#include "stdafx.h" #include <cstring> int LCS(const char * str1, const char * str2) { if(str1 == NULL || str2 == NULL) return -1; int len1 = strlen(str1); int len2 = strlen(str2); if(len1 <=0 || len2 <=0) return -1; int ** LCS = new int *[len1]; for(int i=0;i<len1;i++) LCS[i] = new int[len2]; for(int i=0;i<len1;i++) for(int j=0;j<len2;j++) LCS[i][j] = 0; for(int i=0;i<len1;i++) { for(int j=0;j<len2;j++) { if(i==0 || j==0) { if(str1[i] == str2[j]) LCS[i][j] = 1; else LCS[i][j] = 0; } else if(str1[i] == str2[j]) LCS[i][j] = LCS[i-1][j-1] + 1; else if(LCS[i-1][j] > LCS[i][j-1]) LCS[i][j] = LCS[i-1][j]; else LCS[i][j] = LCS[i][j-1]; } } int len = LCS[len1-1][len2-1]; for(int i=0;i<len1;i++) delete [] LCS[i]; delete[] LCS; return len; } int _tmain(int argc, _TCHAR* argv[]) { printf("%d\n", LCS("hello","3el2l")); return 0; }
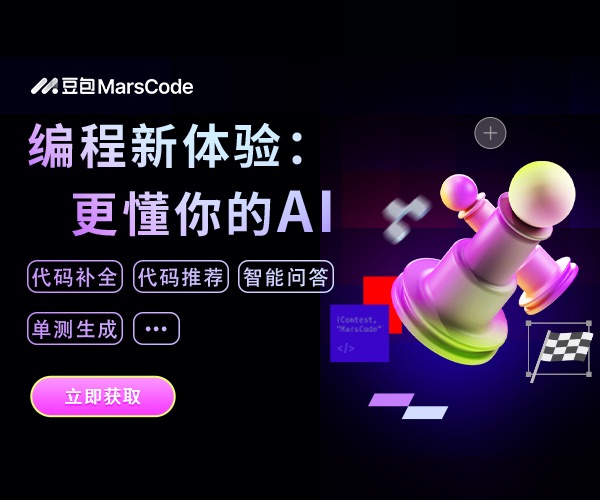
