
#import "ViewController.h"
@interface ViewController ()<UITextViewDelegate>
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
UIView *bgview = [[UIView alloc] initWithFrame:CGRectMake(20, 200, self.view.frame.size.width-40,60)];
bgview.backgroundColor = [UIColor lightGrayColor];
[self.view addSubview:bgview];
UITextView *textview = [[UITextView alloc] initWithFrame:CGRectMake(50, 10, bgview.frame.size.width-100, 40) textContainer:nil];
textview.backgroundColor = [UIColor whiteColor];
textview.font = [UIFont systemFontOfSize:20];
textview.delegate = self;
textview.text = @"我的网址:http://www.baidu.com";
[bgview addSubview:textview];
//UILabel UITextField UItextView
//多样化显示文本:下划线 字体大小颜色粗细 URL链接
//attributedText
//1.将原来的普通文本转化为属性文本 NSAttributedString
NSMutableAttributedString*attrstr= [[NSMutableAttributedString alloc] initWithString:textview.text] ;
//2.设置自己的样式 我的网址:http://www.baidu.com
/*(1)下划线 NSUnderlineStyleAttributeName
下划线颜色
NSUnderlineColorAttributeName
(2)删除线
NSStrikeyhroughStyleAttributeName
删除线颜色NSStrikethroughColorAttributeName
(3)字体
NSFontAttributeName
(4)
NSStrokeWidthAttributeName ->设置笔画宽度(粗细),取值为 NSNumber 对象(整数),负值填充效果,正值中空效果 NSStrokeColorAttributeName ->填充部分颜色,不是字体颜色,取值为 UIColor 对象
(5)url链接
NSLinkAttributeName
*/
[attrstr setAttributes:@{NSUnderlineStyleAttributeName:@1,NSUnderlineColorAttributeName:[UIColor redColor],NSStrikethroughStyleAttributeName:@1,NSStrikethroughColorAttributeName:[UIColor blackColor],NSFontAttributeName:[UIFont systemFontOfSize:25],NSLinkAttributeName:@"http://www.baidu.com"} range:NSMakeRange(5, 20)];
//将多样化文本赋给textview
textview.attributedText = attrstr;
//创建图片附件NSTextAttachment 文本附件 实现图文混排
NSTextAttachment *attach=[[NSTextAttachment alloc] init];
attach.image = [UIImage imageNamed:@"face"];
attach.bounds = CGRectMake(0, 0, 20, 20);
//将这个图片转化为属性字符串
NSAttributedString *imageAttr = [NSAttributedString attributedStringWithAttachment:attach];
//属性字符串的拼接
[attrstr appendAttributedString:imageAttr];
//赋值属性字符串
textview.attributedText=attrstr;
}

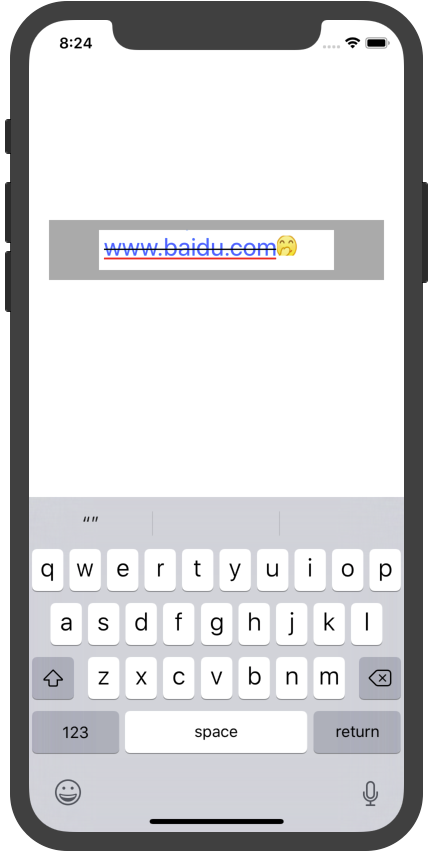