A + B Problem II
Problem Description
I have a very simple problem for you. Given two integers A and B, your job is to calculate the Sum of A + B.
Input
The first line of the input contains an integer T(1<=T<=20) which means the number of test cases. Then T lines follow, each line consists of two positive integers, A and B. Notice that the integers are very large, that means you should not process them by using 32-bit integer. You may assume the length of each integer will not exceed 1000.
Output
For each test case, you should output two lines. The first line is "Case #:", # means the number of the test case. The second line is the an equation "A + B = Sum", Sum means the result of A + B. Note there are some spaces int the equation. Output a blank line between two test cases.
Sample Input
2 1 2 112233445566778899 998877665544332211
Sample Output
Case 1: 1 + 2 = 3 Case 2: 112233445566778899 + 998877665544332211 = 1111111111111111110
代码 如下,为了 省事 ,我用C#写的控制台 程序,并且考虑有点 不太周全 ,只考虑到了A数字长度 大于等于B数字的情况。
using System; using System.Text; namespace TestSum { class Program { static void Main(string[] args) { long a = 112233445566778899; long b = 998877665544332211; //long a = 123; //long b = 29; char[] ac = a.ToString().ToCharArray(); char[] bc = b.ToString().ToCharArray(); StringBuilder result = new StringBuilder(); if(ac.Length>=bc.Length) { int temp = 0; for (int i = ac.Length - 1, j = bc.Length-1; i>=0; i--,j--) { int indexValue = 0; if (temp == 1) { indexValue++; } int _a = 0, _b = 0; if(j<0) { _b = 0; _a = int.Parse(ac[i].ToString()); } else { _a = int.Parse(ac[i].ToString()); _b = int.Parse(bc[j].ToString()); } if(_a+_b>9) { indexValue+= ((_a + _b-10 )); temp = 1; } else { indexValue+= (_a + _b); temp = 0; } result.Append(indexValue); } } reverse(result.ToString()); Console.ReadKey(); } static void reverse(string result) { for(int i =result.Length-1;i>=0;i--) { Console.Write(result[i]); } } } }
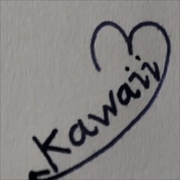
作者:
KMSFan
出处:http://www.cnblogs.com/kmsfan
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。
欢迎大家加入KMSFan之家,以及访问我的优酷空间!
出处:http://www.cnblogs.com/kmsfan
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。
欢迎大家加入KMSFan之家,以及访问我的优酷空间!