#include "stdafx.h" #include <stack> void bottom(std::stack<int>& s, int t) { if(s.empty()) s.push(t); else { int temp = s.top(); s.pop(); bottom(s,t); s.push(temp); } } void reverse(std::stack<int>& s) { if(s.empty()) return; else { int t = s.top(); s.pop(); reverse(s); bottom(s,t); } } int _tmain(int argc, _TCHAR* argv[]) { std::stack<int> s; s.push(1); s.push(2); s.push(3); reverse(s); while(!s.empty()) { printf("%d ", s.top()); s.pop(); } return 0; }
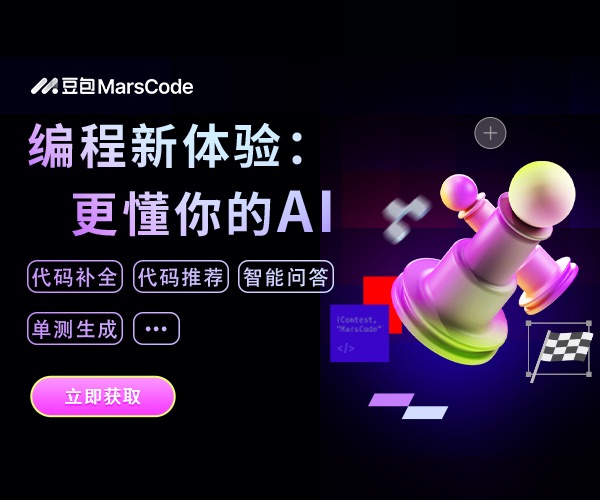
