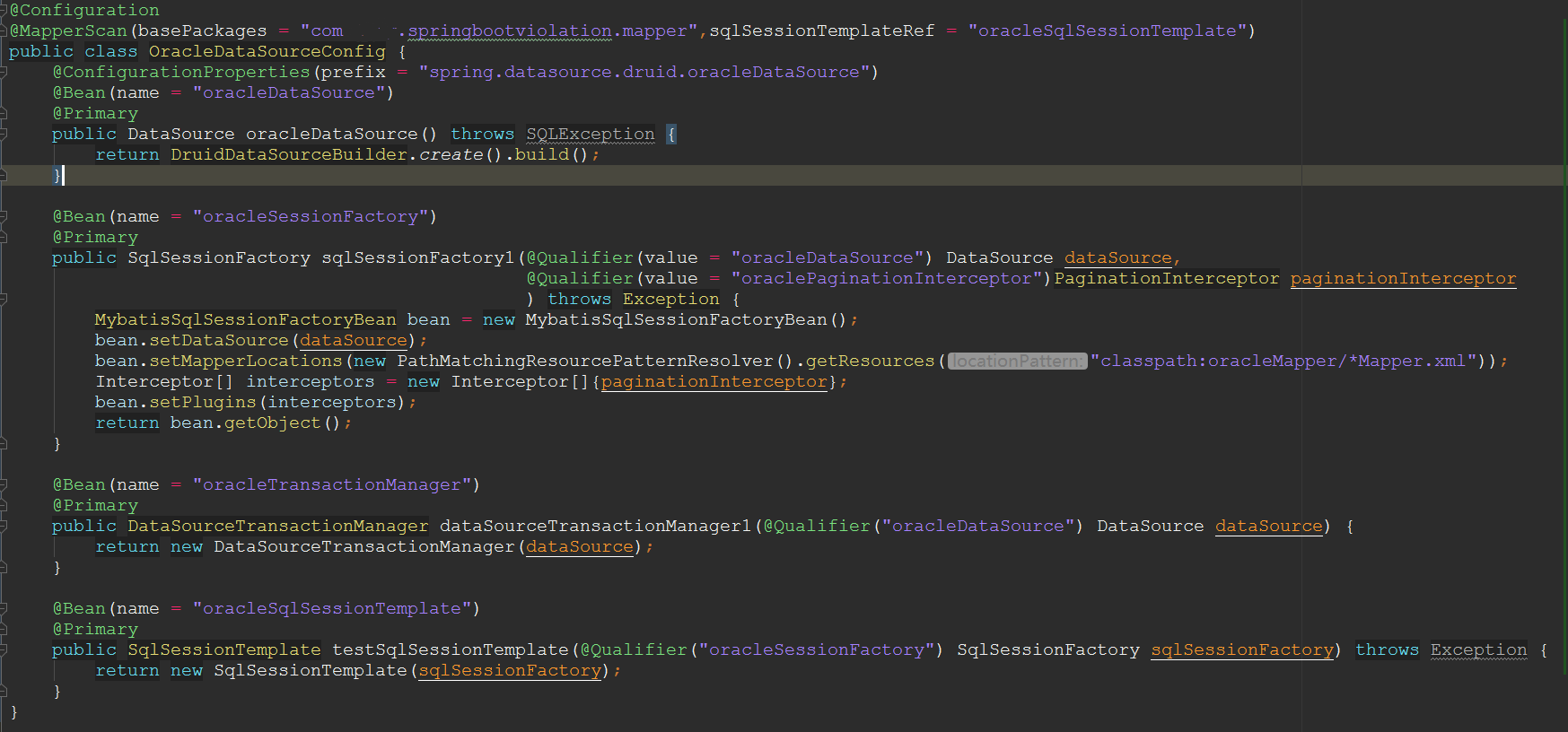
步骤五:引入包
<mybatisplus-spring-boot-starter.version>1.0.5</mybatisplus-spring-boot-starter.version>
<mybatisplus.version>2.1.8</mybatisplus.version>
<!-- mybatis-plus begin -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatisplus-spring-boot-starter</artifactId>
<version>${mybatisplus-spring-boot-starter.version}</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus</artifactId>
<version>${mybatisplus.version}</version>
</dependency>
<!--数据库连接池-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.1.6</version>
</dependency>
错误情况及解决方法:
1.错误:
Caused by: org.springframework.beans.factory.NoUniqueBeanDefinitionException: No qualifying bean of type 'javax.sql.DataSource' available: more than one 'primary' bean found among candidates: [mysqlDataSource, oracleDataSource]
出现原因:@SpringBootApplication未配置DataSourceAutoConfiguration.class
这个jar包中 有个DataSourceAutoConfiguration 会初始化DataSourceInitializer 这个类 ,这个类有一个init方法 会去获取DataSource(数据源),初始化方法中 会获取数据源 需要初始化一些ddl操作 也是就runSchemaScripts()方法 检查初始化时是否需要执行sql script ,当你有两个数据源的时候,程序不知道取哪一个 ,所以报错。 spring boot 启动类加上 exclude = DataSourceAutoConfiguration.class 代表启动项目的时候 不加载这个类
解决方法:
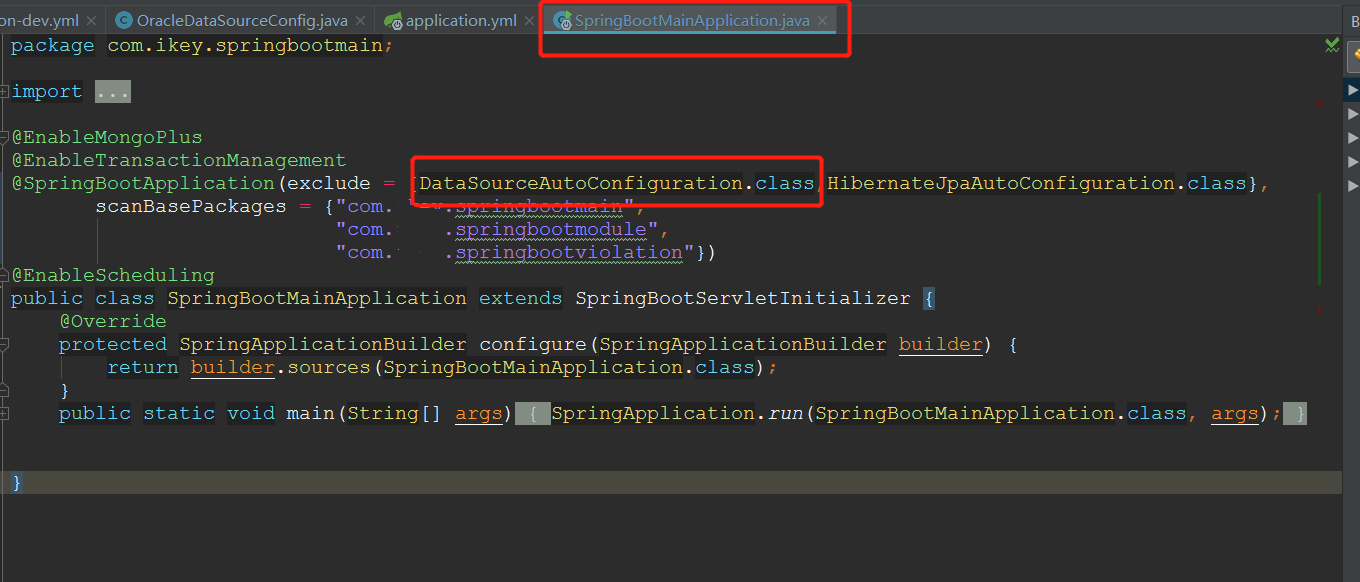
2.错误:
Cannot determine embedded database driver class for database type NONE. If you want an embedded database please put a supported one on the classpath. If you have database settings to be loaded from a particular profile you may need to active it (the profiles "dev" are currently active).
出现原因:未配置主数据源,数据库链接池中找不到driverClassName
解决方法:
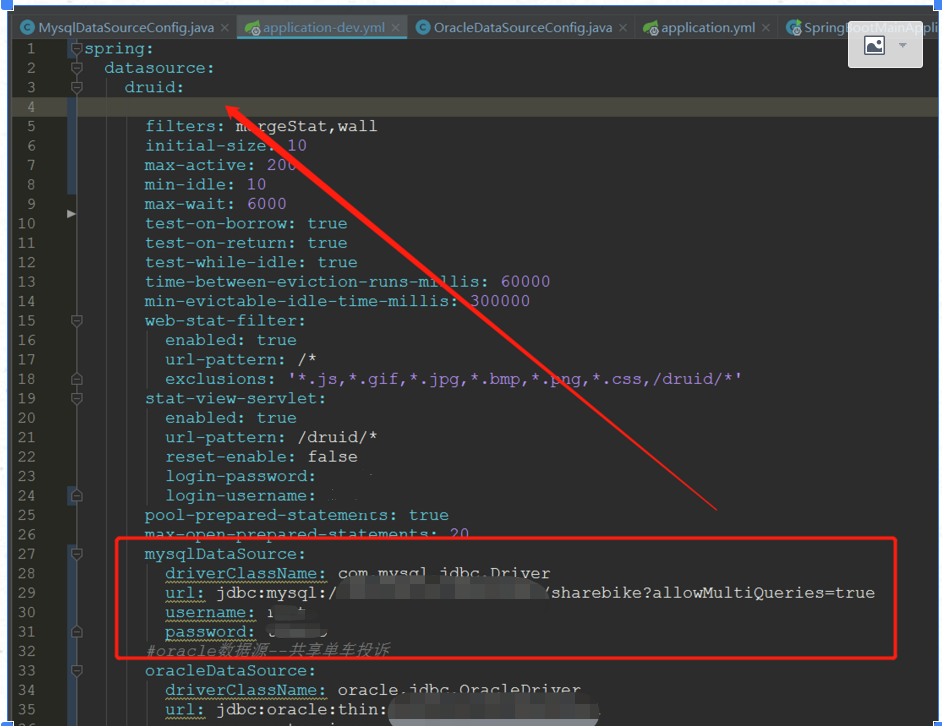
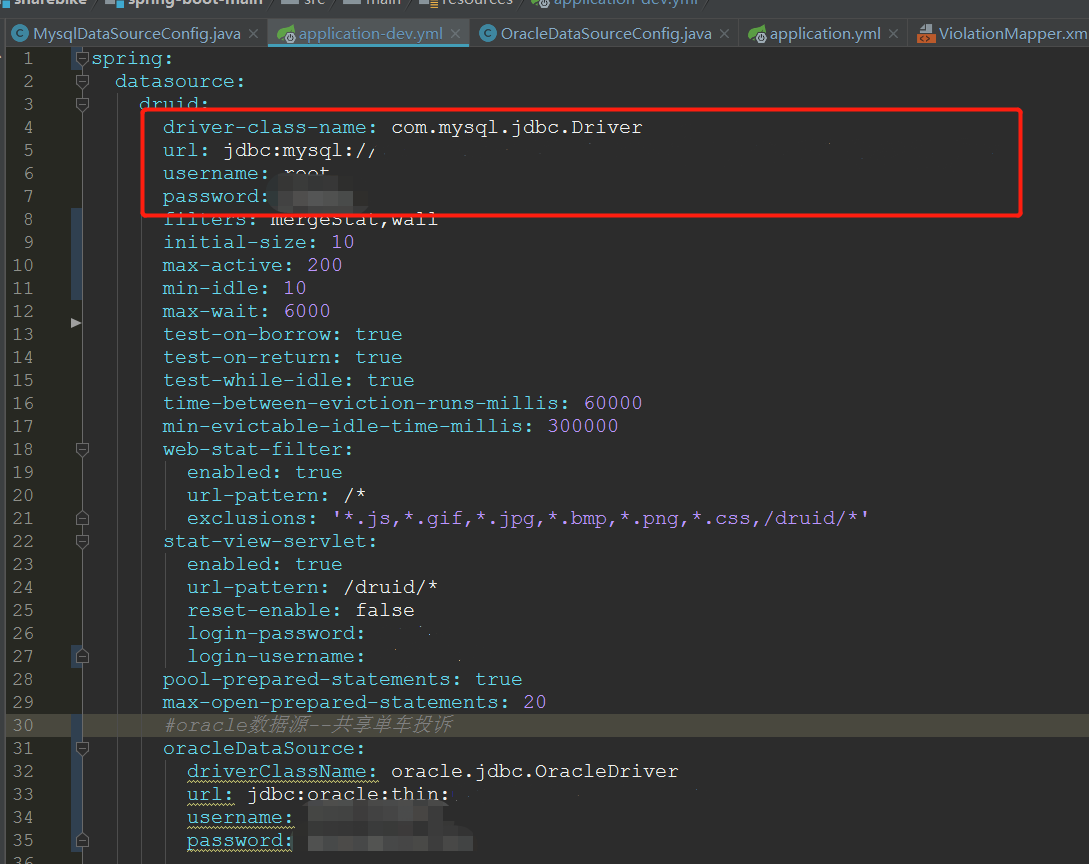
3.错误:
No unique bean of type [org.apache.ibatis.session.SqlSessionFactory] is defined: expected single matching bean but found 2: [sqlSessionFactory1, sqlSessionFactory2]
出现原因:sqlsessionFactory不是唯一的
解决方法:使用sqlSessionTemplate代替sqlSessionFactory
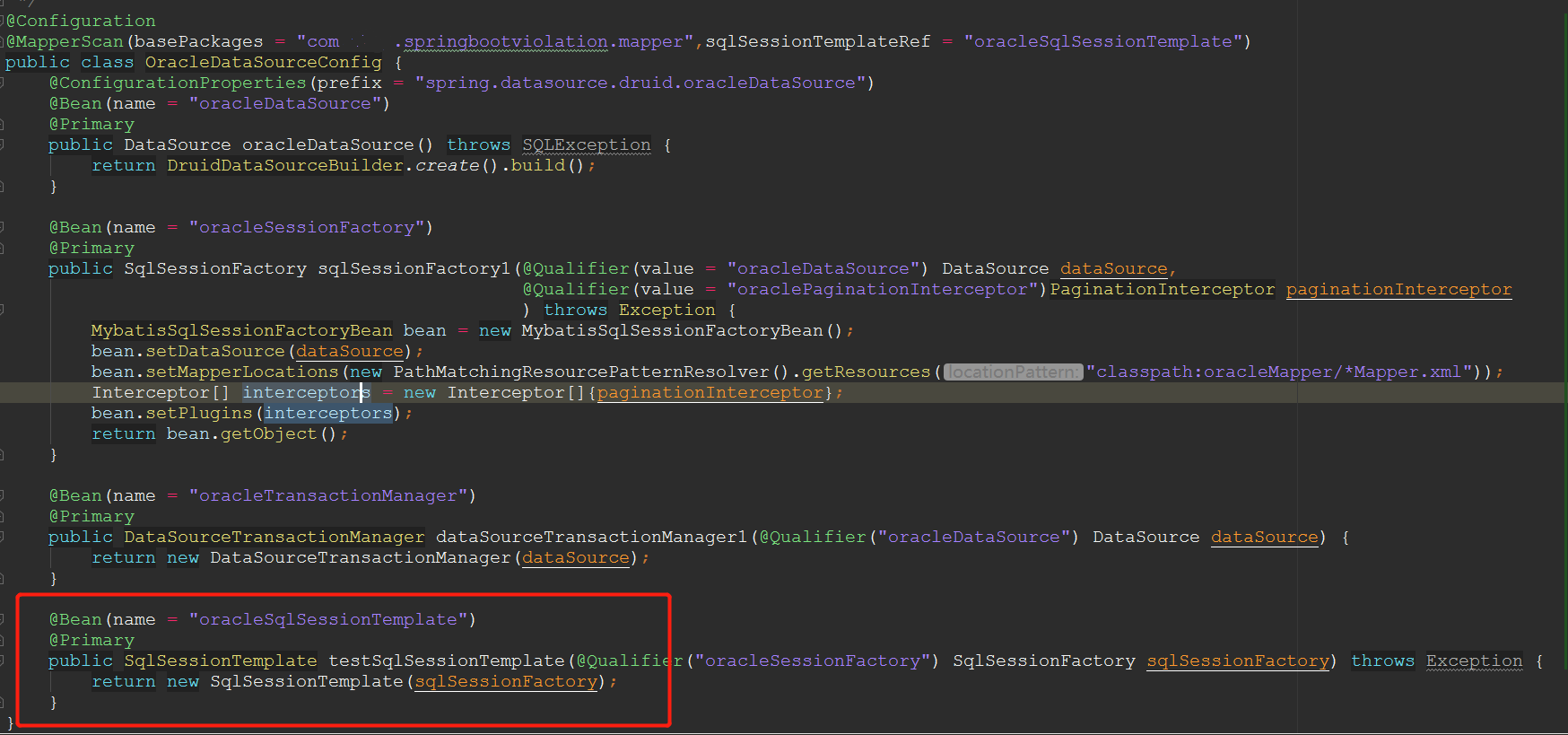
4.错误
org.apache.ibatis.binding.BindingException: Invalid bound statement (not found): com.xxxx.springbootviolation.mapper.ViolationMapper.count
出现原因:打包项目之后两个数据的源的查询文件都在同一个mapper文件夹下,查询时将找不到对应的方法。
解决办法:两个数据源的mybatis映射文件放在不同的文件夹下面。