chdir
int chdir(const char *path);
// 改变文件路径,改变应用程序执行时进程所在的路径
int fchdir(int fd);
getcdw
char *getcwd(char *buf, size_t size);
// 相当于linux命令:pwd
char *getwd(char *buf);
char *get_current_dir_name(void);
mkdir
int mkdir(const char *pathname, mode_t mode);
//
rmdir
int rmdir(const char *pathname);
//
opendir,closedir
DIR *opendir(const char *name);
DIR *fdopendir(int fd);
int closedir(DIR *dirp);
The opendir() and fdopendir() functions return a pointer to the directory stream
On error, NULL is returned, and errno is set appropriately
// 成功,返回一个指向目录流的指针;失败,返回NULL,并设备errno值
readdir
struct dirent *readdir(DIR *dirp);
int readdir_r(DIR *dirp, struct dirent *entry, struct dirent **result);
struct dirent
{
ino_t d_ino; // 此目录进入点的inode
off_t d_off; // 目录文件开头至目录进入点的位移
unsigned short d_reclen; // d_name的长度,不包含NULL字符
unsigned char d_type; // 所指的文件类型
char d_name[256]; // filename,文件名
};
// d_type的取值
DT_BLK // This is a block device.块设备
DT_CHR // This is a character device.字符设备
DT_DIR // This is a directory.目录
DT_FIFO // This is a named pipe (FIFO).管道
DT_LNK // This is a symbolic link.符号链接
DT_REG // This is a regular file.普通文件
DT_SOCK // This is a UNIX domain socket.套接字
DT_UNKNOWN // The file type is unknown.未知
The readdir() function returns a pointer to a dirent structure representing the next directory entry in the directory stream pointed to by dirp. It returns NULL on reaching the end of the directory stream or if an error occurred.
// 成功,返回一个指向由dirp指针所指的目录流中dirent结构体所指示下一个目录入口的指针
// 到达目录尾部或发生错误,返回NULL
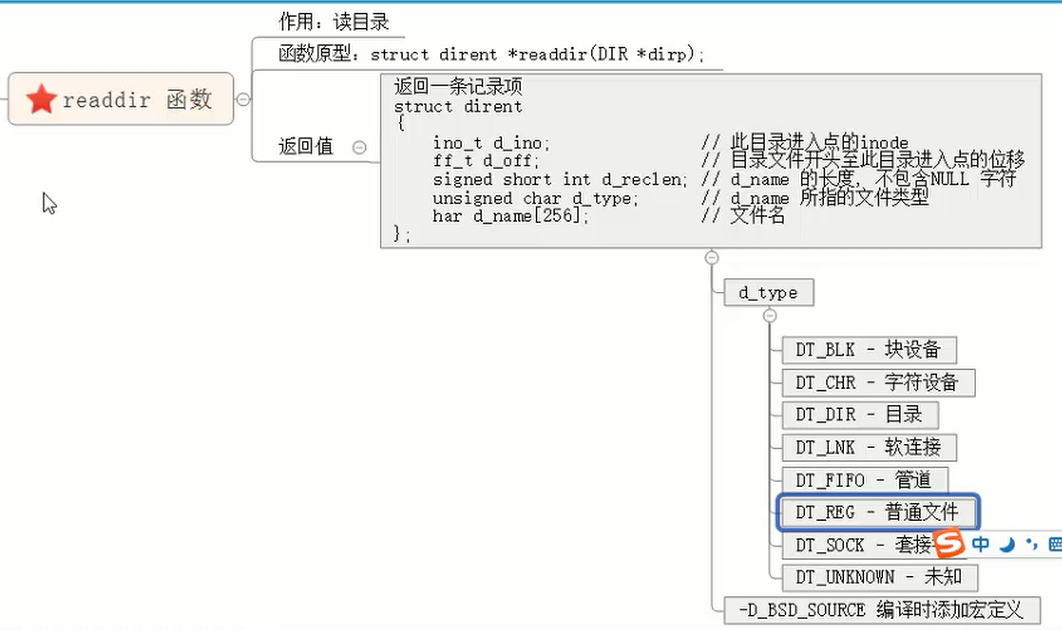
读目录例子
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <dirent.h>
// 读目录,获取目中文件个数
int xxx_getFileNumber(char* path)
{
// 打开目录
DIR* dir = opendir(path);
if(dir == NULL)
{
perror("opendir failed:");
exit(1);
}
// 定义辅助指针ptr
struct dirent* ptr = NULL;
// 定义字符数组,存放拼接的新目录
char path_new[1024] = { 0 };
// 记录总的普通文件个数
int total = 0;
// 遍历当前打开的目录
while((ptr = readdir(dir)) != NULL)
{
// 读取到的是当前目录和上级目录,即遇到它们不处理
if(strcmp(ptr->d_name, ".") == 0 || strcmp(ptr->d_name, "..") == 0)
{
continue;
}
// 读取到的是普通目录,则递归读目录
if(ptr->d_type == DT_DIR)
{
// 先拼接一个目录名称,传递给readdir函数读
sprintf(path_new, "%s/%s", path, ptr->d_name);
total += xxx_getFileNumber(path_new);
}
// 如果是普通文件,文件数目增加
if(ptr->d_type == DT_REG)
{
total++;
}
}
closedir(dir);
return total;
}
int main(int argc, char** argv)
{
if(argc < 2)
{
printf("./a.out dir\n");
exit(1);
}
int total = xxx_getFileNumber(argv[1]);
printf("%s has file number is %d\n", argv[1], total);
return 0;
}