1 using System;
2 using System.Collections.Generic;
3 using System.ComponentModel;
4 using System.Data;
5 using System.Drawing;
6 using System.Linq;
7 using System.Text;
8 using System.Windows.Forms;
9
10 namespace _Random
11 {
12 public partial class Form1 : Form
13 {
14 public Form1()
15 {
16 InitializeComponent();
17 }
18 public static int Count = 0;
19 private int t = 60;
20 public static int right = 0;
21
22 private void button1_Click(object sender, EventArgs e)
23 {
24 label2.Text = t.ToString();
25 timer1.Enabled = true;
26 timer1.Interval = 1000;
27 timer1.Start();
28 }
29
30 private void RDN()
31 {
32 Random rd = new Random();
33 int r1, r2;
34 if (textBox4.Text == "" && textBox5.Text == "")
35 {
36 MessageBox.Show("请输入取值范围!");
37 return;
38 }
39 r1 = rd.Next(int.Parse(textBox4.Text), int.Parse(textBox5.Text));
40 r2 = rd.Next(int.Parse(textBox4.Text), int.Parse(textBox5.Text));
41 textBox1.Text=r1.ToString();
42 textBox2.Text=r2.ToString();
43 string[] fuhao = new string[]{"+","-","×","÷"};
44 label3.Text = fuhao[rd.Next(0,5)];
45 int result = 0;
46 switch (label3.Text)
47 {
48 case "+":
49 result = int.Parse(textBox1.Text) + int.Parse(textBox2.Text);
50 return;
51 case "-":
52 if (int.Parse(textBox1.Text) >= int.Parse(textBox2.Text))
53 {
54 result = int.Parse(textBox1.Text) - int.Parse(textBox2.Text);
55 }
56 else
57 {
58 MessageBox.Show("请回车进行下一题!此题不计入答题总数!");
59 }
60 return;
61 case "×":
62 result = int.Parse(textBox1.Text) * int.Parse(textBox2.Text);
63 return;
64 case "÷":
65 if (textBox2.Text == "0")
66 {
67 MessageBox.Show("分母为0,不计入答题总数,请回车继续答题!");
68 }
69 else
70 {
71 result = int.Parse(textBox1.Text) / int.Parse(textBox2.Text);
72 }
73 return;
74 }
75 }
76
77 private void RandomNum()
78 {
79 Random ran=new Random();
80 int n1,n2;
81 if (textBox4.Text==""&&textBox5.Text=="")
82 {
83 MessageBox.Show("请输入取值范围!");
84 return;
85 }
86 n1=ran.Next(int.Parse(textBox4.Text),int.Parse(textBox5.Text));
87 n2 = ran.Next(int.Parse(textBox4.Text), int.Parse(textBox5.Text));
88 textBox1.Text=n1.ToString();
89 textBox2.Text=n2.ToString();
90 textBox3.Text="";
91 }
92
93 private void timer1_Tick(object sender, EventArgs e)
94 {
95 if (t <= 0)
96 {
97 timer1.Enabled = false;
98 textBox3.Enabled = false;
99 MessageBox.Show("时间到!");
100 textBox3.Enabled = false;
101 Form2 frm2 = new Form2();
102 frm2.ShowDialog();
103 }
104 t = t - 1;
105 label2.Text = t.ToString();
106 }
107
108 private void button2_Click(object sender, EventArgs e)
109 {
110 timer1.Stop();
111 Form2 frm2 = new Form2();
112 frm2.ShowDialog();
113 }
114
115 private void textBox3_KeyDown(object sender, KeyEventArgs e)
116 {
117 int result = 0;
118 string s = label3.Text;
119 if (Count == int.Parse(textBox6.Text))
120 {
121 Form2 frm2 = new Form2();
122 frm2.ShowDialog();
123 }
124 switch (s)
125 {
126 case "+":
127 result = int.Parse(textBox1.Text) + int.Parse(textBox2.Text);
128 break;
129 case "-":
130 if (int.Parse(textBox1.Text) >= int.Parse(textBox2.Text))
131 {
132 result = int.Parse(textBox1.Text) - int.Parse(textBox2.Text);
133 }
134 else
135 {
136 MessageBox.Show("请回车进行下一题!此题不计入答题总数!");
137 }
138 break;
139 case "×":
140 result = int.Parse(textBox1.Text) * int.Parse(textBox2.Text);
141 break;
142 case "÷":
143 if (textBox2.Text=="0")
144 {
145 MessageBox.Show("分母为0,不计入答题总数,请回车继续答题!");
146 }
147 else
148 {
149 result = int.Parse(textBox1.Text) / int.Parse(textBox2.Text);
150 }
151 break;
152 }
153 if (e.KeyCode == Keys.Enter)
154 {
155 if (textBox3.Text == result.ToString())
156 {
157 right++;
158 Count++;
159 MessageBox.Show("回答正确!");
160 }
161 else
162 {
163 if (textBox2.Text=="0"||int.Parse(textBox1.Text)-int.Parse(textBox2.Text)<0)
164 {
165 RandomNum();
166 }
167 else
168 {
169 MessageBox.Show("答题错误!");
170 RandomNum();
171 Count++;
172 }
173 }
174 RandomNum();
175 }
176 }
177
178 private void button3_Click(object sender, EventArgs e)
179 {
180 label3.Text = button3.Text;
181 RandomNum();
182 }
183
184 private void button4_Click(object sender, EventArgs e)
185 {
186 label3.Text = button4.Text;
187 RandomNum();
188 }
189
190 private void button5_Click(object sender, EventArgs e)
191 {
192 label3.Text = button5.Text;
193 RandomNum();
194 }
195
196 private void button6_Click(object sender, EventArgs e)
197 {
198 label3.Text = button6.Text;
199 RandomNum();
200 }
201
202 private void button7_Click(object sender, EventArgs e)
203 {
204 if (textBox4.Text == "" && textBox5.Text == "")
205 {
206 MessageBox.Show("请输入取值范围!");
207 return;
208 }
209 else
210 {
211 for (int i = 0; i < int.Parse(textBox6.Text);i++)
212 {
213 RDN();
214 }
215 }
216 }
217 }
218 }
10 namespace _Random
11 {
12 public partial class Form2 : Form
13 {
14 public Form2()
15 {
16 InitializeComponent();
17 }
18
19 private void Form2_Load(object sender, EventArgs e)
20 {
21 textBox1.Text = Form1.Count.ToString();
22 textBox2.Text = Form1.right.ToString();
23 textBox3.Text = (Form1.Count - Form1.right).ToString();
24 }
25
26 }
27 }
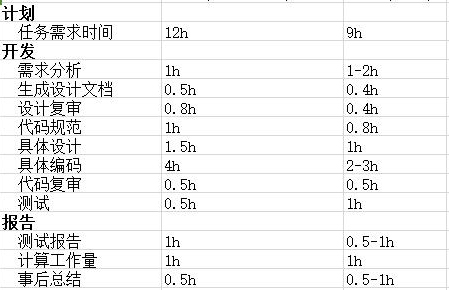