#include "stdafx.h" #include <vector> struct Node { Node * left; Node * right; int value; }; void findPath(Node * root, std::vector<int> path, int sum, int cur_sum) { if(root==NULL) return; cur_sum += root->value; path.push_back(root->value); if(cur_sum == sum && root->left==NULL && root->right == NULL) { std::vector<int>::iterator iter = path.begin(); for(;iter!=path.end();iter++) printf("%d ",*iter); printf("\n"); } if(root->left) findPath(root->left,path,sum,cur_sum); if(root->right) findPath(root->right,path,sum,cur_sum); } int _tmain(int argc, _TCHAR* argv[]) { Node * n1 = new Node(); Node * n2 = new Node(); Node * n3 = new Node(); Node * n4 = new Node(); Node * n5 = new Node(); n1->left = n2; n1->right = n3; n1->value = 10; n2->left = n4; n2->right = n5; n2->value = 5; n3->left = NULL; n3->right = NULL; n3->value = 12; n4->left = NULL; n4->right = NULL; n4->value = 4; n5->left = NULL; n5->right = NULL; n5->value = 7; std::vector<int> path; findPath(n1, path, 22, 0); return 0; }
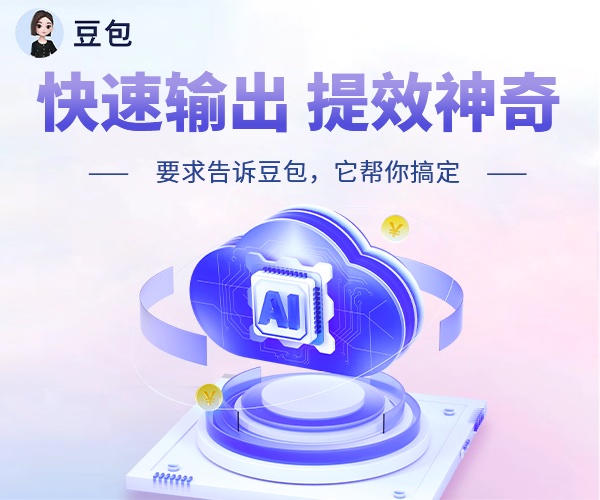
