netty版本
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.39.Final</version>
</dependency>
服务端代码
点击查看代码
package com.shenle.qianhe.netty.c1;
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.string.StringDecoder;
/**
* @author : gaoqiqiang
* @date : 2023/3/29 0029 22:33
* @modyified By :
*/
public class HelloServer {
public static void main(String[] args) {
// 1 服务端的启动器,负责组装netty组件,启动服务器
new ServerBootstrap()
//2 添加了 BossEventLoop,WorkerEventLoop(selector,thread) accept read
//16 由某个EventLoop处理read事件,接收到ByteBuf
.group(new NioEventLoopGroup())
//3 选择服务器的 ServerSocketChannel 实现
.channel(NioServerSocketChannel.class)
//4 boss负责处理连接,worker(child)负责处理读写,决定了 worker(child)能执行哪些操作(handler)
.childHandler(
//5 Channel代表和客户端进行数据读写的通道 Initializer 初始化,负责添加别的 handler
new ChannelInitializer<NioSocketChannel>() {
// 12 连接建立后,调用初始化方法
@Override
protected void initChannel(NioSocketChannel ch) throws Exception {
// 添加具体 handler StringDecoder 17 将ByteBuf 转为字符串
ch.pipeline().addLast(new StringDecoder());
// ChannelInboundHandlerAdapter 自定义 handler
ch.pipeline().addLast(new ChannelInboundHandlerAdapter() {
// 读事件
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
// 打印上一步转化好的字符串 18 执行read方法 打印由17步传过来的字符串
System.out.println("shenle receive:" + msg);
}
});
}
})
//6 绑定的监听端口
.bind(8080);
}
}
客户端代码
点击查看代码
package com.shenle.qianhe.netty.c1;
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.string.StringEncoder;
import java.net.InetSocketAddress;
/**
* @author : gaoqiqiang
* @date : 2023/3/29 0029 22:54
* @modyified By :
*/
public class HelloClient {
public static void main(String[] args) throws InterruptedException {
//7 启动器
new Bootstrap()
//8
.group(new NioEventLoopGroup())
//9 选择客户端channel实现
.channel(NioSocketChannel.class)
//10 添加处理器
.handler(new ChannelInitializer<NioSocketChannel>() {
//12 在连接建立后被调用
@Override
protected void initChannel(NioSocketChannel ch) throws Exception {
//15 将字符串编码成ByteBuf
ch.pipeline().addLast(new StringEncoder());
}
})
// 11 连接到服务器
.connect(new InetSocketAddress("localhost",8080))
//13 阻塞方法 直到连接建立
.sync()
// 代表连接对象
.channel()
//14 向服务器发送数据
.writeAndFlush("hello world");
}
}
运行结果
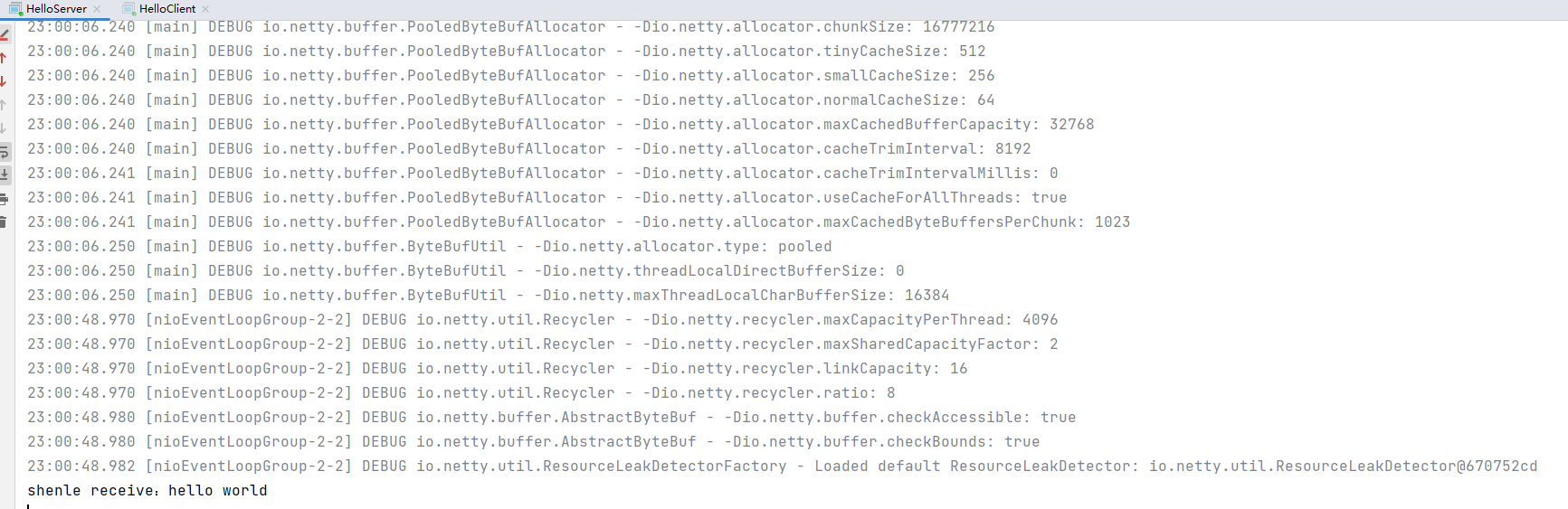
图解 (看编号就是执行步骤)
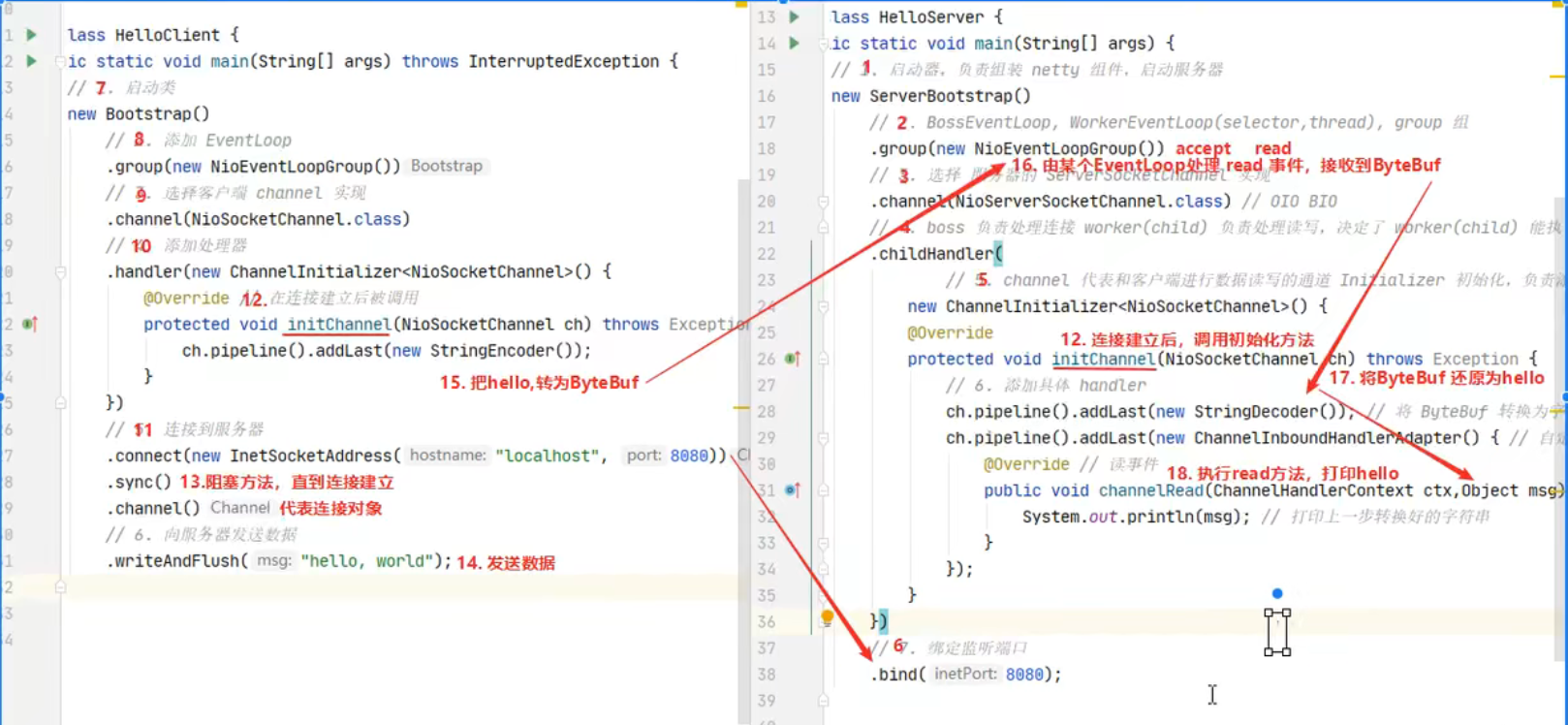
为了便于理解
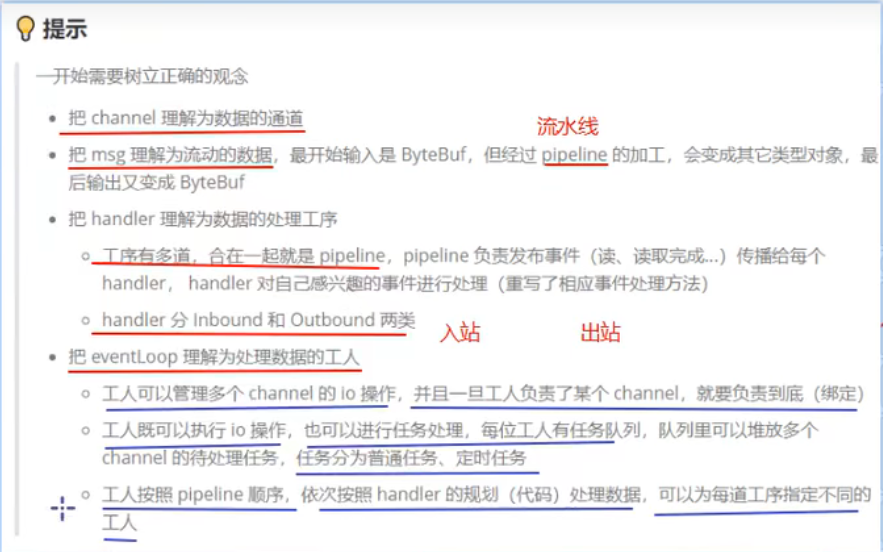