#include "stdafx.h" struct Node { int value; Node * next; }; //判断是否有环,返回bool,如果有环,返回环里的节点 bool isCircle(Node * head, Node *& circleNode, Node *& lastNode) { Node * fast = head->next; Node * slow = head; while(fast != slow && fast && slow) { if(fast->next != NULL) fast = fast->next; if(fast->next == NULL) lastNode = fast; if(slow->next == NULL) lastNode = slow; fast = fast->next; slow = slow->next; } if(fast == slow && fast && slow) { circleNode = fast; return true; } else return false; } bool detect(Node * head1, Node * head2) { Node * circleNode1; Node * circleNode2; Node * lastNode1; Node * lastNode2; bool isCircle1 = isCircle(head1,circleNode1, lastNode1); bool isCircle2 = isCircle(head2,circleNode2, lastNode2); //一个有环,一个无环 if(isCircle1 != isCircle2) return false; //两个都无环,判断最后一个节点是否相等 else if(!isCircle1 && !isCircle2) { return lastNode1 == lastNode2; } //两个都有环,判断环里的节点是否能到达另一个链表环里的节点 else { Node * temp = circleNode1; while(temp != circleNode1) { if(temp == circleNode2) return true; temp = temp->next; } return false; } return false; } int _tmain(int argc, _TCHAR* argv[]) { Node * n1 = new Node(); Node * n2 = new Node(); Node * n3 = new Node(); Node * n4 = new Node(); n1->next = n2; n2->next = n3; n3->next = n4; n4->next = NULL; Node * n5 = new Node(); Node * n6 = new Node(); Node * n7 = new Node(); Node * n8 = new Node(); n5->next = n6; n6->next = n7; n7->next = n8; n8->next = n5; if(detect(n1,n2)) printf("相交\n"); else printf("不相交\n"); return 0; }
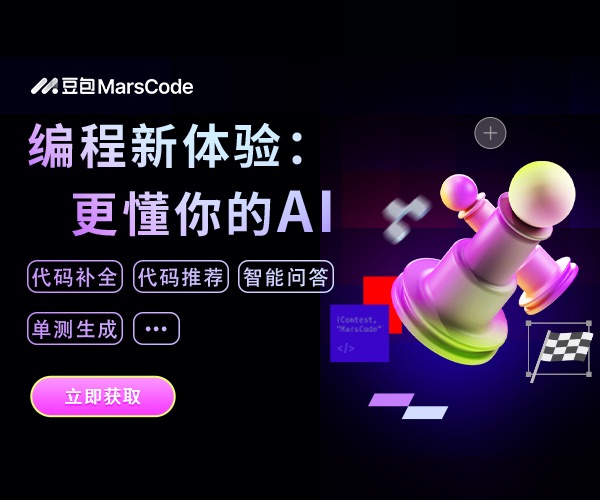
