Python基础 - 10模块和包
一、模块
1.1 自定义模块: 新建 calculate10.py 文件, 包括 函数add、类Calculate、变量number
把功能相近的函数放到一个文件中,一个Python文件就是一个模块,模块名就是文件名去掉后缀py。
提高代码的可复用、可维护性。 解决了命名冲突。
number = 10
name = 'calculater'
def add(*args):
sum = 0
if len(args)> 1:
for i in args:
sum += i
return sum
else:
print('至少传入两个参数')
return 0
class Calculate:
def __init__(self, num):
self.num = num
def test(self):
print('正在使用calculate10进行计算...')
@classmethod
def test1(self):
print('--> calculate10 中 类方法')
1.2 引用模块,使用模块的函数、类、变量
导入模块:
import 模块名 模块名.变量 模块名.函数 模块名.类
from 模块名 import 变量 | 函数 | 类 代码中直接使用变量、函数、类
from 模块名 import *
import calculate10 # 导入模块
list1 = [3,4,5,6]
result = calculate10.add(*list1)
print(result) # 18
print(calculate10.number) # 10
cal = calculate10.Calculate(88)
cal.test() # 正在使用calculate10进行计算...
calculate10.Calculate.test1() # --> calculate10 中 类方法
from calculate10 import add, number, Calculate
list2 = [12,98,22]
result2 = add(*list2)
print(result2) # 132
sum = result2 +number
print(sum) # 142
c = Calculate(89)
c.test() # 正在使用calculate10进行计算...
Calculate.test1() # --> calculate10 中 类方法
# calculate10.py 新增函数, 并调用
def test():
print('我是测试......')
test()
from calculate10 import *
list3 = [12,98,22,76]
result3 = add(*list3)
print(result3) # 我是测试...... \n 208
print(result3) # 208
print(number) # 10
c3 = Calculate(1)
c3.test() # 正在使用calculate10进行计算...
Calculate.test1() # --> calculate10 中 类方法
1.3 在 calculate10.py 定义 __all__ 指定可引用的内容
__all__ = ['add', 'number', 'Calculate'] # __all__ 指定可以被其他的文件引用的模块 内容
# 执行 calculate10.py, 引用calculate10 不执行以下方法
if __name__ == '__main__': print(__name__) test() ''' __main__ 我是测试...... '''
二、包
2.1 创建包 ,包含一个__init__.py

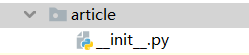
2.2 案例
一个包中可以包含多个模块。 项目 > 包 > 模块 > 类 函数 变量
'''
目录结构:
article
|--models.py
|--__init__.py
|--..
user
|--models.py
|--__init__.py
|--
package.py
from 包 import 模块
from 包.模块 import 类 | 函数 | 变量
'''
# article/models.py
class Article: def __init__(self, name, author): self.name = name self.author = author def show(self): print('发表文章名字:{},作者是{}'.format(self.name, self.author)) class Tag: def __init__(self, name): self.name = name
# user/models.py
class User:
def __init__(self, username, password):
self.username = username
self.password = password
def login(self, username, password):
if username == self.username and password == self.password:
print('登录成功')
else:
print('登录失败')
def publish_article(self, article):
print(self.username, '发表了文章:', article.name)
def show(self):
print('当前用户为:{}, 密码为{}'.format(self.username, self.password))
# package.py 使用包 模块中的User类
from user import models
u = models.User('admin','1234')
u.show() # 当前用户为:admin, 密码为1234
from user.models import User # from .models import User 当在当前目录下的models里面的User类
u = User('admin','1234')
u.show() # 当前用户为:admin, 密码为1234
from article.models import Article
a = Article('期末总结','王花花')
a.show() # 发表文章名字:期末总结,作者是王花花
u.publish_article(a) # admin 发表了文章: 期末总结
2.3 __init__.py文件、导入模块和包
__init__.py文件: 当导入包的时候,默认调用__init__.py文件。
1. 当导入包时,把一些初始化的函数、变量、类定义在__init__.py文件中;
2. 此文件中的函数、变量的访问,只能通过 包名.函数 ...
3. 结合__all__定义可访问的模块
from 模块 import *
表示可以使用模块里面的所有内容,如果没有定义__all__所有的都可以访问,
如果添加了__all__,只能访问__all__列表中的内容
from 包 import *
表示该包中内容(模块)不能访问,需要在__init__.py文件定义__all__
__all__=['*'] 访问全部内容
#user / __init__.py
__all__ = ['models','test']
print('----> user包的__init__')
def create_app():
print('----> create_app')
def printA():
print('**** printA')
from user.models import User
import user
user.create_app()
user.printA()
'''
----> user包的__init__ # 只要import user 就输出
----> create_app
**** printA
'''
from user import *
u1 = models.User('aa','11')
u1.show()
'''
----> user包的__init__ # user 包中的 __init__ 文件
----user---- # models 中的 print 语句
当前用户为:aa, 密码为11
'''
2.4 循环导入
避免产生循环导入的方法
1. 重新架构
2. 将导入的语句放在函数里面
3. 将导入的语句放在模块的最后
# cycle02.py
def func():
print('循环导入2里面的func----1----')
from cycle01 import task1
task1()
print('循环导入2里面的func----2----')
def task2():
print('循环导入2里面的----task2----')
func()
task2()
# cycle01.py
def func():
print('循环导入1里面的func----1----')
from cycle02 import task2
print('循环导入1里面的func----2----')
task2()
def task1():
print('循环导入1里面的----task1-----')
func()
def task2():
print('循环导入1里面的----task2----')
func()
if __name__ == '__main__':
task1()
task2()
# RecursionError: maximum recursion depth exceeded while calling a Python object
'''
循环导入1里面的----task1----- 1. task1 开始
循环导入1里面的func----1---- 2. task1 的 func
循环导入2里面的----task2---- 3. 执行 2里面的 task2
循环导入2里面的func----1---- 4. 执行 2里面的 task2 执行 func
循环导入1里面的----task1----- 5. 执行 2里面的 task2 导入 1里面的 task1
循环导入1里面的func----1---- 6. 执行 2里面的 task2 导入 1里面的 task1 执行 func
循环导入1里面的func----2---- 7. 执行 2里面的 task2 导入 1里面的 task1 执行 func 结束, 没有再次导入2
循环导入2里面的----task2---- 8. 导入 2里面的 task2
循环导入2里面的func----1---- 9. 导入 2里面的 task2 执行 func
循环导入1里面的----task1----- 10. 导入 2里面的 task2 执行 func 执行 1里面的 task1, 跳过导入1
循环导入1里面的func----1---- 11. 导入 2里面的 task2 执行 func 执行 1里面的 task1 执行func
循环导入1里面的func----2---- 12. 导入 2里面的 task2 执行 func 执行 1里面的 task1 执行func
循环导入2里面的----task2---- 13. 导入 2里面的 task2 执行 func 执行 1里面的 task1 执行func 的 2里面的 task2
循环导入2里面的func----1---- 14. 执行 2里面的 task2, 回到第9步
循环导入1里面的----task1----- 15. 10
循环导入1里面的func----1---- 16. 11
循环导入1里面的func----2---- 19. 12
循环导入2里面的----task2---- 20. 13
循环导入2里面的func----1---- 21. 9
循环导入1里面的----task1----- 22. 10
循环导入1里面的func----1---- 23. 11
循环导入1里面的func----2---- 24. 12
循环导入2里面的----task2---- 25. 13
'''
三、常见模块
当导入一个模块时,Python解析器对模块位置的搜索顺序:
1. 当前目录
2. 如果不在当前目录, Python则搜索在 shell 变量 PYTHONPATH 下的每个目录。
3. 如果都找不到, Python 查看默认路径。Linux: /usr/local/lib/python/>。
模块搜索路径存储在 system 模块的 sys.path 变量中。变量包括当前目录、PYTHONPATH和安装过程中决定的默认目录。
['E:\\PythonLearn\\pythonBase\\02door2', 'E:\\PythonLearn\\pythonBase',
'D:\\Program Files\\JetBrains\\PyCharm 2020.3.4\\plugins\\python\\helpers\\pycharm_display',
'D:\\Program Files\\Python39\\python39.zip', 'D:\\Program Files\\Python39\\DLLs',
'D:\\Program Files\\Python39\\lib', 'D:\\Program Files\\Python39', 'E:\\PythonLearn\\pythonBase\\venv',
'E:\\PythonLearn\\pythonBase\\venv\\lib\\site-packages',
'D:\\Program Files\\JetBrains\\PyCharm 2020.3.4\\plugins\\python\\helpers\\pycharm_matplotlib_backend']
常见标准库:
builtins(内建函数默认加载) math(数学库)
random(生成随机数) time(时间) datetime(日期和时间) calendar(日历) re(字符串正则匹配)
hashlib(加密算法) copy(拷贝) functools(常用工具) os(操作系统接口) sys(Python自身的运行环境)
multiprocessing(多进程) threading(多线程) json(编码和解码JSON对象) logging(记录日志,调试)
3.1 sys模块
import sys
print(sys.path)
print(sys.version) # 3.9.2 (tags/v3.9.2:1a79785, Feb 19 2021, 13:44:55) [MSC v.1928 64 bit (AMD64)]
print(sys.argv) # ['E:/PythonLearn/pythonBase/02door2/10-module-02.py']
3.2 time模块
# 时间戳
import time
t = time.time()
print(t) # 1633066930.5659792
for i in range(10000):
pass
t1 = time.time()
print(t1) # 1633075771.3982167
# 将时间戳转成字符串
s = time.ctime(t1)
print(s) # Fri Oct 1 16:06:59 2021
# 将时间戳转成元祖
ss = time.localtime(t1)
print(ss) # time.struct_time(tm_year=2021, tm_mon=10, tm_mday=1, tm_hour=16,
# tm_min=7, tm_sec=38, tm_wday=4, tm_yday=274, tm_isdst=0)
print(ss.tm_yday) # 274
print(ss.tm_hour) # 16
# 将元祖转成时间戳
tt = time.mktime(ss)
print(tt) # 1633075771.0
# 将元祖的时间转成字符串
s2 = time.strftime('%Y-%m-%d %H:%M:%S')
print(s2) # 2021-10-01 16:11:15
# 将字符串转成元祖形式
r = time.strptime('2019/06/20','%Y/%m/%d')
print(r) # time.struct_time(tm_year=2019, tm_mon=6, tm_mday=20, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=3, tm_yday=171, tm_isdst=-1)
3.3 datetime模块
time: 时间 date: 日期 datetime: 日期时间 timedelta:时间差
import datetime, time
print(datetime.time.hour) # <attribute 'hour' of 'datetime.time' objects>
d = datetime.date(2021,10,1)
print(datetime.date.day) # <attribute 'day' of 'datetime.date' objects>
print(time.time()) # 1633076111.1546452
print(datetime.date.ctime(d)) # Fri Oct 1 00:00:00 2021
print(datetime.date.today()) # 2021-10-01
timedel = datetime.timedelta(hours=2)
print(timedel) # 2:00:00
now = datetime.datetime.now()
print(now) # 2021-10-01 16:38:57.440715
result = now - timedel
print(result) # 2021-10-01 14:38:57.440715
result = now + timedel
print(result) # 2021-10-01 18:39:33.240022
timedel = datetime.timedelta(days=7, hours=12)
print(timedel) # 7 days, 12:00:00
3.4 random模块
import random
ran = random.random()
print(ran) # 0.599162803272479
ran = random.randrange(1,10,2)
print(ran) # 3
ran = random.randint(1,10) # [1,10]
print(ran)
list = ['张三', '飞飞', '菲菲', '悟空','八戒']
ran = random.choice(list)
print(ran) # 飞飞
list1 = [1,2,3,4,5,6,7,8,9,10]
result = random.shuffle(list1)
print(result) # None
print(list1) # [5, 4, 3, 1, 2, 9, 6, 10, 8, 7]
3.5 hashlib模块
import hashlib
msg = '今天喝酒。'
str = hashlib.md5('aaa'.encode('utf-8'))
print(str) # md5 _hashlib.HASH object @ 0x000000A9058B0EF0>
print(str.hexdigest()) # 47bce5c74f589f4867dbd57e9ca9f808
print(len(str.hexdigest())) # 32
str_sha1 = hashlib.sha1(msg.encode('utf-8'))
print(str_sha1.hexdigest()) # 60d1482eb9e334c54fe6ba56d8c4a1be7644bbc3
str_sha2 = hashlib.sha1(msg.encode('utf-8'))
print(str_sha2.hexdigest()) # 60d1482eb9e334c54fe6ba56d8c4a1be7644bbc3
print(len(str_sha2.hexdigest())) # 40
str_sha256 = hashlib.sha256(msg.encode('utf-8'))
print(len(str_sha256.hexdigest())) # 64
3.6 第三方模块
安装第三方模块: pip install pillow
import requests
response = requests.get('https://www.baidu.com')
print(response) # <Response [200]>
print(response.text)
print(response.content)