// 100_12.cpp : Defines the entry point for the console application. // #include "stdafx.h" #include <queue> struct Node { Node * left; Node * right; int value; }; void visit(Node * root) { if(!root) return; std::queue<Node*> q; q.push(root); while(!q.empty()) { Node * temp = q.front(); q.pop(); printf("%d ",temp->value); if(temp->left) q.push(temp->left); if(temp->right) q.push(temp->right); } } int _tmain(int argc, _TCHAR* argv[]) { Node * n1 = new Node(); Node * n2 = new Node(); Node * n3 = new Node(); n1->left = n2; n1->right = n3; n1->value = 1; n2->left = NULL; n2->right = NULL; n2->value = 2; n3->left = NULL; n3->right = NULL; n3->value = 3; visit(n1); return 0; }
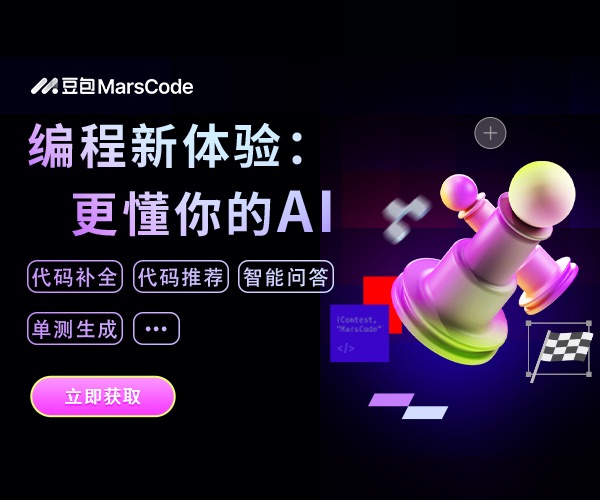
