// 3_10.cpp : 定义控制台应用程序的入口点。 // #include "stdafx.h" #include <vector> struct Node { int data; Node * left; Node * right; }; int printByLevel(Node * root, int level) { if(!root || level < 0) return 0; if(level == 0) { printf("%d ",root->data); return 1; } return printByLevel(root->left, level-1) + printByLevel(root->right, level-1); } //不使用queue的原因是不能区分每一层的结束,没法分行输出 void print(Node * root) { std::vector<Node*> vec; vec.push_back(root); int cur = 0; int last = 1; while(cur<vec.size()) { last = vec.size(); while(cur < last) { printf("%d ", vec[cur]->data); if(vec[cur]->left != NULL) vec.push_back(vec[cur]->left); if(vec[cur]->right != NULL) vec.push_back(vec[cur]->right); cur++; } printf("\n"); } } int _tmain(int argc, _TCHAR* argv[]) { Node * root = new Node(); Node * n1 = new Node(); Node * n2 = new Node(); Node * n3 = new Node(); root->data = 0; root->left = n1; root->right = n2; n1->left = NULL; n1->right = NULL; n2->left = n3; n2->right = NULL; n3->left = NULL; n3->right = NULL; n1->data = 1; n2->data = 2; n3->data = 3; printByLevel(root,1); printf("\n"); print(root); return 0; }
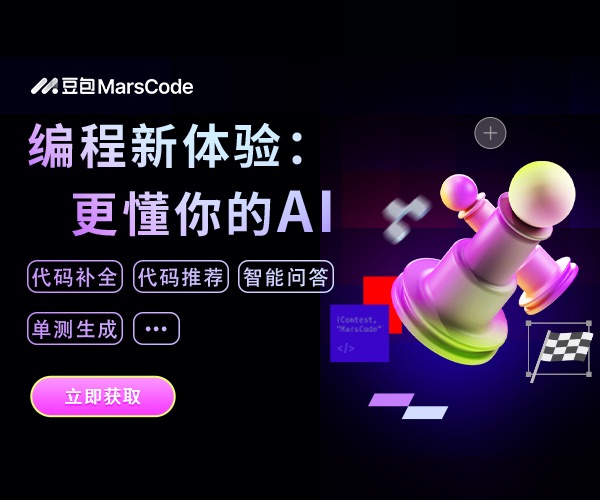
