C++ 重载
重载: C ++允许为同一范围内的函数名称或运算符指定多个定义,分别称为函数重载和运算符重载。
重载声明是在同一范围内与先前声明的声明同名的声明,只是两个声明具有不同的参数和明显不同的定义(实现)。
当调用重载函数或运算符时,编译器通过将您用于调用函数或运算符的参数类型与定义中指定的参数类型进行比较,来确定要使用的最合适的定义。
选择最合适的重载函数或运算符的过程称为重载决议。
C++中的函数重载 n在同一个作用域中,可以为同一个函数名具有多个定义。
函数的定义必须因参数列表中的参数类型和/或参数数量而异。不能重载仅返回类型不同的函数
使用相同函数print()打印不同数据类型的示例:
#include using namespace std; class printData { public: void print(int i) { cout <<< span=""> "Printing int: " <<< span=""> i <<< span=""> endl; } void print(double f) { cout <<< span=""> "Printing float: " <<< span=""> f <<< span=""> endl; } void print(char* c) { cout <<< span=""> "Printing character: " <<< span=""> c <<< span=""> endl; } }; int main(void) { printData pd; // Call print to print integer pd.print(5); // Call print to print float pd.print(500.263); // Call print to print character pd.print("Hello C++"); return 0; }
结果如下:
Printing int: 5 Printing float: 500.263 Printing character: Hello C++
运算符的重载
可以重新定义或重载 C++ 中可用的大多数内置运算符。因此,程序员也可以使用具有用户定义类型的运算符。
重载运算符是具有特殊名称的函数:关键字“运算符”后跟所定义运算符的符号。与任何其他函数一样,重载运算符具有返回类型和参数列表。
Box operator+(const Box&);
声明可用于添加两个 Box 对象并返回最终 Box 对象的加法运算符。大多数重载运算符可以定义为普通的非成员函数或类成员函数。如果我们将上述函数定义为类的非成员函数,那么我们必须为每个操作数传递两个参数,如下所示
Box operator+(const Box&, const Box&);
以下是使用成员函数展示运算符重载概念的示例。这里一个对象作为参数传递,其属性将使用此对象访问,将调用此运算符的对象可以使用此运算符访问
#include using namespace std; class Box { public: double getVolume(void) { return length * breadth * height; } void setLength( double len ) { length = len; } void setBreadth( double bre ) { breadth = bre; } void setHeight( double hei ) { height = hei; } // Overload + operator to add two Box objects. Box operator+(const Box& b) { Box box; box.length = this->length + b.length; box.breadth = this->breadth + b.breadth; box.height = this->height + b.height; return box; } private: double length; // Length of a box double breadth; // Breadth of a box double height; // Height of a box }; // Main function for the program int main() { Box Box1; // Declare Box1 of type Box Box Box2; // Declare Box2 of type Box Box Box3; // Declare Box3 of type Box double volume = 0.0; // Store the volume of a box here // box 1 specification Box1.setLength(6.0); Box1.setBreadth(7.0); Box1.setHeight(5.0); // box 2 specification Box2.setLength(12.0); Box2.setBreadth(13.0); Box2.setHeight(10.0); // volume of box 1 volume = Box1.getVolume(); cout <<"Volume of Box1 : " <<volume <<endl; // volume of box 2 volume = Box2.getVolume(); cout << "Volume of Box2 : " << volume <<endl; // Add two object as follows: Box3 = Box1 + Box2; // volume of box 3 volume = Box3.getVolume(); cout << "Volume of Box3 : " << volume <<< span="">endl; return 0; }
产生的结果
Volume of Box1 : 210 Volume of Box2 : 1560 Volume of Box3 : 5400
可重载/不可重载运算符
可重载运算符
+ | - | * | / | % | ^ | ||
& | | | ~ | ! | , | = | ||
<< span=""> | > | << span="">= | >= | ++ | -- | ||
<< span=""><< span=""> | >> | == | == | && | || | ||
+= | -= | /= |
|
|
&= | ||
|= | *= | << span=""><=< span=""> | >>= | [] | () | ||
-> | ->* |
|
new[] | delete | delete[] |
不可重载运算符
|
|
. | ?: |
class derived-class: access baseA, access baseB....
其中,access为public、protected、private之一,用逗号隔开
#include using namespace std; // Base class Shape class Shape { public: void setWidth(int w) { width = w; } void setHeight(int h) { height = h; } protected: int width; int height; }; // Base class PaintCost class PaintCost { public: int getCost(int area) { return area * 70; } }; // Derived class class Rectangle: public Shape, public PaintCost { public: int getArea() { return (width * height); } }; int main(void) { Rectangle Rect; int area; Rect.setWidth(5); Rect.setHeight(7); area = Rect.getArea(); // Print the area of the object. cout << "Total area: " << Rect.getArea() << endl; // Print the total cost of painting cout <<"Total paint cost: $" <<Rect.getCost(area) <<endl; return 0; }
结果
Total area: 35 Total paint cost: $2450
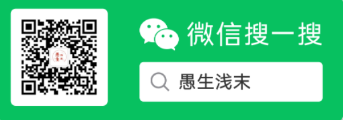